C++ Map<T1, T2> Equivalent in C#
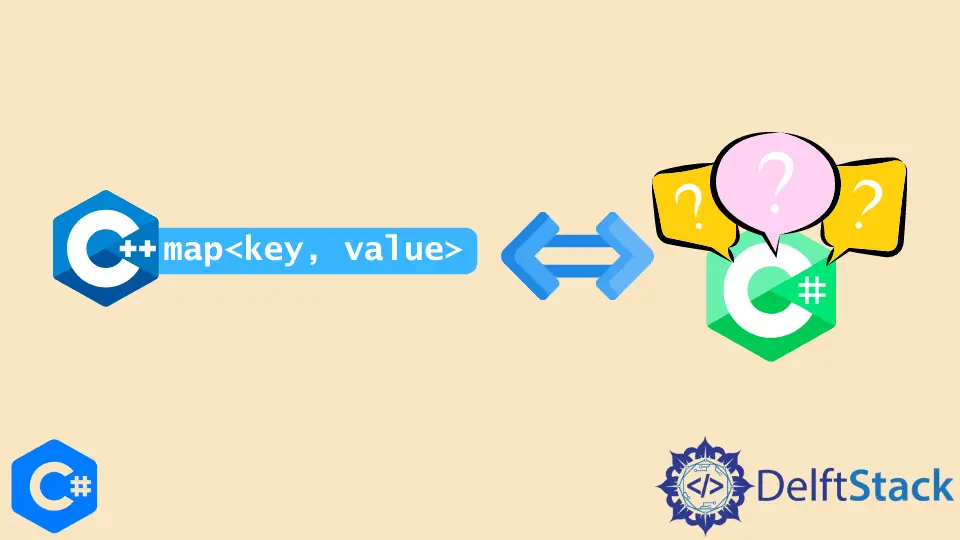
This tutorial will introduce C# equivalent of the map<T1, T2> in C++.
C++ map<key, value>
Equivalent in C#
The map<key, value>
data structure in C++ is used to hold data in the form of key-value
pairs. The closest alternative to this is the Dictionary<Tkey, Tvalue>
class in C#. The Dictionary
data structure also holds data in the form of key-value
pairs in C#. If we are concerned with the order of the entries inside the dictionary, we can use the SortedDictionary<Tkey, Tvalue>
class. The following code example shows us how we can store data in the form of a key-value
pair with the SortedDictionary<Tkey, Tvalue>
class in C#.
using System;
using System.Collections.Generic;
namespace C__map_alternative {
class Program {
static void Main(string[] args) {
SortedDictionary<int, string> person = new SortedDictionary<int, string>();
person.Add(1, "ABC");
person.Add(2, "DEF");
person.Add(3, "GHI");
foreach (var pair in person) {
Console.WriteLine(pair);
}
}
}
}
Output:
[1, ABC]
[2, DEF]
[3, GHI]
In the above code, we create the sorted dictionary person
with the SortedDictionary<int, string>
class in C#. We pass data into the person
dictionary in the form of key-value
pairs with the SortedDictionary.Add()
function. In the end, we print the data inside the person
dictionary with a foreach
loop.
C++ unordered_map<key, value>
Equivalent in C#
When we talk about the unordered_map<key, value>
data structure in C++, we are only concerned with storing the data in the form of key-value
pairs and not concerned with the order of the pairs. In this case, we can utilize the Dictionary<Tkey, Tvalue>
class to store data in the form of key-value
pairs in C#. See the below example.
using System;
using System.Collections.Generic;
namespace C__map_alternative {
class Program {
static void Main(string[] args) {
Dictionary<int, string> person = new Dictionary<int, string>();
person.Add(1, "ABC");
person.Add(2, "DEF");
person.Add(3, "GHI");
foreach (var pair in person) {
Console.WriteLine(pair);
}
}
}
}
Output:
[1, ABC]
[2, DEF]
[3, GHI]
In the above code, we create the unsorted dictionary person
with the Dictionary<int, string>
class in C#. We pass the data into the person
dictionary in the form of key-value
pairs with the Dictionary.Add()
function. In the end, we print the data inside the person
dictionary with a foreach
loop.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn