JSON 문자열을 C# 개체로 변환
-
Newtonsoft.Json
을 사용하여 JSON 문자열을 C# 개체로 변환 - JavaScriptSerializer를 사용하여 JSON 문자열을 C# 개체로 변환
- JSON 문자열을 C# 개체 코드 예제로 변환
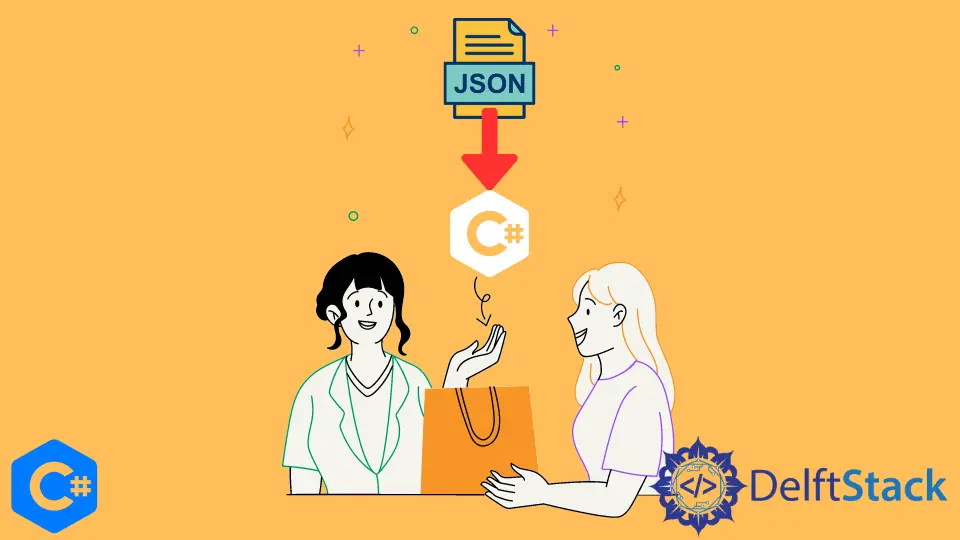
이 자습서에서는 두 가지 방법을 사용하여 JSON 문자열을 C# 개체로 변환하는 방법을 보여줍니다.
첫 번째는 Newtonsoft.Json
라이브러리를 사용하고 두 번째는 JavaScriptSerializer
를 사용합니다. 두 방법 모두 비슷하지만 약간 다른 구문을 사용하고 다른 참조가 필요합니다.
Newtonsoft.Json
을 사용하여 JSON 문자열을 C# 개체로 변환
Newtonsoft.Json
라이브러리는 .NET
용으로 일반적으로 사용되는 JSON 프레임워크입니다. 이 라이브러리는 솔루션에 패키지를 설치한 후 사용할 수 있습니다. 아래 명령과 함께 .NET CLI를 사용하여 패키지를 설치할 수 있습니다.
> dotnet add package Newtonsoft.Json --version 13.0.1
JSON 문자열은 개체 유형에 대해 유효한 JSON 문자열인 한 모든 유형의 C# 개체로 변환할 수 있습니다. 이것은 문자열을 역직렬화하고 지정된 유형(T)으로 캐스팅하고 입력 JSON 문자열을 제공하여 수행됩니다.
JsonConvert.DeserializeObject<T>(json_string);
JavaScriptSerializer를 사용하여 JSON 문자열을 C# 개체로 변환
JSON 문자열을 C# 개체로 변환하는 이전 옵션은 JavaScriptSerializer
입니다. Newtonsoft.Json
솔루션만큼 빠르지는 않지만 여전히 잘 활용될 수 있습니다. 이 방법을 사용하려면 System.Web.Extensions.dll
에 대한 참조를 프로젝트에 추가해야 합니다.
참조를 추가하려면 아래 단계를 따르세요.
-
솔루션 탐색기로 이동
-
참조를 마우스 오른쪽 버튼으로 클릭
-
참조 추가
를 클릭합니다. -
왼쪽의
Assemblies
탭을 클릭합니다. -
System.Web.Extensions.dll
파일을 찾아확인
을 클릭합니다.
JavaScriptSerializer
는 JSON 문자열과 역직렬화할 유형만 필요하다는 점에서 Newtonsoft.Json
메소드와 유사하게 작동합니다.
JavaScriptSerializer().Deserialize<T>(json_string)
JSON 문자열을 C# 개체 코드 예제로 변환
아래 코드 예제는 JsonConvert.DeserializeObject<T>
및 JavaScriptSerializer
함수를 모두 사용하여 JSON 문자열을 모든 유형의 C# 개체로 변환하는 방법을 보여줍니다. 이 예에서는 문자열을 정수를 포함하는 목록 개체( List<Int32>
)와 프로그램에서 지정한 사용자 지정 클래스로 변환합니다.
using Newtonsoft.Json;
using System.Data;
using System;
using System.ComponentModel;
using System.Collections.Generic;
using System.Web.Script.Serialization;
namespace JSON_To_Object_Example {
class Program {
static void Main(string[] args) {
// Declare the JSON Strings
string list_str = "[1, 2, 3, 9, 8, 7]";
string custom_class_str =
"{ \"Name\":\"John Doe\",\"Age\":21,\"Birthday\":\"2000-01-01T00:00:00\"}";
// ----- Newtonsoft Method -----
// Deserialize the string by specifying the object type
List<Int32> list_converted_ns = JsonConvert.DeserializeObject<List<Int32>>(list_str);
// Print the contents of the newly converted List object to the Console.
Console.WriteLine("Converted List - Newtonsoft: " + String.Join(", ", list_converted_ns) +
"\n");
// The same can be done for any type of C# object, including custom classes. Just change the
// specified Type.
Custom_Class class_converted_ns =
JsonConvert.DeserializeObject<Custom_Class>(custom_class_str);
Console.WriteLine("Converted Class - Newtonsoft:");
PrintPropreties(class_converted_ns);
Console.WriteLine("\n\n");
// ----- JavaScriptSerializer Method -----
// Deserialize the string by specifying the object type
List<Int32> list_converted_js = new JavaScriptSerializer().Deserialize<List<Int32>>(list_str);
Console.WriteLine(
"Converted List - JavaScriptSerializer: " + String.Join(", ", list_converted_js) + "\n");
Custom_Class class_converted_js =
new JavaScriptSerializer().Deserialize<Custom_Class>(custom_class_str);
Console.WriteLine("Converted Class - JavaScriptSerializer:");
PrintPropreties(class_converted_js);
Console.ReadLine();
}
public class Custom_Class {
// Custom Class created for the purposes of this tutorial
public string Name { get; set; }
public int Age { get; set; }
public DateTime Birthday { get; set; }
}
public static void PrintPropreties(object obj) {
// Uses the System.Component Model to read each property of a class and print its value
foreach (PropertyDescriptor descriptor in TypeDescriptor.GetProperties(obj)) {
string name = descriptor.Name;
object value = descriptor.GetValue(obj);
Console.WriteLine("{0}: {1}", name, value);
}
}
}
}
이 코드는 변환된 사용자 정의 클래스 내의 모든 속성과 쉼표로 결합된 정수 목록을 출력합니다. 두 방법 모두 동일한 출력을 생성하는 것을 관찰할 수 있습니다.
출력:
Converted List - Newtonsoft: 1, 2, 3, 9, 8, 7
Converted Class - Newtonsoft:
Name: John Doe
Age: 21
Birthday: 01/01/2000 12:00:00 am
Converted List - JavaScriptSerializer: 1, 2, 3, 9, 8, 7
Converted Class - JavaScriptSerializer:
Name: John Doe
Age: 21
Birthday: 01/01/2000 12:00:00 am