將 JSON 字串轉換為 C# 物件
Fil Zjazel Romaeus Villegas
2023年10月12日
Csharp
Csharp JSON
Csharp Object
-
使用
Newtonsoft.Json
將 JSON 字串轉換為 C# 物件 - 使用 JavaScriptSerializer 將 JSON 字串轉換為 C# 物件
- 將 JSON 字串轉換為 C# 物件程式碼示例
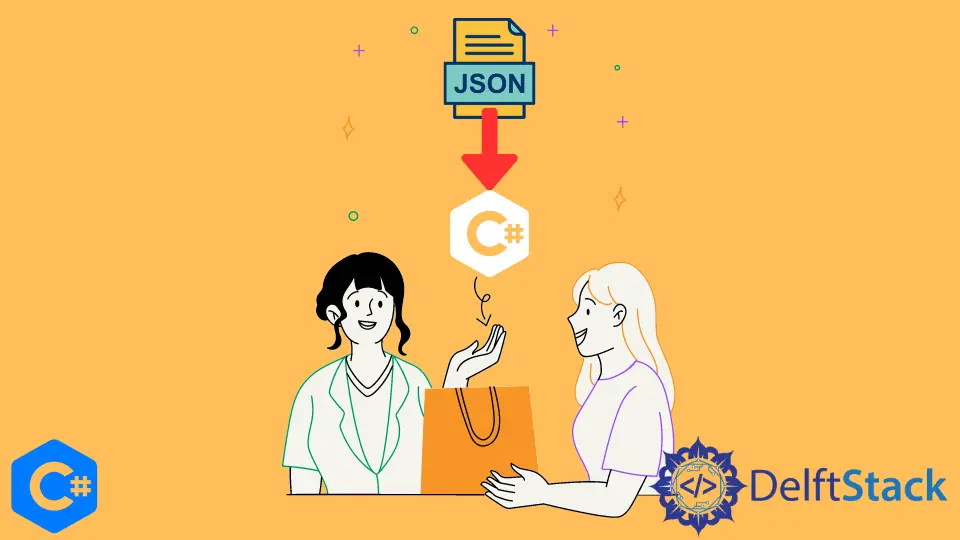
本教程將演示如何使用兩種不同的方法將 JSON 字串轉換為 C# 物件。
第一個使用 Newtonsoft.Json
庫,而第二個使用 JavaScriptSerializer
。這兩種方法相似,但使用的語法略有不同,並且需要不同的引用。
使用 Newtonsoft.Json
將 JSON 字串轉換為 C# 物件
Newtonsoft.Json
庫是 .NET
的常用 JSON 框架。在將軟體包安裝到你的解決方案後,可以使用此庫。你可以使用帶有以下命令的 .NET CLI 來安裝軟體包。
> dotnet add package Newtonsoft.Json --version 13.0.1
JSON 字串可以轉換為任何型別的 C# 物件,只要它是物件型別的有效 JSON 字串即可。這是通過反序列化字串、將其轉換為指定型別 (T) 並提供輸入 JSON 字串來完成的。
JsonConvert.DeserializeObject<T>(json_string);
使用 JavaScriptSerializer 將 JSON 字串轉換為 C# 物件
將 JSON 字串轉換為 C# 物件的舊選項是 JavaScriptSerializer
。雖然它沒有 Newtonsoft.Json
解決方案那麼快,但它仍然可以很好地利用。要使用此方法,你需要在專案中新增對 System.Web.Extensions.dll
的引用。
要新增該引用,請按照以下步驟操作。
-
導航到解決方案資源管理器
-
右鍵單擊引用
-
點選
新增引用
-
單擊左側的
Assemblies
選項卡 -
找到
System.Web.Extensions.dll
檔案並單擊OK
。
JavaScriptSerializer
的工作方式與 Newtonsoft.Json
方法類似,因為它只需要 JSON 字串和要反序列化的型別。
JavaScriptSerializer().Deserialize<T>(json_string)
將 JSON 字串轉換為 C# 物件程式碼示例
下面的程式碼示例演示瞭如何使用 JsonConvert.DeserializeObject<T>
和 JavaScriptSerializer
函式將 JSON 字串轉換為任何型別的 C# 物件。對於此示例,我們將字串轉換為包含整數的列表物件 (List<Int32>
) 以及我們在程式中指定的自定義類。
using Newtonsoft.Json;
using System.Data;
using System;
using System.ComponentModel;
using System.Collections.Generic;
using System.Web.Script.Serialization;
namespace JSON_To_Object_Example {
class Program {
static void Main(string[] args) {
// Declare the JSON Strings
string list_str = "[1, 2, 3, 9, 8, 7]";
string custom_class_str =
"{ \"Name\":\"John Doe\",\"Age\":21,\"Birthday\":\"2000-01-01T00:00:00\"}";
// ----- Newtonsoft Method -----
// Deserialize the string by specifying the object type
List<Int32> list_converted_ns = JsonConvert.DeserializeObject<List<Int32>>(list_str);
// Print the contents of the newly converted List object to the Console.
Console.WriteLine("Converted List - Newtonsoft: " + String.Join(", ", list_converted_ns) +
"\n");
// The same can be done for any type of C# object, including custom classes. Just change the
// specified Type.
Custom_Class class_converted_ns =
JsonConvert.DeserializeObject<Custom_Class>(custom_class_str);
Console.WriteLine("Converted Class - Newtonsoft:");
PrintPropreties(class_converted_ns);
Console.WriteLine("\n\n");
// ----- JavaScriptSerializer Method -----
// Deserialize the string by specifying the object type
List<Int32> list_converted_js = new JavaScriptSerializer().Deserialize<List<Int32>>(list_str);
Console.WriteLine(
"Converted List - JavaScriptSerializer: " + String.Join(", ", list_converted_js) + "\n");
Custom_Class class_converted_js =
new JavaScriptSerializer().Deserialize<Custom_Class>(custom_class_str);
Console.WriteLine("Converted Class - JavaScriptSerializer:");
PrintPropreties(class_converted_js);
Console.ReadLine();
}
public class Custom_Class {
// Custom Class created for the purposes of this tutorial
public string Name { get; set; }
public int Age { get; set; }
public DateTime Birthday { get; set; }
}
public static void PrintPropreties(object obj) {
// Uses the System.Component Model to read each property of a class and print its value
foreach (PropertyDescriptor descriptor in TypeDescriptor.GetProperties(obj)) {
string name = descriptor.Name;
object value = descriptor.GetValue(obj);
Console.WriteLine("{0}: {1}", name, value);
}
}
}
}
此程式碼將輸出由逗號連線的整數列表以及轉換後的自定義類中的所有屬性。你可以觀察到兩種方法都會產生相同的輸出。
輸出:
Converted List - Newtonsoft: 1, 2, 3, 9, 8, 7
Converted Class - Newtonsoft:
Name: John Doe
Age: 21
Birthday: 01/01/2000 12:00:00 am
Converted List - JavaScriptSerializer: 1, 2, 3, 9, 8, 7
Converted Class - JavaScriptSerializer:
Name: John Doe
Age: 21
Birthday: 01/01/2000 12:00:00 am
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe