在 C# 中的文本框中仅接受数字
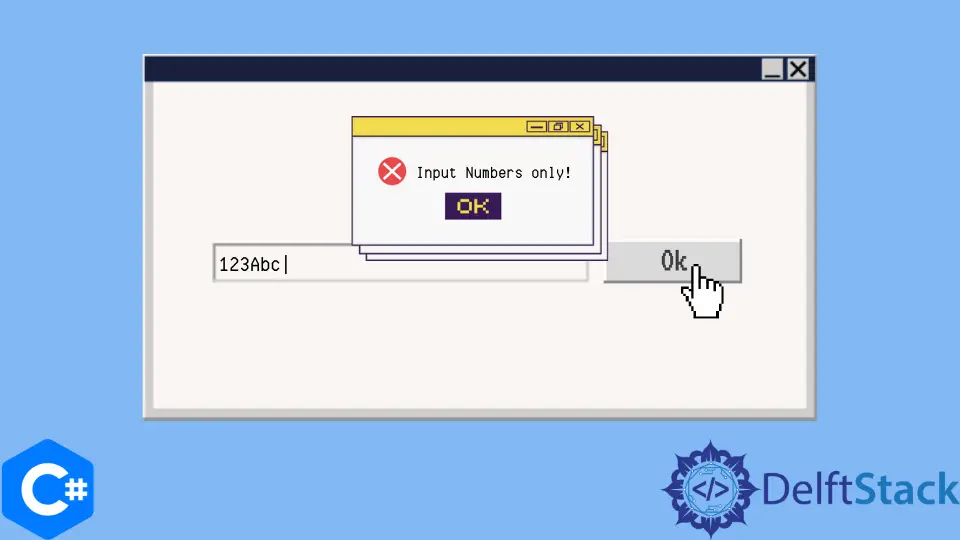
本教程将介绍在 C# 中创建一个只接受数字的文本框的方法。
C# 中带有 NumberUpDown
视图的仅数字文本框
NumberUpDown
视图用于从用户使用 C# 获取数字输入。要在 Windows 窗体应用程序中添加 NumberUpDown
视图,我们只需从工具箱中选择 NumberUpDown
并将其拖到我们的窗体中即可。NumberUpDown
视图不会从用户那里获取任何非数字值。它还允许我们使用光标键上下移动一个值。下面的代码示例向我们展示了如何使用 C# 中的 NumberUpDown
视图创建一个仅接受来自用户的数值的文本框。
using System;
using System.Windows.Forms;
namespace textbox_numbers_only {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void numericUpDown1_KeyPress(object senderObject, KeyPressEventArgs KeyPressEvent) {
if (!char.IsControl(KeyPressEvent.KeyChar) && !char.IsDigit(KeyPressEvent.KeyChar) &&
(KeyPressEvent.KeyChar != '.')) {
KeyPressEvent.Handled = true;
}
if ((KeyPressEvent.KeyChar == '.') && ((senderObject as TextBox).Text.IndexOf('.') > -1)) {
KeyPressEvent.Handled = true;
}
}
private void numericUpDown1_ValueChanged(object sender, EventArgs e) {}
}
}
输出:
在上面的代码中,我们创建了一个文本框,该文本框仅使用 C# 中的 NumberUpDown
视图接受用户的数值。在 numericUpDown1_KeyPress()
函数中,我们添加了对 .
值的检查,以允许用户输入小数点值。此文本框仅接受 2 位数字的数值。
在 C# 中仅带有 TextBox
视图的数字文本框
如果我们不想使用任何专有的视图,我们还可以修改原始的 TextBox
视图以仅接受数字值。我们可以使用 TextBox_KeyPress()
函数内的 KeyPressEventArgs.Handled
属性来指定文本框应处理哪些按键。以下代码示例向我们展示了如何使用 C# 中的 TextBox
视图创建仅接受数值的文本框。
using System;
using System.Windows.Forms;
namespace textbox_numbers_only {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void textBox1_TextChanged(object sender, EventArgs e) {}
private void textBox1_KeyPress(object sender, KeyPressEventArgs KeyPressEvent) {
KeyPressEvent.Handled = !char.IsDigit(KeyPressEvent.KeyChar);
}
}
}
输出:
在上面的代码中,我们指定了我们的文本框不应使用 textBox1_KeyPress()
函数中的 KeyPressEvent.Handled
属性处理任何非数字值。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn