How to Round a Double Value to an Integer Value in C#
-
Round Up a Double Value to an Integer Value With the
Math.Ceiling()
Function in C# -
Round a Double Value to an Integer Value With
Math.Round()
in C# -
Round a Double Value to an Integer in C# Using
Math.Floor
-
Round Up a Double Value to an Integer in C# With
Math.Truncate
andMath.Sign
- Conclusion
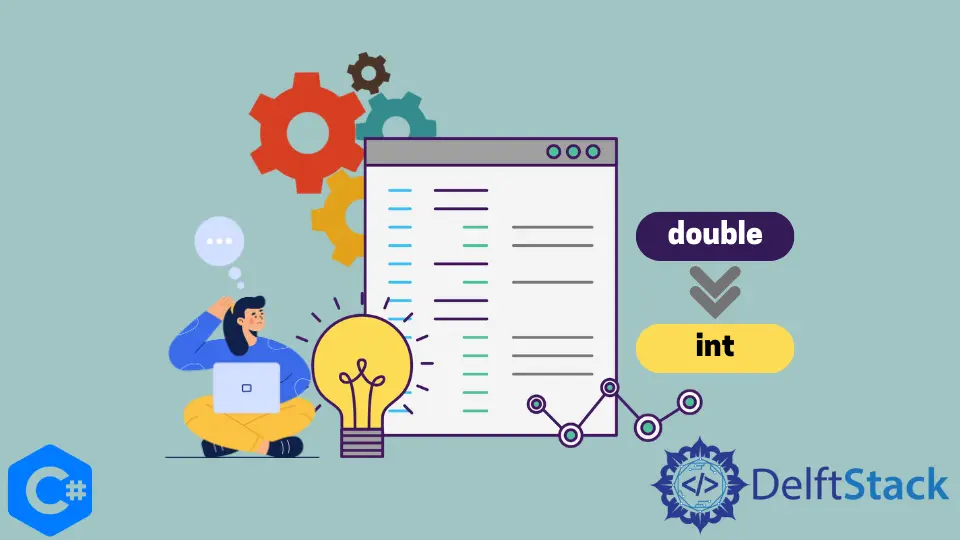
Rounding double values to integers is a common operation in programming, especially when dealing with mathematical calculations or financial applications. In C#, there are several methods available to achieve this task, each with its own characteristics and use cases.
In this guide, we’ll explore different approaches to round a double value to an integer in C#.
Round Up a Double Value to an Integer Value With the Math.Ceiling()
Function in C#
The Math.Ceiling()
function in C# returns the smallest integral value greater than or equal to the specified double-precision floating-point number. In simpler terms, it rounds up the given value to the nearest integer.
The method takes a double
parameter and returns a double
. To obtain an integer result, you can cast the result to an integer using (int)
.
Here’s a basic example demonstrating how to use Math.Ceiling()
to round up a double
value:
using System;
class Program {
static void Main() {
double doubleValue = 10.75;
int roundedValue = (int)Math.Ceiling(doubleValue);
Console.WriteLine($"Original value: {doubleValue}");
Console.WriteLine($"Rounded up value: {roundedValue}");
}
}
Output:
Original value: 10.75
Rounded up value: 11
In this example, the Math.Ceiling()
function is applied to the doubleValue
, and the result is cast to an integer. The output will display the original value and the rounded-up value.
Let’s consider a practical scenario where rounding up is commonly used - calculating the total cost of items in a shopping cart.
Assume you have a list of product prices stored as double
values, and you want to calculate the total cost:
using System;
using System.Linq;
class Program {
static void Main() {
double[] productPrices = { 12.99, 8.75, 24.50, 15.30 };
double totalCost = productPrices.Sum();
int roundedTotalCost = (int)Math.Ceiling(totalCost);
Console.WriteLine($"Total cost before rounding is {totalCost}");
Console.WriteLine($"Rounded total cost is {roundedTotalCost}");
}
}
Output:
Total cost before rounding is 61.54
Rounded total cost is 62
In this example, the Sum()
method is used to calculate the total cost of the products, and Math.Ceiling()
is applied to round up the total cost to the nearest integer.
The results are then displayed, showing the total cost before and after rounding.
Round a Double Value to an Integer Value With Math.Round()
in C#
The Math.Round
method in C# is part of the System
namespace and provides a flexible way to round a floating-point number to the nearest integral value. By default, Math.Round
uses the “round half to even” strategy, which means it rounds to the nearest even number in case of a tie (midpoint).
However, for our purposes, we’ll focus on rounding to the nearest integer.
Here is the basic syntax:
Math.Round(double value);
This version of Math.Round()
takes a double
value and rounds it to the nearest integer using the default rounding strategy, bankers’ rounding.
Let’s start with a basic example:
using System;
class Program {
static void Main() {
double doubleValue = 10.75;
int roundedValue = (int)Math.Round(doubleValue);
Console.WriteLine($"Original value: {doubleValue}");
Console.WriteLine($"Rounded value: {roundedValue}");
}
}
Output:
Original value: 10.75
Rounded value: 11
In this example, Math.Round
is applied to the doubleValue
, and the result is cast to an integer. The output will display the original value and the rounded value.
Custom Rounding Strategies With Math.Round
Additionally, Math.Round()
has other overloaded versions that allow you to customize the rounding behavior. Here is an example of a more complex syntax:
Math.Round(double value, int decimals, MidpointRounding mode);
Where:
value
: The number to be rounded.decimals
: The number of decimal places to round to.mode
: An enumeration of typeMidpointRounding
that specifies how to round the value in case of a tie (midpoint).
You can customize the rounding behavior by using this overloaded version of Math.Round
. For example, you can specify the number of decimal places to round to or use the MidpointRounding
enumeration to control the rounding strategy.
using System;
class Program {
static void Main() {
double doubleValue = 10.75;
// Round to 1 decimal place
double roundedToOneDecimal = Math.Round(doubleValue, 1);
int roundedAwayFromZero = (int)Math.Round(doubleValue, MidpointRounding.AwayFromZero);
Console.WriteLine($"Original value: {doubleValue}");
Console.WriteLine($"Rounded to 1 decimal place: {roundedToOneDecimal}");
Console.WriteLine($"Rounded away from zero: {roundedAwayFromZero}");
}
}
Output:
Original value: 10.75
Rounded to 1 decimal place: 10.8
Rounded away from zero: 11
In this example, we round doubleValue
to one decimal place and also demonstrate rounding away from zero using MidpointRounding.AwayFromZero
.
Dealing With Ties and MidpointRounding
When dealing with midpoint values (those exactly halfway between two integers), the default behavior of Math.Round
is to round to the nearest even number. However, you can customize this behavior with the MidpointRounding
enumeration.
using System;
class Program {
static void Main() {
double midpointValue = 0.5;
// Default behavior (round to the nearest even number)
int roundedDefault = (int)Math.Round(midpointValue);
int roundedAwayFromZero = (int)Math.Round(midpointValue, MidpointRounding.AwayFromZero);
Console.WriteLine($"Midpoint value: {midpointValue}");
Console.WriteLine($"Rounded (default): {roundedDefault}");
Console.WriteLine($"Rounded away from zero: {roundedAwayFromZero}");
}
}
Output:
Midpoint value: 0.5
Rounded (default): 0
Rounded away from zero: 1
In this example, we demonstrate the default behavior and rounding away from zero for a midpoint value.
Round a Double Value to an Integer in C# Using Math.Floor
The Math.Floor
method in C# is part of the System
namespace and is designed to return the largest integer less than or equal to the specified number. When applied to a positive double
value, Math.Floor
effectively removes the fractional part, rounding down to the nearest whole number.
Let’s have a basic example of using Math.Floor
to round a double
value down to the nearest integer:
using System;
class Program {
static void Main() {
double doubleValue = 10.75;
int roundedValue = (int)Math.Floor(doubleValue);
Console.WriteLine($"Original value: {doubleValue}");
Console.WriteLine($"Rounded down value: {roundedValue}");
}
}
Output:
Original value: 10.75
Rounded down value: 10
In this example, Math.Floor
is applied to the doubleValue
, and the result is cast to an integer. The output will display the original value and the rounded-down value.
Now, consider a scenario where you have a quantity of items represented as a double
value, and you want to round it down to the nearest whole number:
using System;
class Program {
static void Main() {
double quantity = 7.8;
int roundedQuantity = (int)Math.Floor(quantity);
Console.WriteLine($"Original quantity: {quantity}");
Console.WriteLine($"Rounded down quantity: {roundedQuantity}");
}
}
Output:
Original quantity: 7.8
Rounded down quantity: 7
Here, Math.Floor
is used to round down the quantity
to the nearest integer. The results are then displayed, showing the original quantity and the rounded-down quantity.
Round Up a Double Value to an Integer in C# With Math.Truncate
and Math.Sign
The Math.Truncate
function in C# returns the integral part of a specified double-precision floating-point number. This effectively removes the fractional part of the number, leaving only the integer component.
On the other hand, Math.Sign
returns an integer that indicates the sign of a double-precision floating-point number.
By combining these two functions, we can round a positive double
value up to the nearest integer. The Math.Truncate
function removes the decimal part, and the Math.Sign
ensures that we round up rather than down for positive numbers.
Here’s a simple example demonstrating how to use Math.Truncate
and Math.Sign
to round up a double
value:
using System;
class Program {
static void Main() {
double doubleValue = 10.75;
int roundedValue = (int)(Math.Truncate(doubleValue) + Math.Sign(doubleValue));
Console.WriteLine($"Original value: {doubleValue}");
Console.WriteLine($"Rounded up value: {roundedValue}");
}
}
Output:
Original value: 10.75
Rounded up value: 11
In this example, Math.Truncate
is applied to remove the decimal part of doubleValue
, and Math.Sign
ensures that we round up for positive numbers. The result is cast to an integer to obtain the rounded-up value.
The output will display the original value and the rounded-up value.
Consider a scenario where you have a quantity of items represented as a double
value, and you want to round it up to the nearest whole number:
using System;
class Program {
static void Main() {
double quantity = 7.3;
int roundedQuantity = (int)(Math.Truncate(quantity) + Math.Sign(quantity));
Console.WriteLine($"Original quantity: {quantity}");
Console.WriteLine($"Rounded up quantity: {roundedQuantity}");
}
}
Output:
Original quantity: 7.3
Rounded up quantity: 8
In this example, Math.Truncate
is used to remove the decimal part of the quantity
, and Math.Sign
ensures that the rounding is done towards positive infinity.
The results are then displayed, showing the original quantity and the rounded-up quantity.
Conclusion
We have discussed the different methods for rounding double values to integers in C#. Whether utilizing the straightforward Math.Ceiling()
for rounding up, the versatile Math.Round()
for customizable rounding strategies, the precise Math.Floor()
for rounding down, or the combination of Math.Truncate()
and Math.Sign()
for rounding up positive values, you now have a range of tools at your disposal.
The choice of method depends on the specific needs of the application, offering flexibility to handle diverse scenarios such as calculating costs in a shopping cart or dealing with quantities of items.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn