C# 中等效的 C++ Map<T1, T2>
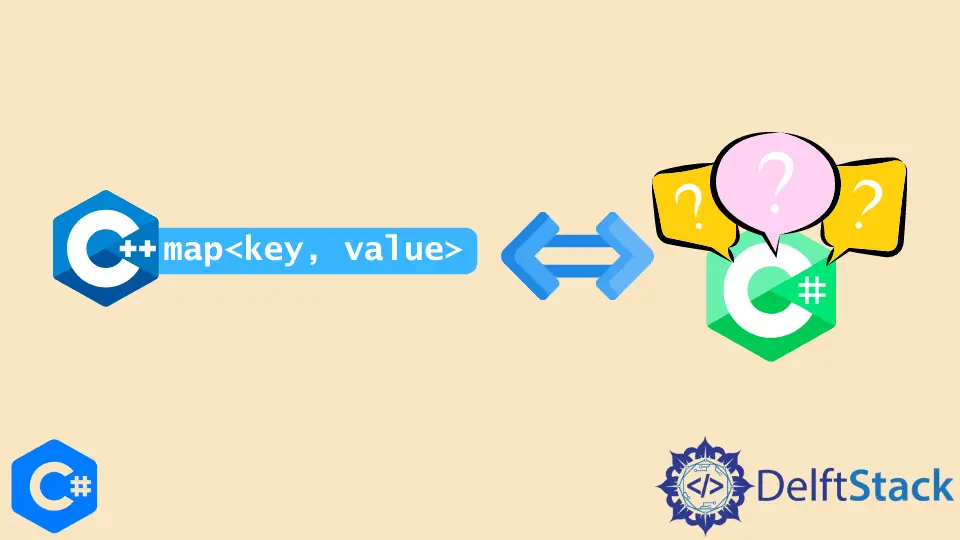
本教程将介绍 C# 中相当于 C++ 中的 map<T1, T2>
的数据类型。
C++map<key, value>
在 C# 中等效
C++ 中的 map<key, value>
数据结构用于以 key-value
对的形式保存数据。与此最接近的替代方法是 C# 中的 Dictionary<Tkey, Tvalue>
类。Dictionary
数据结构在 C# 中也是以 key-value
对的形式保存数据。如果我们关心字典中条目的顺序,则可以使用 C# 中的 SortedDictionary<Tkey, Tvalue>
类。以下代码示例向我们展示了如何使用 C# 中的 SortedDictionary<Tkey, Tvalue>
类以 key-value
对的形式存储数据。
using System;
using System.Collections.Generic;
namespace C__map_alternative {
class Program {
static void Main(string[] args) {
SortedDictionary<int, string> person = new SortedDictionary<int, string>();
person.Add(1, "ABC");
person.Add(2, "DEF");
person.Add(3, "GHI");
foreach (var pair in person) {
Console.WriteLine(pair);
}
}
}
}
输出:
[1, ABC]
[2, DEF]
[3, GHI]
在上面的代码中,我们使用 C# 中的 SortedDictionary<int, string>
类创建排序的字典 person
。我们通过 SortedDictionary.Add()
函数以键值
对的形式将数据传递到 person
字典中。最后,我们使用 foreach
循环在 person
字典中打印数据。
C++ unordered_map<key, value>
在 C# 中等效
当我们谈论 C++ 中的 unordered_map<key, value>
数据结构时,我们只关心以 key-value
对的形式存储数据,而不关心对中的顺序。在这种情况下,我们可以利用 Dictionary<Tkey, Tvalue>
类在 C# 中以 key-value
对的形式存储数据。请参见以下示例。
using System;
using System.Collections.Generic;
namespace C__map_alternative {
class Program {
static void Main(string[] args) {
Dictionary<int, string> person = new Dictionary<int, string>();
person.Add(1, "ABC");
person.Add(2, "DEF");
person.Add(3, "GHI");
foreach (var pair in person) {
Console.WriteLine(pair);
}
}
}
}
输出:
[1, ABC]
[2, DEF]
[3, GHI]
在上面的代码中,我们使用 C# 中的 Dictionary<int, string>
类创建未排序的字典 person
。我们通过 Dictionary.Add()
函数以键值
对的形式将数据传递到人
字典中。最后,我们使用 foreach
循环在 person
字典中打印数据。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn