How to Have Numbers Only in TextBox in C#
-
Numbers Only TextBox With the
NumberUpDown
View inC#
-
Numbers Only TextBox With the
TextBox
View inC#
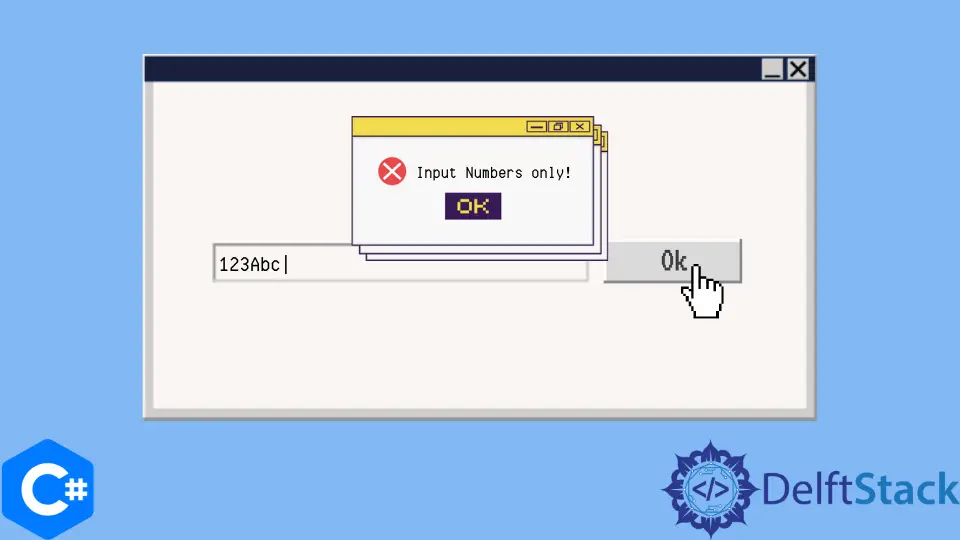
This tutorial will introduce the methods to create a text box that only accepts numbers in C#.
Numbers Only TextBox With the NumberUpDown
View in C#
The NumberUpDown
view is used to get numeric input from the user in C#. To add a NumberUpDown
view in our Windows Form application, we just select NumberUpDown
from the toolbox and drag it to our form. The NumberUpDown
view does not take any non-numeric values from the user. It also allows us to move one value up or down with the cursor keys. The following code example shows us how we can create a text box that only accepts numeric values from the user with the NumberUpDown
view in C#.
using System;
using System.Windows.Forms;
namespace textbox_numbers_only {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void numericUpDown1_KeyPress(object senderObject, KeyPressEventArgs KeyPressEvent) {
if (!char.IsControl(KeyPressEvent.KeyChar) && !char.IsDigit(KeyPressEvent.KeyChar) &&
(KeyPressEvent.KeyChar != '.')) {
KeyPressEvent.Handled = true;
}
if ((KeyPressEvent.KeyChar == '.') && ((senderObject as TextBox).Text.IndexOf('.') > -1)) {
KeyPressEvent.Handled = true;
}
}
private void numericUpDown1_ValueChanged(object sender, EventArgs e) {}
}
}
Output:
In the above code, we create a text box that only accepts numeric values from the user with the NumberUpDown
view in C#. In the numericUpDown1_KeyPress()
function, we have added a check for .
value to allow the user to enter decimal point values. This text box only takes numeric values with 2 digits.
Numbers Only TextBox With the TextBox
View in C#
If we do not want to use any proprietary views, we can also modify the original TextBox
view to accept only numeric values. We can use the KeyPressEventArgs.Handled
property inside the TextBox_KeyPress()
function to specify which key presses should be handled by our text box. The following code example shows us how we can create a text box that only accepts numeric values with the TextBox
view in C#.
using System;
using System.Windows.Forms;
namespace textbox_numbers_only {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void textBox1_TextChanged(object sender, EventArgs e) {}
private void textBox1_KeyPress(object sender, KeyPressEventArgs KeyPressEvent) {
KeyPressEvent.Handled = !char.IsDigit(KeyPressEvent.KeyChar);
}
}
}
Output:
In the above code, we specified that our text box should not handle any non-numeric values with the KeyPressEvent.Handled
property in the textBox1_KeyPress()
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn