How to Post JSON to a Server in C#
-
Use
HttpWebRequest
to Post JSON to a Server inC#
-
Use
new HttpClient()
to Post JSON to a Server inC#
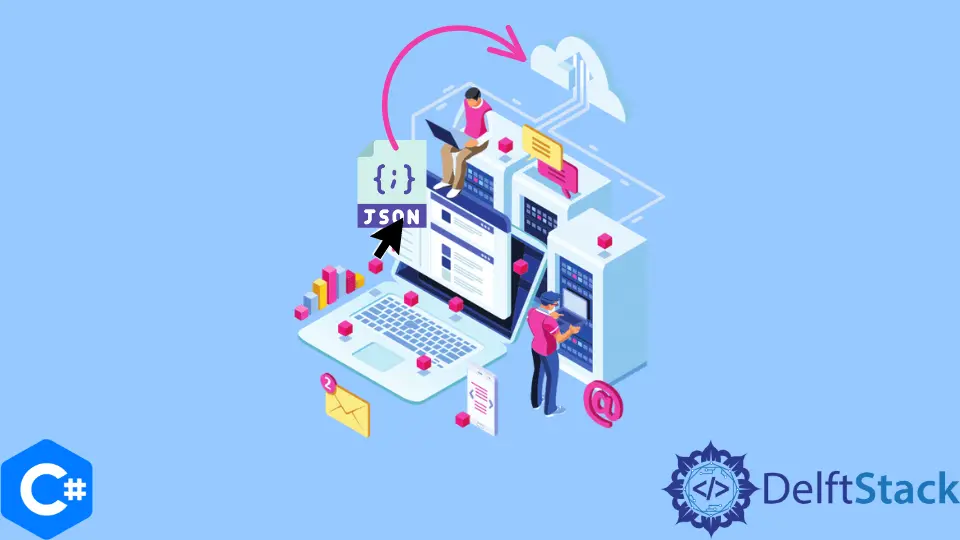
So what is JSON? JSON stands for Javascript Object Notation. It follows a structure similar to having a value mapped to a key encapsulated within square brackets.
Today we will learn how to post a JSON to a server in C#.
Use HttpWebRequest
to Post JSON to a Server in C#
To use the web services, you need to boot up your IDE in .NET ASP, a web framework with C# supported in the backend.
Inside the booted ASP.NET application, you are now required to add a new ASPX page to add a button to post JSON as text and another label to display the posted JSON text.
BUTTON:
LABEL:
The webpage will be displayed as follows:
We will post some JSON to this webpage once the button is clicked. The JSON will be displayed on the textbox below the button.
Now let’s go ahead and inside the button click function, let’s create the request for sending the data and then read the status code, which would tell us if the request was successful or not.
var url = "https://reqres.in/api/users";
var httpRequest = (HttpWebRequest)WebRequest.Create(url);
httpRequest.Method = "POST";
httpRequest.Accept = "application/json";
httpRequest.ContentType = "application/json";
var data =
@"{
""name"": ""morpheus"",
""job"": ""leader"",
""id"": ""264"",
""createdAt"": ""2022-07-09T07:33:55.681Z""
}";
using (var streamWriter = new StreamWriter(httpRequest.GetRequestStream())) {
streamWriter.Write(data);
}
var httpResponse = (HttpWebResponse)httpRequest.GetResponse();
using (var streamReader = new StreamReader(httpResponse.GetResponseStream())) {
var result = streamReader.ReadToEnd();
}
this.jsontext.Text = httpResponse.StatusCode.ToString();
Here we have used the URL, a free URL to post and get data. The image for the status code once the process is shown below:
OUTPUT:
You create the httpRequest
variable for the URL. Then you tend to define the Accept
requirement, which is set to JSON. Then, once the streamWriter
writes the data to the server, the retrieved StatusCode
from the response is put into the text of the jsontext
variable we defined earlier.
In case you don’t want to handcraft JSON, you can use the Serializer
as follows:
string json = new JavaScriptSerializer().Serialize(
new { name = "morpheus", job = "leader", id = "264", createdAt = "2022-07-09T07:33:55.681Z" });
And then, write it as follows:
streamWriter.Write(json);
However, to implement this, you need to include the System.Web.Script.Serialization
namespace.
The full code is shown below:
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Net;
using System.Text;
using System.Web;
using System.Web.Script.Serialization;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace WebApplication2 {
public partial class WebForm1 : System.Web.UI.Page {
protected void Page_Load(object sender, EventArgs e) {}
protected void json_Click(object sender, EventArgs e) {
var url = "https://reqres.in/api/users";
var httpRequest = (HttpWebRequest)WebRequest.Create(url);
httpRequest.Method = "POST";
httpRequest.Accept = "application/json";
httpRequest.ContentType = "application/json";
// HANDCRAFTED JSON
var data =
@"{
""name"": ""morpheus"",
""job"": ""leader"",
""id"": ""264"",
""createdAt"": ""2022-07-09T07:33:55.681Z""
}";
// SERIALIZED JSON
string json =
new JavaScriptSerializer().Serialize(new { name = "morpheus", job = "leader", id = "264",
createdAt = "2022-07-09T07:33:55.681Z" });
using (var streamWriter = new StreamWriter(httpRequest.GetRequestStream())) {
streamWriter.Write(json);
}
var httpResponse = (HttpWebResponse)httpRequest.GetResponse();
using (var streamReader = new StreamReader(httpResponse.GetResponseStream())) {
var result = streamReader.ReadToEnd();
}
this.jsontext.Text = httpResponse.StatusCode.ToString();
}
}
}
Use new HttpClient()
to Post JSON to a Server in C#
We can very simply use the following code to post JSON to the server:
using (var client = new HttpClient()) {
var result = client.PostAsync(url, new StringContent(json, Encoding.UTF8, "application/json"));
}
This uses CLIENT.POSTASYNC()
to issue the POST
request. The full code is shown below:
protected void json_Click(object sender, EventArgs e) {
var url = "https://reqres.in/api/users";
var httpRequest = (HttpWebRequest)WebRequest.Create(url);
httpRequest.Method = "POST";
httpRequest.Accept = "application/json";
httpRequest.ContentType = "application/json";
// HANDCRAFTED JSON
var data =
@"{
""name"": ""morpheus"",
""job"": ""leader"",
""id"": ""264"",
""createdAt"": ""2022-07-09T07:33:55.681Z""
}";
// SERIALIZED JSON
string json =
new JavaScriptSerializer().Serialize(new { name = "morpheus", job = "leader", id = "264",
createdAt = "2022-07-09T07:33:55.681Z" });
using (var client = new HttpClient()) {
var result = client.PostAsync(url, new StringContent(json, Encoding.UTF8, "application/json"));
}
// using (var streamWriter = new StreamWriter(httpRequest.GetRequestStream()))
//{
// streamWriter.Write(json);
// }
var httpResponse = (HttpWebResponse)httpRequest.GetResponse();
using (var streamReader = new StreamReader(httpResponse.GetResponseStream())) {
var result = streamReader.ReadToEnd();
}
this.jsontext.Text = httpResponse.StatusCode.ToString();
}
Replace the function given above and then view the results. The code will work perfectly now.
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHubRelated Article - Csharp JSON
- C# Parse JSON in C#
- How to Convert the Object to a JSON String in C#
- How to Deserialize JSON With C#
- How to Write JSON Into File in C#
- How to Convert JSON String to C# Object