How to Delete Object in C#
-
Assign
null
to the Object to Delete a User-Defined Class Object in C# - Utilize Garbage Collection to Delete a User-Defined Class Object in C#
- Conclusion
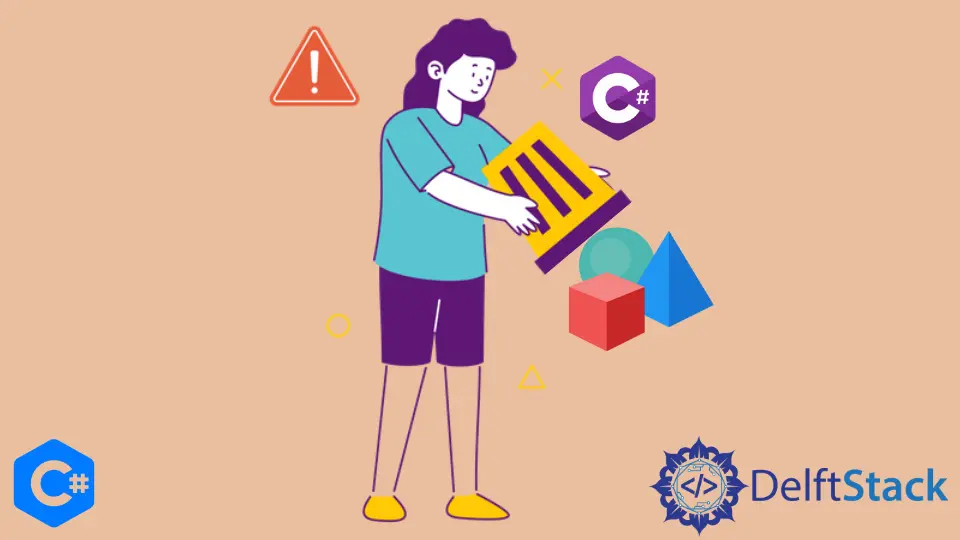
In C#, user-defined class objects are reference variables that point to specific memory locations. Deleting such objects involves removing the reference to their associated memory location.
This tutorial will explore the methods to delete a user-defined class object in C# effectively.
Assign null
to the Object to Delete a User-Defined Class Object in C#
A user-defined class object can be deleted by assigning the null
value to it. This action severs the connection between the object and its memory location.
Below is an example:
using System;
namespace DeleteObjectDemo {
public class Sample {
public string Value { get; set; }
}
class Program {
static void Main(string[] args) {
Sample x = new Sample();
x.Value = "Some Value";
x = null; // Deleting the object by assigning null
// Console.WriteLine(x.Value); // This line will throw a NullReferenceException
}
}
}
Output:
Unhandled Exception: System.NullReferenceException: Object reference not set to an instance of an object.
In the above code, we created an object x
of the Sample
class, assigned a value to its Value
property, and then deleted the object by setting it to null
.
Assigning the null
value to the variable severs the connection between the x
variable and the memory location where the object resides. The object becomes eligible for garbage collection because there are no more references to it.
Attempting to access any property or method of the deleted object (in this case, x.Value
) will result in a NullReferenceException
. This exception occurs because x
no longer points to a valid object in memory.
Utilize Garbage Collection to Delete a User-Defined Class Object in C#
Another beneficial approach is to call the garbage collector after deleting the object. This ensures that any resources associated with the object are properly released.
Here’s how you can do it:
using System;
namespace DeleteObjectDemo {
public class Sample {
public string Value { get; set; }
}
class Program {
static void Main(string[] args) {
Sample x = new Sample();
x.Value = "Some Value";
x = null; // Deleting the object by assigning null
GC.Collect(); // Calling the garbage collector
// Console.WriteLine(x.Value); // Accessing x.Value after this point may lead to unexpected
// behavior
}
}
}
In this code, we invoke the garbage collector using GC.Collect()
after setting x
to null
. The garbage collector is responsible for identifying and freeing up memory that is no longer in use.
In this case, it recognizes that the object referred to by x
is no longer accessible. The garbage collector then reclaims the memory occupied by the deleted object and releases any associated resources.
As with the first method, attempting to access properties or methods of the deleted object after garbage collection may lead to unexpected behavior or exceptions, such as NullReferenceException
. This is because, after garbage collection, the object is no longer available in memory.
Implement IDisposable
for Explicit Resource Cleanup
While C# and the .NET runtime handle memory management automatically, there are situations where you need to clean up resources explicitly, such as file handles, database connections, or unmanaged resources. In such cases, it’s a good practice to implement the IDisposable
interface in your user-defined classes.
Here’s an example of how to implement IDisposable
:
using System;
public class Sample : IDisposable {
public string Value { get; set; }
public void Dispose() {
// Clean up resources associated with the Sample object (if any)
Console.WriteLine($"Disposing Sample with Value: {Value}");
}
}
public class MyClass : IDisposable {
private IntPtr unmanagedResource;
private AnotherDisposableObject disposableField;
private Sample sampleObject; // Add Sample object
public MyClass() {
unmanagedResource = SomeNativeLibrary.AllocateResource();
disposableField = new AnotherDisposableObject();
sampleObject = new Sample(); // Initialize Sample object
sampleObject.Value = "Some Value"; // Set its value
}
public void Dispose() {
if (unmanagedResource != IntPtr.Zero) {
SomeNativeLibrary.ReleaseResource(unmanagedResource);
unmanagedResource = IntPtr.Zero;
}
disposableField.Dispose();
sampleObject.Dispose(); // Dispose of the Sample object
GC.SuppressFinalize(this);
}
~MyClass() {
Dispose();
}
}
public class AnotherDisposableObject : IDisposable {
public void Dispose() {
// Clean up resources associated with AnotherDisposableObject (if any)
}
}
public static class SomeNativeLibrary {
public static IntPtr AllocateResource() {
return IntPtr.Zero;
}
public static void ReleaseResource(IntPtr resource) {
// Simulate releasing the unmanaged resource
}
}
class Program {
static void Main() {
using (var myObject = new MyClass()) {
// Use myObject and its resources here
// ...
} // Dispose is automatically called when exiting the using block
}
}
In this example:
-
The
MyClass
class implements theIDisposable
interface to manage the cleanup of unmanaged resources (unmanagedResource
) and a disposable field (disposableField
). -
The
AnotherDisposableObject
class is another disposable object that also implementsIDisposable
for resource cleanup. -
The
SomeNativeLibrary
class simulates a native library with resource allocation and release methods. -
In the
Main
method, we use theusing
statement to automatically dispose of theMyClass
instance when it goes out of scope, ensuring proper cleanup of resources.
Remember to replace the simulated resource allocation and release logic in the SomeNativeLibrary
class with actual code that deals with unmanaged resources.
Conclusion
Both methods are effective for deleting objects, but it’s important to understand the implications of deleting an object and the role of garbage collection in managing memory resources. Method 2 is especially useful when the object holds significant resources that should be released promptly.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn