在 C# 中将双精度数转换为整数
- 在 C# 中使用显式类型转换将双精度值转换为整型
-
使用 C# 中的
Convert.ToInt32()
函数将 Double 转换为 Int -
使用 C# 中的
Math.Round()
函数将 Double 转换为 Int
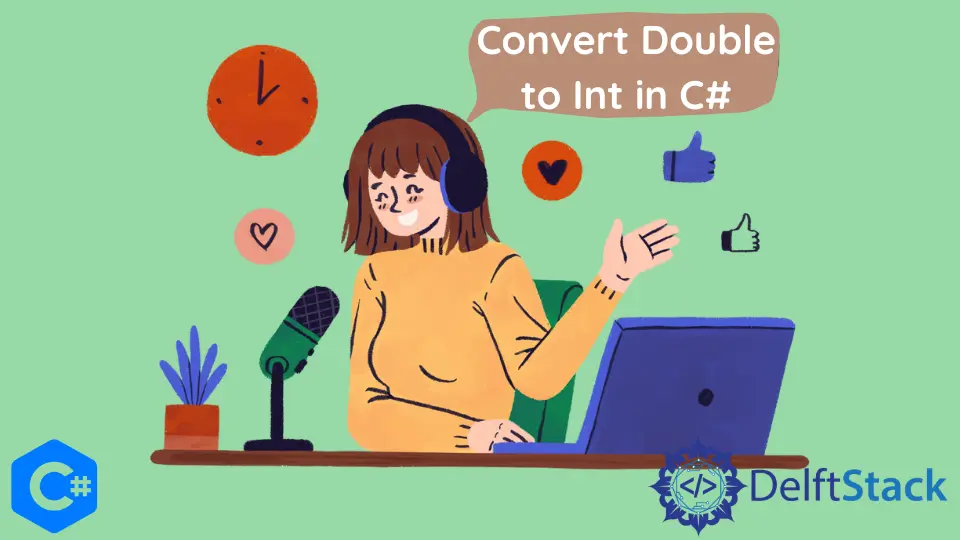
本教程将介绍在 C# 中将双精度值转换为 int 值的方法。
在 C# 中使用显式类型转换将双精度值转换为整型
众所周知,双精度数据类型比整数需要更多的字节。我们需要使用显式类型转换在 C# 中把一个双精度值转换为一个 int 值。下面的代码示例向我们展示了如何在 C# 中使用显式类型转换将 double 值转换为 int 值。
using System;
namespace convert_double_to_int {
class Program {
static void Main(string[] args) {
double d = 7.7;
int x = (int)d;
Console.WriteLine(x);
}
}
}
输出:
7
我们使用 C# 中的显式类型转换将双精度值 d
转换为整数值 x
。我们得到 7
作为输出,因为显式类型转换完全忽略了小数点后的值。不建议使用显式类型转换,因为在使用显式类型转换时会发生大量数据丢失。
使用 C# 中的 Convert.ToInt32()
函数将 Double 转换为 Int
Convert.ToInt32()
函数将值转换为整数值。Convert.ToInt32()
函数将值转换为等效的 32 位带符号整数。下面的代码示例向我们展示了如何使用 Convert.ToInt32()
函数在 C# 中将双精度值转换为整数值。
using System;
namespace convert_double_to_int {
class Program {
static void Main(string[] args) {
double d = 7.7;
int x = Convert.ToInt32(d);
Console.WriteLine(x);
}
}
}
输出:
8
在上面的代码中,我们使用 C# 中的 Convert.ToInt32()
函数将双精度值 d
转换为整数值 x
。
使用 C# 中的 Math.Round()
函数将 Double 转换为 Int
Math.Round()
函数用于将十进制值舍入为其最接近的整数值。Math.Round()
返回一个十进制值,四舍五入到最接近的整数值。以下代码示例向我们展示了如何使用 Math.Round()
函数在 C# 中将十进制值转换为整数值。
using System;
namespace convert_double_to_int {
class Program {
static void Main(string[] args) {
double d = 7.7;
int x = (int)Math.Round(d);
Console.WriteLine(x);
}
}
}
输出:
8
在上面的代码中,我们使用 C# 中的 Math.Round()
函数将双精度值 d
转换为整数值 x
。为了将值存储在整数变量 x
中,我们必须使用显式类型转换,因为 Math.Round()
函数返回一个双精度值。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn