C#에서 개체 삭제
Muhammad Maisam Abbas
2024년2월16일
Csharp
Csharp Object
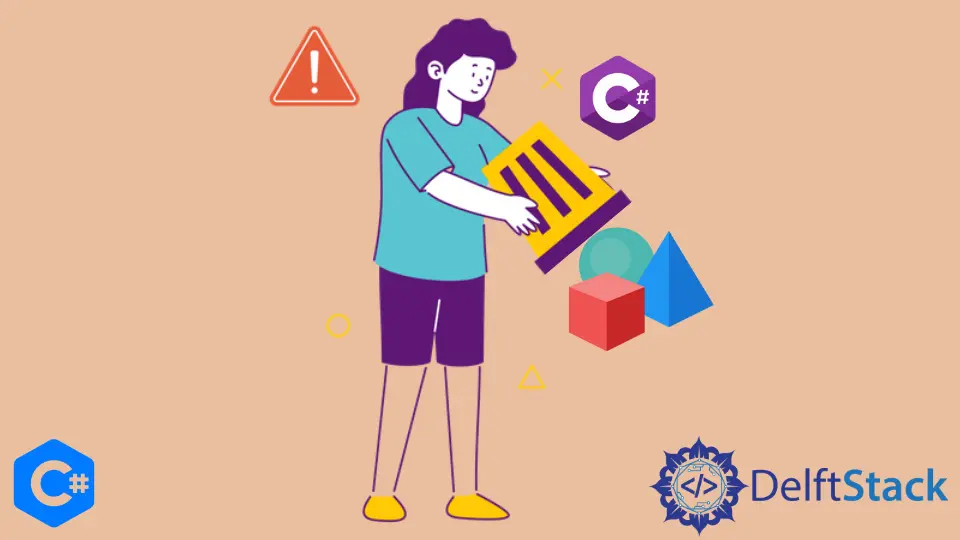
이 자습서에서는 C#에서 사용자 정의 클래스의 개체를 삭제하는 방법에 대해 설명합니다.
null
값을 할당하여 C#에서 사용자 정의 클래스 개체 삭제
클래스 객체는 해당 클래스의 메모리 위치를 가리키는 참조 변수입니다. null
값을 할당하여 객체를 삭제할 수 있습니다. 이는 현재 객체에 메모리 위치에 대한 참조가 없음을 의미합니다. 다음 예를 참조하십시오.
using System;
namespace delete_object {
public class Sample {
public string value { get; set; }
}
class Program {
static void Main(string[] args) {
Sample x = new Sample();
x.value = "Some Value";
x = null;
Console.WriteLine(x.value);
}
}
}
출력:
Unhandled Exception: System.NullReferenceException: Object reference not set to an instance of an object.
위 코드에서Sample
클래스의 객체x
를 초기화하고value
속성에 값을 할당했습니다. 그런 다음x
에null
을 할당하여 객체를 삭제하고x.value
속성을 인쇄했습니다. x
가 메모리 위치를 가리 키지 않기 때문에 예외가 발생합니다.
또 다른 유익한 접근 방식은 개체를 삭제 한 후 가비지 수집기를 호출하는 것입니다. 이 접근 방식은 아래 코드 예제에 설명되어 있습니다.
using System;
namespace delete_object {
public class Sample {
public string value { get; set; }
}
class Program {
static void Main(string[] args) {
Sample x = new Sample();
x.value = "Some Value";
x = null;
GC.Collect();
Console.WriteLine(x.value);
}
}
}
위의 코드에서는 C#의 GC.Collect()
메서드를 사용하여x
개체에null
값을 할당 한 후 가비지 수집기를 호출했습니다.
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn