How to Convert JSON String to C# Object
-
Convert a JSON String to a C# Object Using
Newtonsoft.Json
- Convert a JSON String to a C# Object Using JavaScriptSerializer
- Convert a JSON String to a C# Object Code Example
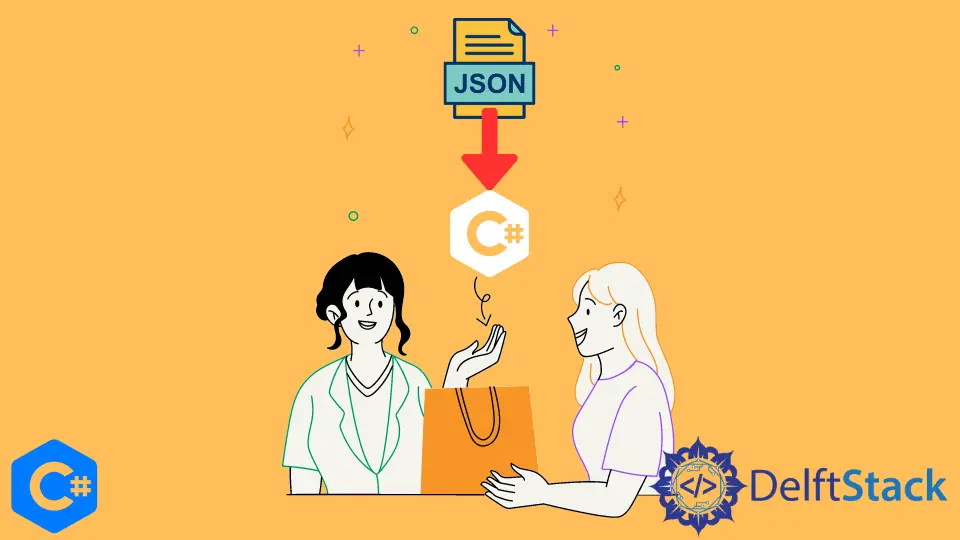
This tutorial will demonstrate how to convert a JSON string to a C# Object using two different methods.
The first uses the Newtonsoft.Json
library, while the second uses the JavaScriptSerializer
. Both methods are similar but use slightly different syntax and require different references.
Convert a JSON String to a C# Object Using Newtonsoft.Json
The Newtonsoft.Json
library is a commonly used JSON Framework for .NET
. This library can be utilized after installing the package to your solution. You can use the .NET CLI with the command below to install the package.
> dotnet add package Newtonsoft.Json --version 13.0.1
A JSON string can be converted to any type of C# Object as long as it is a valid JSON string for the object type. This is done by de-serializing the string, casting it to the specified type (T), and supplying the input JSON string.
JsonConvert.DeserializeObject<T>(json_string);
Convert a JSON String to a C# Object Using JavaScriptSerializer
An older option to convert JSON strings to C# Objects is JavaScriptSerializer
. While it is not as fast as the Newtonsoft.Json
solution, it can still be utilized well. To use this method, you need to add a reference to System.Web.Extensions.dll
to your project.
To add the reference, follow the steps below.
-
Navigate to the Solution Explorer
-
Right-click on References
-
Click
Add Reference
-
Click the
Assemblies
tab on the left -
Find the
System.Web.Extensions.dll
file and clickOK
.
The JavaScriptSerializer
works similarly to the Newtonsoft.Json
method in that it requires just the JSON string and the type to be de-serialized into.
JavaScriptSerializer().Deserialize<T>(json_string)
Convert a JSON String to a C# Object Code Example
The code example below demonstrates how to convert a JSON string to a C# Object of any type using both the JsonConvert.DeserializeObject<T>
and JavaScriptSerializer
functions. For this example, we are converting strings to a list object containing integers ( List<Int32>
) and to a custom class that we specified in the program as well.
using Newtonsoft.Json;
using System.Data;
using System;
using System.ComponentModel;
using System.Collections.Generic;
using System.Web.Script.Serialization;
namespace JSON_To_Object_Example {
class Program {
static void Main(string[] args) {
// Declare the JSON Strings
string list_str = "[1, 2, 3, 9, 8, 7]";
string custom_class_str =
"{ \"Name\":\"John Doe\",\"Age\":21,\"Birthday\":\"2000-01-01T00:00:00\"}";
// ----- Newtonsoft Method -----
// Deserialize the string by specifying the object type
List<Int32> list_converted_ns = JsonConvert.DeserializeObject<List<Int32>>(list_str);
// Print the contents of the newly converted List object to the Console.
Console.WriteLine("Converted List - Newtonsoft: " + String.Join(", ", list_converted_ns) +
"\n");
// The same can be done for any type of C# object, including custom classes. Just change the
// specified Type.
Custom_Class class_converted_ns =
JsonConvert.DeserializeObject<Custom_Class>(custom_class_str);
Console.WriteLine("Converted Class - Newtonsoft:");
PrintPropreties(class_converted_ns);
Console.WriteLine("\n\n");
// ----- JavaScriptSerializer Method -----
// Deserialize the string by specifying the object type
List<Int32> list_converted_js = new JavaScriptSerializer().Deserialize<List<Int32>>(list_str);
Console.WriteLine(
"Converted List - JavaScriptSerializer: " + String.Join(", ", list_converted_js) + "\n");
Custom_Class class_converted_js =
new JavaScriptSerializer().Deserialize<Custom_Class>(custom_class_str);
Console.WriteLine("Converted Class - JavaScriptSerializer:");
PrintPropreties(class_converted_js);
Console.ReadLine();
}
public class Custom_Class {
// Custom Class created for the purposes of this tutorial
public string Name { get; set; }
public int Age { get; set; }
public DateTime Birthday { get; set; }
}
public static void PrintPropreties(object obj) {
// Uses the System.Component Model to read each property of a class and print its value
foreach (PropertyDescriptor descriptor in TypeDescriptor.GetProperties(obj)) {
string name = descriptor.Name;
object value = descriptor.GetValue(obj);
Console.WriteLine("{0}: {1}", name, value);
}
}
}
}
This code will output the integer list joined by commas and all the properties within the converted Custom Class. You can observe that both methods will produce the same output.
Output:
Converted List - Newtonsoft: 1, 2, 3, 9, 8, 7
Converted Class - Newtonsoft:
Name: John Doe
Age: 21
Birthday: 01/01/2000 12:00:00 am
Converted List - JavaScriptSerializer: 1, 2, 3, 9, 8, 7
Converted Class - JavaScriptSerializer:
Name: John Doe
Age: 21
Birthday: 01/01/2000 12:00:00 am
Related Article - Csharp JSON
- C# Parse JSON in C#
- How to Convert the Object to a JSON String in C#
- How to Deserialize JSON With C#
- How to Write JSON Into File in C#
- How to Post JSON to a Server in C#