在 C# 中检查文本框是否为空
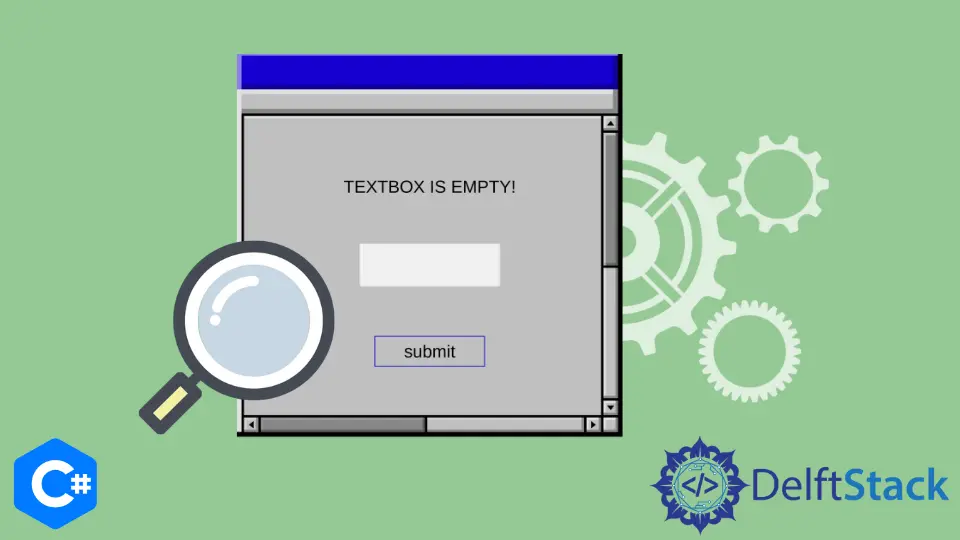
本教程将讨论如何在 C# 中检查文本框是否为空。
使用 C# 中的 String.IsNullOrEmpty()
函数检查文本框是否为空
String.IsNullOrEmpty()
函数检查 C# 中字符串是否为空。String.IsNullOrEmpty()
函数具有布尔返回类型,如果字符串为 null
或为空,则返回 true
,否则返回 false
。我们可以对 TextBox.Text
属性内的字符串使用 String.IsNullOrEmpty()
函数来检查文本框中的文本是否为空。请参见以下代码示例。
using System;
using System.Windows.Forms;
namespace check_if_textbox_is_empty {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
if (String.IsNullOrEmpty(textBox1.Text)) {
label1.Text = "TEXT BOX IS EMPTY";
}
}
}
}
输出:
在上面的代码中,我们使用 C# 中的 String.IsEmptyOrNot()
函数检查了文本框是否为空。我们还可以使用 String.IsNullOrWhitespace()
函数检查文本框内是否有空格,如下所示。
using System;
using System.Windows.Forms;
namespace check_if_textbox_is_empty {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
if (String.IsNullOrWhitespace(textBox1.Text)) {
label1.Text = "TEXT BOX IS EMPTY";
}
}
}
}
如果文本框中仅包含空格,则该方法还将空格考虑在内,并显示错误消息 TEXT BOX IS EMPTY
。
使用 C# 中的 TextBox.Text.Length
属性检查文本框是否为空
在 C# 中,TextBox.Text.Length
属性获取文本框内的文本长度。我们可以在 if
语句中使用 TextBox.Text.Length == 0
条件来检查文本框是否为空。请参见以下代码示例。
using System;
using System.Windows.Forms;
namespace check_if_textbox_is_empty {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
if (textBox1.Text.Length == 0) {
label1.Text = "TEXT BOX IS EMPTY";
}
}
}
}
输出:
在上面的代码中,我们使用 C# 中的 TextBox.Text.Length
属性检查了文本框是否为空。不建议使用此方法,因为它没有考虑空格。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn