C#에서 XML을 개체로 역직렬화
Abdullahi Salawudeen
2024년2월15일
Csharp
Csharp Object
- 수동으로 입력된 클래스를 사용하여 XML 파일을 C# 개체로 역직렬화
-
Visual Studio의
선택하여 붙여넣기
기능을 사용하여Xml
을 C# 개체로 역직렬화 -
XSD 도구
를 사용하여XML
을 C# 개체로 역직렬화
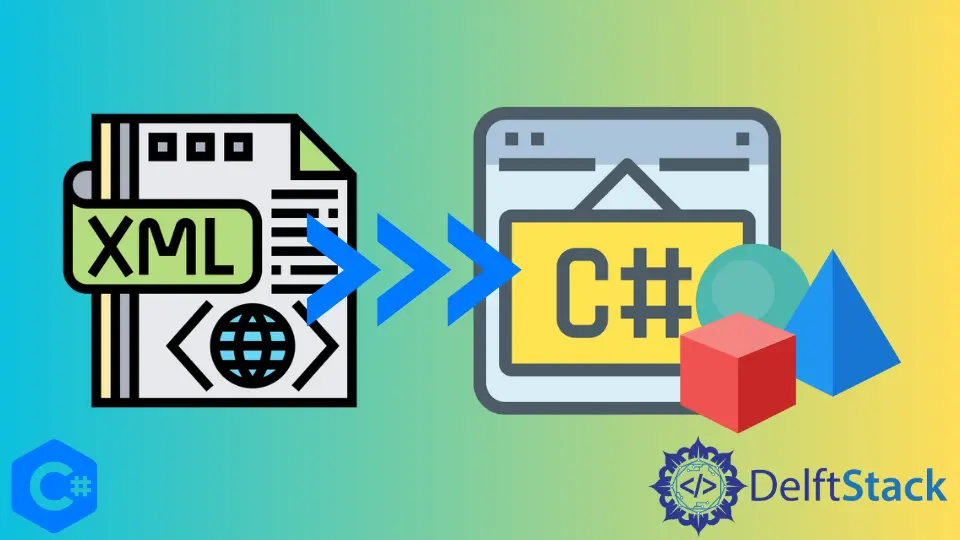
이 기사에서는 XML
파일을 C# 개체로 변환하거나 역직렬화하는 방법을 보여줍니다.
수동으로 입력된 클래스를 사용하여 XML 파일을 C# 개체로 역직렬화
- C#은
속성
및메소드
와 함께클래스
및객체
와 연결됩니다. Objects
는John
또는James
와 같은 프로그래밍 방식으로 클래스로 표시됩니다.속성
은자동차의 색상
,생산 연도
,사람의 나이
또는건물의 색상
과 같은 개체의 특성입니다.XML
은XML
파일을 전송하는 매체에 관계없이XML
데이터를 구문 분석할 수 있는 표준화된 형식입니다.
추가 논의는 이 참조를 통해 가능합니다.
다음은 C# 개체로 변환될 XML
코드 샘플입니다.
1. <?xml version="1.0" encoding="utf-8"?>
2. <Company xmlns:xsi="https://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="https://www.w3.org/2001/XMLSchema">
3. <Employee name="x" age="30" />
4. <Employee name="y" age="32" />
5. </Company>
XML 코드 샘플을 변환하기 위해 유사한 구조의 클래스가 C#으로 생성됩니다.
using System.Xml.Serialization;
[XmlRoot(ElementName = "Company")]
public class Company
{
public Company() {
Employees = new List<Employee>();
}
[XmlElement(ElementName = "Employee")]
public List<Employee> Employees { get; set; }
public Employee this[string name] {
get {
return Employees.FirstOrDefault(
s => string.Equals(s.Name, name, StringComparison.OrdinalIgnoreCase));
}
}
}
public class Employee {
[XmlAttribute("name")]
public string Name { get; set; }
[XmlAttribute("age")]
public string Age { get; set; }
}
XML
개체를 C#으로 변환하는 마지막 단계는 System.Xml.Serialization.XmlSerializer
함수를 사용하여 개체를 직렬화하는 것입니다.
public T DeserializeToObject<T>(string filepath)
where T : class {
System.Xml.Serialization.XmlSerializer ser =
new System.Xml.Serialization.XmlSerializer(typeof(T));
using (StreamReader sr = new StreamReader(filepath)) {
return (T)ser.Deserialize(sr);
}
}
Visual Studio의 선택하여 붙여넣기
기능을 사용하여 Xml
을 C# 개체로 역직렬화
이 방법을 사용하려면 .Net Framework 4.5 이상과 함께 Microsoft Visual Studio 2012 이상을 사용해야 합니다. Visual Studio용 WCF 워크로드도 설치해야 합니다.
XML
문서의 내용을 클립보드에 복사해야 합니다.- 프로젝트 솔루션에 새로운
빈
클래스를 추가합니다. - 새 클래스 파일을 엽니다.
- IDE 메뉴 바에서
편집
버튼을 클릭합니다. - 드롭다운에서
선택하여 붙여넣기
를 선택합니다. - XML을 클래스로 붙여넣기를 클릭합니다.
Visual Studio에서 생성된 클래스를 사용하려면 Helpers
클래스를 만듭니다.
using System;
using System.IO;
using System.Web.Script.Serialization; // Add reference: System.Web.Extensions
using System.Xml;
using System.Xml.Serialization;
namespace Helpers {
internal static class ParseHelpers
{
private static JavaScriptSerializer json;
private static JavaScriptSerializer JSON {
get { return json ?? (json = new JavaScriptSerializer()); }
}
public static Stream ToStream(this string @this) {
var stream = new MemoryStream();
var writer = new StreamWriter(stream);
writer.Write(@this);
writer.Flush();
stream.Position = 0;
return stream;
}
public static T ParseXML<T>(this string @this)
where T : class {
var reader = XmlReader.Create(
@this.Trim().ToStream(),
new XmlReaderSettings() { ConformanceLevel = ConformanceLevel.Document });
return new XmlSerializer(typeof(T)).Deserialize(reader) as T;
}
public static T ParseJSON<T>(this string @this)
where T : class {
return JSON.Deserialize<T>(@this.Trim());
}
}
}
XSD 도구
를 사용하여 XML
을 C# 개체로 역직렬화
‘XSD’는 ‘XML’ 파일 또는 문서에 정의된 스키마와 동일한 ‘클래스’ 또는 ‘객체’를 자동으로 생성하는 데 사용됩니다.
XSD.exe
는 일반적으로 C:\Program Files (x86)\Microsoft SDKs\Windows\{version}\bin\NETFX {version} Tools\
경로에 있습니다. 추가 논의는 이 참조를 통해 가능합니다.
XML 파일이 C:\X\test.XML
경로에 저장되어 있다고 가정합니다.
다음은 XML
을 C# 클래스로 자동으로 역직렬화하기 위해 따라야 할 단계입니다.
- 검색 창에
개발자 명령 프롬프트
를 입력하고 클릭하여 엽니다. cd C:\X
를 입력하여XML
파일 경로로 이동합니다.XML
파일에서 줄 번호와 불필요한 문자를 제거합니다.xsd test.XML
을 입력하여 test.XML과 동등한XSD 파일
을 만듭니다.- 동일한 파일 경로에
test.XSD
파일이 생성됩니다. XSD /c test.XSD
를 입력하여XML
파일에 해당하는C# 클래스
를 생성합니다.XML
파일의 정확한 스키마가 있는 C# 클래스인test.cs
파일이 생성됩니다.
출력:
//------------------------------------------------------------------------------
// <auto-generated>
// This code was generated by a tool.
// Runtime Version:4.0.30319.42000
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
// </auto-generated>
//------------------------------------------------------------------------------
using System.Xml.Serialization;
//
// This source code was auto-generated by xsd, Version=4.8.3928.0.
//
/// <remarks/>
[System.CodeDom.Compiler.GeneratedCodeAttribute("xsd", "4.8.3928.0")]
[System.SerializableAttribute()]
[System.Diagnostics.DebuggerStepThroughAttribute()]
[System.ComponentModel.DesignerCategoryAttribute("code")]
[System.Xml.Serialization.XmlTypeAttribute(AnonymousType=true)]
[System.Xml.Serialization.XmlRootAttribute(Namespace="", IsNullable=false)]
public partial class Company {
private CompanyEmployee[] itemsField;
/// <remarks/>
[System.Xml.Serialization.XmlElementAttribute("Employee", Form=System.Xml.Schema.XmlSchemaForm.Unqualified)]
public CompanyEmployee[] Items {
get {
return this.itemsField;
}
set {
this.itemsField = value;
}
}
}
/// <remarks/>
[System.CodeDom.Compiler.GeneratedCodeAttribute("xsd", "4.8.3928.0")]
[System.SerializableAttribute()]
[System.Diagnostics.DebuggerStepThroughAttribute()]
[System.ComponentModel.DesignerCategoryAttribute("code")]
[System.Xml.Serialization.XmlTypeAttribute(AnonymousType=true)]
public partial class CompanyEmployee {
private string nameField;
private string ageField;
/// <remarks/>
[System.Xml.Serialization.XmlAttributeAttribute()]
public string name {
get {
return this.nameField;
}
set {
this.nameField = value;
}
}
/// <remarks/>
[System.Xml.Serialization.XmlAttributeAttribute()]
public string age {
get {
return this.ageField;
}
set {
this.ageField = value;
}
}
}
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다