Publicar JSON en un servidor en C#
-
Utilice
HttpWebRequest
para publicar JSON en un servidor enC#
-
Utilice
nuevo HttpClient()
para publicar JSON en un servidor enC#
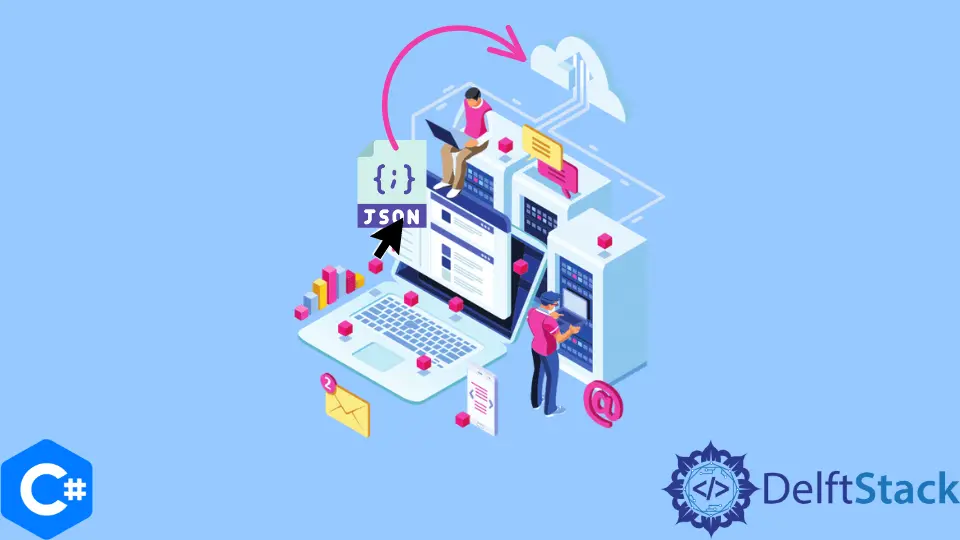
Entonces, ¿qué es JSON? JSON significa Notación de objetos Javascript. Sigue una estructura similar a tener un valor asignado a una clave encapsulada entre corchetes.
Hoy aprenderemos cómo publicar un JSON en un servidor en C#.
Utilice HttpWebRequest
para publicar JSON en un servidor en C#
Para usar los servicios web, debe iniciar su IDE en .NET ASP, un marco web compatible con C# en el backend.
Dentro de la aplicación ASP.NET iniciada, ahora debe agregar una nueva página ASPX para agregar un botón para publicar JSON como texto y otra etiqueta para mostrar el texto JSON publicado.
BOTÓN:
ETIQUETA:
La página web se mostrará de la siguiente manera:
Publicaremos algunos JSON en esta página web una vez que se haga clic en el botón. El JSON se mostrará en el cuadro de texto debajo del botón.
Ahora sigamos adelante y dentro de la función de clic del botón, creemos la solicitud para enviar los datos y luego leamos el código de estado, que nos dirá si la solicitud fue exitosa o no.
var url = "https://reqres.in/api/users";
var httpRequest = (HttpWebRequest)WebRequest.Create(url);
httpRequest.Method = "POST";
httpRequest.Accept = "application/json";
httpRequest.ContentType = "application/json";
var data =
@"{
""name"": ""morpheus"",
""job"": ""leader"",
""id"": ""264"",
""createdAt"": ""2022-07-09T07:33:55.681Z""
}";
using (var streamWriter = new StreamWriter(httpRequest.GetRequestStream())) {
streamWriter.Write(data);
}
var httpResponse = (HttpWebResponse)httpRequest.GetResponse();
using (var streamReader = new StreamReader(httpResponse.GetResponseStream())) {
var result = streamReader.ReadToEnd();
}
this.jsontext.Text = httpResponse.StatusCode.ToString();
Aquí hemos utilizado la URL, una URL gratuita para publicar y obtener datos. La imagen para el código de estado una vez que el proceso se muestra a continuación:
PRODUCCIÓN:
Creas la variable httpRequest
para la URL. Luego, tiende a definir el requisito Aceptar
, que se establece en JSON. Luego, una vez que streamWriter
escribe los datos en el servidor, el StatusCode
recuperado de la respuesta se coloca en el texto de la variable jsontext
que definimos anteriormente.
En caso de que no quiera crear JSON a mano, puede usar el Serializador
de la siguiente manera:
string json = new JavaScriptSerializer().Serialize(
new { name = "morpheus", job = "leader", id = "264", createdAt = "2022-07-09T07:33:55.681Z" });
Y luego, escríbelo de la siguiente manera:
streamWriter.Write(json);
Sin embargo, para implementar esto, debe incluir el espacio de nombres System.Web.Script.Serialization
.
El código completo se muestra a continuación:
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Net;
using System.Text;
using System.Web;
using System.Web.Script.Serialization;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace WebApplication2 {
public partial class WebForm1 : System.Web.UI.Page {
protected void Page_Load(object sender, EventArgs e) {}
protected void json_Click(object sender, EventArgs e) {
var url = "https://reqres.in/api/users";
var httpRequest = (HttpWebRequest)WebRequest.Create(url);
httpRequest.Method = "POST";
httpRequest.Accept = "application/json";
httpRequest.ContentType = "application/json";
// HANDCRAFTED JSON
var data =
@"{
""name"": ""morpheus"",
""job"": ""leader"",
""id"": ""264"",
""createdAt"": ""2022-07-09T07:33:55.681Z""
}";
// SERIALIZED JSON
string json =
new JavaScriptSerializer().Serialize(new { name = "morpheus", job = "leader", id = "264",
createdAt = "2022-07-09T07:33:55.681Z" });
using (var streamWriter = new StreamWriter(httpRequest.GetRequestStream())) {
streamWriter.Write(json);
}
var httpResponse = (HttpWebResponse)httpRequest.GetResponse();
using (var streamReader = new StreamReader(httpResponse.GetResponseStream())) {
var result = streamReader.ReadToEnd();
}
this.jsontext.Text = httpResponse.StatusCode.ToString();
}
}
}
Utilice nuevo HttpClient()
para publicar JSON en un servidor en C#
Simplemente podemos usar el siguiente código para publicar JSON en el servidor:
using (var client = new HttpClient()) {
var result = client.PostAsync(url, new StringContent(json, Encoding.UTF8, "application/json"));
}
Esto utiliza CLIENT.POSTASYNC()
para emitir la solicitud POST
. El código completo se muestra a continuación:
protected void json_Click(object sender, EventArgs e) {
var url = "https://reqres.in/api/users";
var httpRequest = (HttpWebRequest)WebRequest.Create(url);
httpRequest.Method = "POST";
httpRequest.Accept = "application/json";
httpRequest.ContentType = "application/json";
// HANDCRAFTED JSON
var data =
@"{
""name"": ""morpheus"",
""job"": ""leader"",
""id"": ""264"",
""createdAt"": ""2022-07-09T07:33:55.681Z""
}";
// SERIALIZED JSON
string json =
new JavaScriptSerializer().Serialize(new { name = "morpheus", job = "leader", id = "264",
createdAt = "2022-07-09T07:33:55.681Z" });
using (var client = new HttpClient()) {
var result = client.PostAsync(url, new StringContent(json, Encoding.UTF8, "application/json"));
}
// using (var streamWriter = new StreamWriter(httpRequest.GetRequestStream()))
//{
// streamWriter.Write(json);
// }
var httpResponse = (HttpWebResponse)httpRequest.GetResponse();
using (var streamReader = new StreamReader(httpResponse.GetResponseStream())) {
var result = streamReader.ReadToEnd();
}
this.jsontext.Text = httpResponse.StatusCode.ToString();
}
Reemplace la función dada arriba y luego vea los resultados. El código funcionará perfectamente ahora.
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHubArtículo relacionado - Csharp JSON
- C# Parse JSON
- C# Convertir el objeto en una cadena JSON
- Deserializar JSON con C#
- Escribir JSON en un archivo en C#
- Convertir cadena JSON en objeto C#