在 C# 中将双精度值四舍五入为整数值
-
使用 C# 中的
Math.Ceiling()
函数将双精度值四舍五入为整数值 -
使用 C# 中的
Math.Floor()
函数将双精度值四舍五入为整数值 -
使用 C# 中的
Math.Round()
函数将双精度值四舍五入为整数值
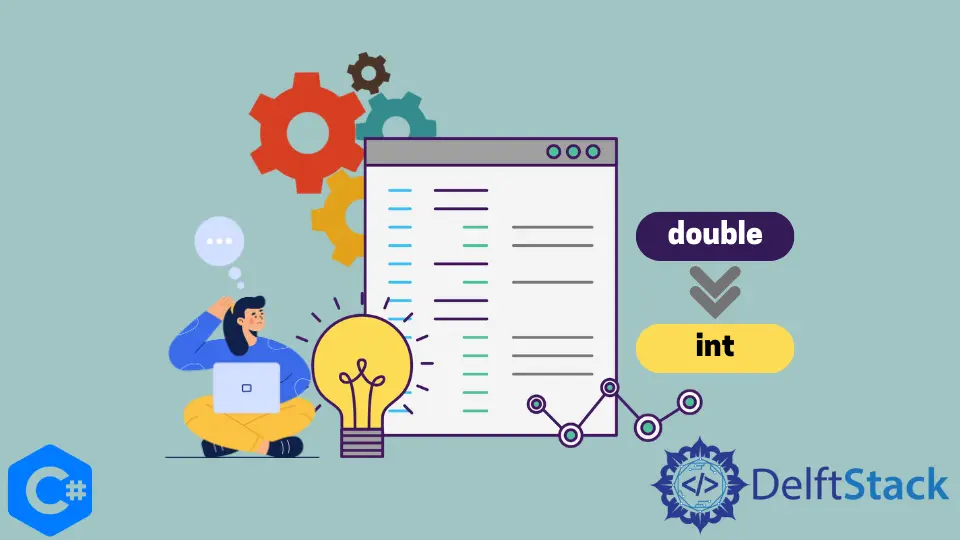
本教程将讨论在 C# 中将双精度值四舍五入为整数值的方法。
使用 C# 中的 Math.Ceiling()
函数将双精度值四舍五入为整数值
如果要将整数值 2.5
舍入为整数值 3
,则必须使用 Math.Ceiling()
函数。Math.Ceiling()
函数将十进制值四舍五入到下一个整数值。下面的代码示例向我们展示了如何使用 C# 中的 Math.Ceiling()
函数将双精度值四舍五入为整数值。
using System;
namespace round_double_to_intt {
class Program {
static void Main(string[] args) {
double d = 2.5;
int i = (int)Math.Ceiling(d);
Console.WriteLine("Original Value = {0}", d);
Console.WriteLine("Rounded Value = {0}", i);
}
}
}
输出:
Original Value = 2.5
Rounded Value = 3
我们使用 C# 中的 Math.Ceiling()
函数将双精度值 2.5
舍入为整数值 3
。这种方法的问题在于,Math.Ceiling()
函数会将小数值 2.3
转换为整数值 3
。
使用 C# 中的 Math.Floor()
函数将双精度值四舍五入为整数值
如果想将双精度值 2.5
舍入为整数值 2
,则必须使用 Math.Floor()
函数。Math.Floor()
函数将一个小数值舍入为前一个整数值。下面的代码示例向我们展示了如何使用 C# 中的 Math.Floor()
函数将双精度值四舍五入为整数值。
using System;
namespace round_double_to_intt {
class Program {
static void Main(string[] args) {
double d = 2.5;
int i = (int)Math.Floor(d);
Console.WriteLine("Original Value = {0}", d);
Console.WriteLine("Rounded Value = {0}", i);
}
}
}
输出:
Original Value = 2.5
Rounded Value = 2
我们使用 C# 中的 Math.Floor()
函数将双精度值 2.5
舍入为整数值 2
。这种方法的问题在于,Math.Floor()
函数会将十进制值 2.9
转换为整数值 2
。
使用 C# 中的 Math.Round()
函数将双精度值四舍五入为整数值
在 C# 中,Math.Round()
函数可用于将双精度值四舍五入最接近的整数值。Math.Round()
函数返回一个双精度值,该值将四舍五入到最接近的整数。下面的代码示例向我们展示了如何使用 C# 中的 Math.Round()
函数将双精度值四舍五入为整数值。
using System;
namespace round_double_to_intt {
class Program {
static void Main(string[] args) {
double d = 2.9;
int i = (int)Math.Round(d);
Console.WriteLine("Original Value = {0}", d);
Console.WriteLine("Rounded Value = {0}", i);
}
}
}
输出:
Original Value = 2.9
Rounded Value = 3
我们使用 C# 中的 Math.Round()
函数将小数值 2.9
舍入为整数值 3
。我们使用类型转换将 Math.Round()
函数返回的双精度值转换为整数值。这种方法只有一个问题。Math.Round()
函数将十进制值 2.5
转换为整数值 2
。
我们可以通过在 Math.Round()
函数的参数中指定 MidpointRounding.AwayFromZero
来解决此问题。下面的代码示例向我们展示了如何使用 C# 中的 Math.Round()
函数将 2.5
舍入为 3
。
using System;
namespace round_double_to_intt {
class Program {
static void Main(string[] args) {
double d = 2.5;
int i = (int)Math.Round(d, MidpointRounding.AwayFromZero);
Console.WriteLine("Original Value = {0}", d);
Console.WriteLine("Rounded Value = {0}", i);
}
}
}
输出:
Original Value = 2.5
Rounded Value = 3
通过在 C# 中的 Math.Round()
函数中指定 MidpointRounding.AwayFromZero
参数,将十进制值 2.5
舍入为整数值 3
。
上面讨论的所有方法在不同的特定情况下都是有用的。将双精度值四舍五入为整数值的最坏方法是通过显式类型转换。这是因为显式类型转换会忽略小数点后的所有值,而只返回小数点前的整数值。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn