How to Convert Double to Int in C#
-
Convert
double
toint
With Explicit Typecasting in C# -
Convert
double
toint
With theConvert.ToInt32()
Function in C# -
Convert
double
toint
With theMath.Round()
Function in C# -
Convert
double
toint
With theMath.Floor()
Function in C# -
Convert
double
toint
With theMath.Floor()
Function in C# - Conclusion
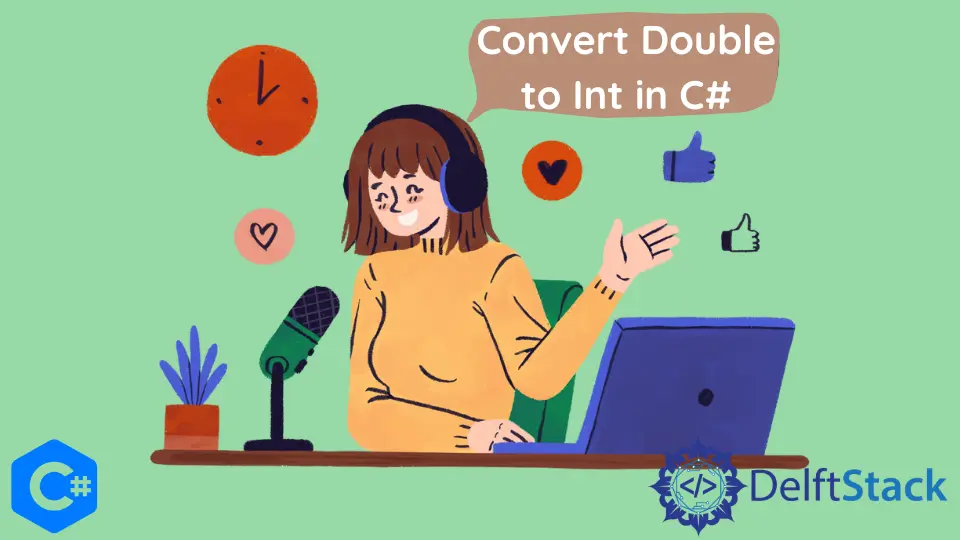
In C#, working with numeric data often involves the need to convert between different data types. When you have a double
and want to obtain an int
representation, you have several options at your disposal.
In this article, we’ll explore various methods for converting a double
value to an int
, each with its characteristics and use cases.
Convert double
to int
With Explicit Typecasting in C#
One of the simplest and most frequently used methods for converting a double
value to an int
is explicit casting or type casting. Before diving into the code, it’s essential to understand the key concepts behind explicit casting.
When you cast a double
to an int
, you’re essentially telling the compiler to treat the double
value as an int
. This operation will truncate the decimal part of the double
, converting it into a whole number.
It’s important to note that explicit casting may lead to data loss, as the fractional part of the double
will be discarded. If the double
value is negative, casting it to an int
will truncate the decimal and round towards zero (i.e., simply removing the decimal part without rounding up or down).
Let’s explore how to perform explicit casting to convert a double
to an int
in C#. Here’s a simple example:
double doubleValue = 3.75;
int intValue = (int)doubleValue;
In this code snippet, doubleValue
is the double
value you want to convert, and intValue
is the resulting int
value.
The (int)
inside the parentheses is the explicit cast operator, which tells the compiler to convert doubleValue
to an int
. The result is stored in intValue
.
Now, if you print the value of intValue
after this operation, you will get 3
because the decimal portion .75
was truncated.
Console.WriteLine(intValue);
Output:
3
When using explicit casting, be aware that you may lose data. For example:
double doubleValue = -3.75;
int intValue = (int)doubleValue;
In this case, intValue
will be -3
because casting a negative double
to an int
simply removes the decimal part without rounding. If you need rounding behavior, you should use other methods like Math.Floor
, Math.Ceiling
, or Math.Round
.
Convert double
to int
With the Convert.ToInt32()
Function in C#
The Convert.ToInt32()
method is part of the C# System
namespace and is designed to convert various types to a 32-bit signed integer (int
). It provides a straightforward way to convert a double
into an int
while taking care of any necessary rounding or truncation.
Here’s the syntax of the method:
public static int ToInt32(object value);
Here, value
is the object or value you want to convert to an int
. This can be of various types, including double
, string
, float
, long
, etc.
It returns an int
representing the converted value.
Let’s go through the steps to convert a double
to an int
using the Convert.ToInt32()
method:
double doubleValue = 3.75;
int intValue = Convert.ToInt32(doubleValue);
In the code above, doubleValue
is the double
value you want to convert, and intValue
is the resulting int
value. The Convert.ToInt32()
method takes care of the conversion, ensuring that the decimal portion of the double
is truncated and you receive a whole number in intValue
.
If you print the value of intValue
after this operation, it will be 3
, as the decimal .75
was truncated.
Console.WriteLine(intValue);
Output:
3
One advantage of using Convert.ToInt32()
is that it handles rounding correctly. For example, if the double
value is negative, it will round towards zero:
double doubleValue = -3.75;
int intValue = Convert.ToInt32(doubleValue);
In this case, intValue
will be -3
. The Convert.ToInt32()
method correctly rounds towards zero, ensuring you receive an int
that reflects the mathematical result.
It’s important to note that the Convert.ToInt32()
method may throw an OverflowException
if the double
value is too large to fit into a 32-bit int
. If the double
value exceeds the range of representable int
values, this exception will be raised.
You should handle this exception to ensure your code is robust and doesn’t crash due to overflow.
double doubleValue = 1e20;
try
{
int intValue = Convert.ToInt32(doubleValue);
Console.WriteLine(intValue);
}
catch (OverflowException ex)
{
Console.WriteLine($"Conversion error: {ex.Message}");
}
Convert double
to int
With the Math.Round()
Function in C#
The Math.Round()
method is part of the C# System
namespace, and it provides a way to round a floating-point number to the nearest integer. The method has several overloads to cater to different rounding strategies, but for converting a double
to an int
, we’ll focus on the simplest overload that rounds to the nearest even number in case of a tie.
Here’s the syntax for using this method:
double roundedValue = Math.Round(value);
Where:
roundedValue
: The variable that will hold the result of rounding thevalue
to the nearest integer.value
: The floating-point number (e.g.,double
,float
, ordecimal
) that you want to round.
The Math.Round()
method without additional parameters uses the default rounding behavior, which is banker’s rounding, meaning it rounds to the nearest even integer when the value is equidistant between two integers.
Let’s walk through the steps to convert a double
to an int
using Math.Round()
:
double doubleValue = 3.75;
int intValue = (int)Math.Round(doubleValue);
In this code snippet, doubleValue
is the double
value you want to convert, and intValue
is the resulting int
value. The Math.Round()
method is employed to round the double
value to the nearest integer.
The cast to int
is used to truncate the result to an int
.
If you print the value of intValue
after this operation, it will be 4
. This is because Math.Round()
rounds 3.75
to the nearest even integer, which is 4
.
Console.WriteLine(intValue);
Output:
4
As mentioned earlier, Math.Round()
uses banker’s rounding by default. This means that when rounding a value that is equidistant between two integers, it rounds to the nearest even integer.
For example:
double doubleValue = 2.5;
int intValue = (int)Math.Round(doubleValue);
In this case, intValue
will be 2
because 2.5
is rounded to the nearest even number, which is 2
.
If you need a different rounding behavior, you can use the other overloads of Math.Round()
. For example, you can specify MidpointRounding.AwayFromZero
to always round away from zero:
double doubleValue = 2.5;
int intValue = (int)Math.Round(doubleValue, MidpointRounding.AwayFromZero);
In this case, intValue
will be 3
because 2.5
is rounded away from zero to the next higher integer.
Convert double
to int
With the Math.Floor()
Function in C#
Math.Floor()
is a method provided by the System
namespace in C#. It is designed to round down a floating-point number to the nearest integer value that is less than or equal to the original number.
When using Math.Floor()
to convert a double
to an int
, you will essentially truncate the decimal portion of the double
.
Here’s the syntax for using this method:
double floorValue = Math.Floor(value);
Where:
floorValue
: The variable that will hold the result of rounding down thevalue
to the nearest integer.value
: The floating-point number (e.g.,double
,float
, ordecimal
) that you want to round down.
The Math.Floor()
method takes the value
as an argument and returns the greatest integer that is less than or equal to the value
.
Here’s how you can convert a double
to an int
using Math.Floor()
:
double doubleValue = 3.75;
int intValue = (int)Math.Floor(doubleValue);
In this code snippet, doubleValue
is the double
value you want to convert, and intValue
is the resulting int
value. The Math.Floor()
method is then used to round down the double
value to the nearest integer that is less than or equal to the original value.
The cast to int
is used to truncate the result to an int
.
If you print the value of intValue
after this operation, it will be 3
. This is because Math.Floor()
rounds 3.75
down to the nearest integer that is less than or equal to 3.75
, which is 3
.
Console.WriteLine(intValue);
Output:
3
It’s important to note that Math.Floor()
always rounds down, which means it takes the floor value of the number. If the double
value is negative, it will round down to the nearest integer that is less than or equal to the original value.
For example:
double doubleValue = -3.75;
int intValue = (int)Math.Floor(doubleValue);
In this case, intValue
will be -4
. This is because Math.Floor()
rounds down to the nearest integer that is less than or equal to -3.75
, which is -4
.
Convert double
to int
With the Math.Floor()
Function in C#
The Math.Ceiling()
method is part of the System
namespace in C#. It is used to round up a floating-point number to the nearest integer value that is greater than or equal to the original number.
When using Math.Ceiling()
to convert a double
to an int
, you will essentially round the value up to the nearest integer.
Here’s the syntax for using this method:
double ceilingValue = Math.Ceiling(value);
Where:
ceilingValue
: The variable that will hold the result of rounding up thevalue
to the nearest integer.value
: The floating-point number (e.g.,double
,float
, ordecimal
) that you want to round up.
The Math.Ceiling()
method takes the value
as an argument and returns the smallest integer that is greater than or equal to the value
. This effectively rounds the value up to the nearest integer, ensuring that the result is a whole number that is greater than or equal to the original value.
Here’s how you can convert a double
to an int
using Math.Ceiling()
:
double doubleValue = 3.75; // Your double value
int intValue = (int)Math.Ceiling(doubleValue);
In this code snippet, doubleValue
is the double
value you want to convert, and intValue
is the resulting int
value. The Math.Ceiling()
method is used to round up the double
value to the nearest integer that is greater than or equal to the original value.
The cast to int
is used to truncate the result to an int
.
If you print the value of intValue
after this operation, it will be 4
. This is because Math.Ceiling()
rounds 3.75
up to the nearest integer that is greater than or equal to 3.75
, which is 4
.
Console.WriteLine(intValue); // Output: 4
Math.Ceiling()
always rounds up, meaning it takes the ceiling value of the number. If the double
value is negative, it will round up to the nearest integer that is greater than or equal to the original value. For example:
double doubleValue = -3.75; // Negative double value
int intValue = (int)Math.Ceiling(doubleValue);
In this case, intValue
will be -3
. This is because Math.Ceiling()
rounds up to the nearest integer that is greater than or equal to -3.75
, which is -3
.
Conclusion
When converting from a double
to an int
, it’s crucial to consider potential data loss due to truncating the fractional part. Be cautious when dealing with large double
values that could lead to overflows when converted to an int
.
The appropriate method to use depends on your specific requirements for rounding and precision. If you need to ensure accurate rounding behavior, consider the use of Math.Floor
, Math.Ceiling
, or Math.Round
based on your rounding preferences.
For simple truncation without rounding, explicit casting can be used effectively. Choose the method that best aligns with your specific use case, whether you need precise rounding or simple truncation.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn