How to Deserialize JSON With C#
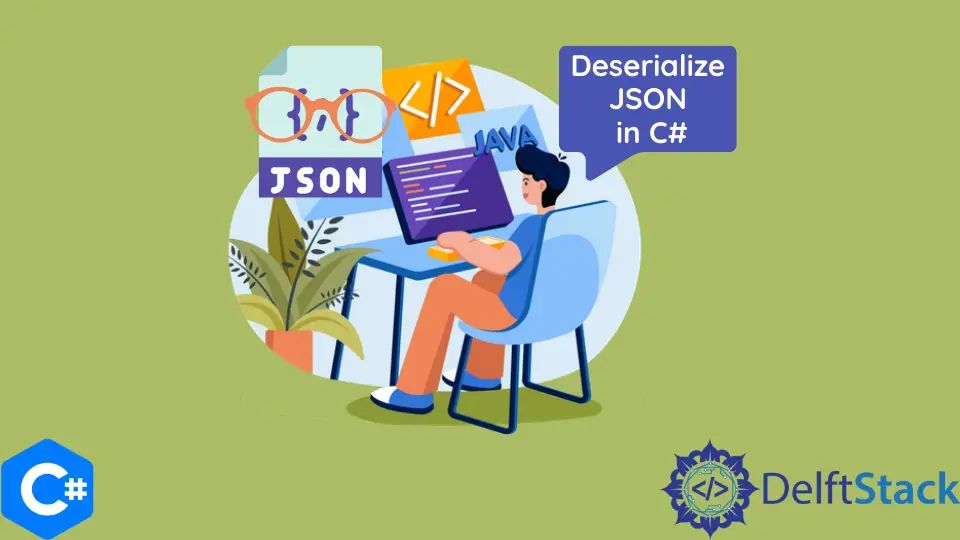
Deserialization in C# refers to retrieving the serialized object so it may load it into memory. Then, it allows the object to be used again. Finally, it brings the object’s previous state back to life by setting its attributes etc.
This tutorial teaches you how to deserialize a JSON object using the C# programming language.
Deserialize JSON With C#
Let’s have an example of deserializing a JSON object using the C# programming language. In the below example, we’ll use students’ data to deserialize JSON with C#. But, first, let’s go through the following example step-by-step.
-
To begin, we’ve to import the following libraries.
using System; using System.Collections.Generic; using System.Web; using System.Web.Script.Serialization; using Newtonsoft.Json; using System.Linq; using System.Text; using System.Threading.Tasks;
-
We’ve to create a
Student
structure which will hold Student’s info, for example,Studentid
andStudentname
as follows.public class Students { public List<studentinfo> MyStudents { get; set; } } public class studentinfo { public string Studentid { get; set; } public string Studentname { get; set; } }
-
Now, we’ll create a JSON list and populate it using random students’ data.
string json = @"{""MyStudents"":[ {""Studentid"":""3227"",""Studentname"":""Muhammad Zeeshan""}, {""Studentid"":""3256"",""Studentname"":""Haseeb Khan""}, {""Studentid"":""3241"",""Studentname"":""Saad Ashrafi""}, {""Studentid"":""3267"",""Studentname"":""Bawa Khan""} ]}";
-
Then, use
.Deserialize()
function to deserialize the list.Students derializedStudent = new System.Web.Script.Serialization.JavaScriptSerializer().Deserialize<Students>(json);
-
Lastly, we’ll use the
foreach
loop to display results on the console.foreach (var item in derializedStudent.MyStudents) { Console.WriteLine("id: {0}, name: {1}", item.Studentid, item.Studentname); }
The complete source code is given below.
Complete Source Code
using System;
using System.Collections.Generic;
using System.Web;
using System.Web.Script.Serialization;
using Newtonsoft.Json;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DeserializeJSONbyZeeshan {
class Program {
static void Main(string[] args) {
string json =
@"{""MyStudents"":[
{""Studentid"":""3227"",""Studentname"":""Muhammad Zeeshan""},
{""Studentid"":""3256"",""Studentname"":""Haseeb Khan""},
{""Studentid"":""3241"",""Studentname"":""Saad Ashrafi""},
{""Studentid"":""3267"",""Studentname"":""Bawa Khan""}
]}";
Students derializedStudent =
new System.Web.Script.Serialization.JavaScriptSerializer().Deserialize<Students>(json);
foreach (var item in derializedStudent.MyStudents) {
Console.WriteLine("id: {0}, name: {1}", item.Studentid, item.Studentname);
}
}
}
}
public class Students {
public List<studentinfo> MyStudents { get; set; }
}
public class studentinfo {
public string Studentid { get; set; }
public string Studentname { get; set; }
}
Output:
id: 3227, name: Muhammad Zeeshan
id: 3256, name: Haseeb Khan
id: 3241, name: Saad Ashrafi
id: 3267, name: Bawa Khan
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn