How to Compare Arrays in C#
-
Use the
SequenceEqual()
Method to Compare Arrays in C# - Manually Compare Matched Elements to Compare Arrays in C#
-
Use the
EqualityComparer.Default.Equals()
Method to Compare Arrays in C# - Conclusion
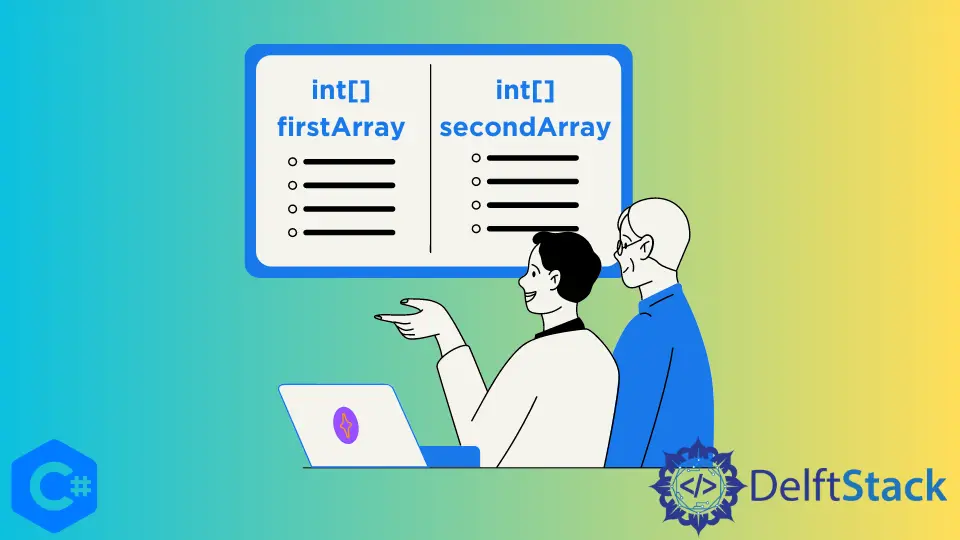
In C#, arrays are fundamental data structures used to store collections of elements. When working with arrays, it is often necessary to compare them to check for equality.
This process involves verifying if the content and order of elements in two or more arrays are identical. In this article, we’ll explore various techniques to compare arrays in C#, including SequenceEqual()
, manual comparison of matched elements, Array.Equals()
, and EqualityComparer.Default.Equals()
.
Use the SequenceEqual()
Method to Compare Arrays in C#
One effective method to determine whether two or more arrays are equal is by using the SequenceEqual()
method provided by the LINQ library. This method allows you to compare the elements of two arrays to check if they are the same, respecting both the content and order.
First, let’s take a look at the syntax of the SequenceEqual()
method before proceeding with the process.
bool result = array1.SequenceEqual(array2);
Here, array1
and array2
are the arrays you want to compare. The SequenceEqual()
method returns a boolean value (true
if the arrays are equal, false
otherwise).
To use the SequenceEqual()
method, you need to import the LINQ library, which contains this function. Add the following using
directive at the beginning of your code:
using System.Linq;
Initialize the arrays you want to compare. Ensure that the arrays are of the same type and have the same length for a meaningful comparison.
For instance:
int[] array1 = { 10, 20, 30, 40, 50 };
int[] array2 = { 10, 20, 30, 40, 50 };
Apply the SequenceEqual()
method to check the equality of the arrays. The result is typically stored in a boolean variable for later use:
bool areEqual = array1.SequenceEqual(array2);
Depending on the result, provide appropriate feedback to the user. Use an if-else
statement to handle the different outcomes:
if (areEqual) {
Console.WriteLine("The arrays are equal.");
} else {
Console.WriteLine("The arrays are not equal.");
}
Here is the complete working code example:
using System;
using System.Linq;
class ArrayComparison {
static void Main() {
int[] array1 = { 10, 20, 30, 40, 50 };
int[] array2 = { 10, 20, 30, 40, 50 };
bool areEqual = array1.SequenceEqual(array2);
if (areEqual) {
Console.WriteLine("The arrays are equal.");
} else {
Console.WriteLine("The arrays are not equal.");
}
}
}
In this example, we declare two arrays (array1
and array2
) with integer values. The SequenceEqual()
method is applied to check if the arrays are equal, and the result is stored in the areEqual
variable.
The if-else
statement is then used to print a corresponding message based on the result of the comparison.
When you run this code with equal arrays, the output will be:
The arrays are equal.
If you modify any element in one of the arrays, the output will change to:
The arrays are not equal.
This demonstrates the simplicity and effectiveness of using the SequenceEqual()
method for array comparisons in C#.
Manually Compare Matched Elements to Compare Arrays in C#
While the SequenceEqual()
method provides a convenient way to compare arrays in C#, there are scenarios where a more granular comparison of matched elements is necessary. This method involves checking each element to ensure that both the content and order of the arrays match.
To start the manual comparison process, create a boolean variable and set its initial value to true
. This variable will be used to track the overall equality of the arrays.
bool isEqual = true;
Initialize the arrays you want to compare, ensuring they are of the same type and length. For example:
int[] array1 = { 10, 20, 30, 40, 50 };
int[] array2 = { 10, 20, 30, 40, 50 };
Verify that the lengths of both arrays are equal. If they are not, set the isEqual
variable to false
.
if (array1.Length == array2.Length) {
// Manual comparison logic will go here
} else {
isEqual = false;
}
Use a for
loop to iterate through the elements of one of the arrays (assuming the lengths are equal). Compare the corresponding elements of both arrays and set the isEqual
variable to false
if any pair of elements does not match.
for (int i = 0; i < array1.Length; i++) {
if (array1[i] != array2[i]) {
isEqual = false;
break;
}
}
Finally, based on the value of the isEqual
variable, display an appropriate message to indicate whether the arrays are equal or not.
if (isEqual) {
Console.WriteLine("The arrays are equal.");
} else {
Console.WriteLine("The arrays are not equal.");
}
Here is a code example incorporating the manual comparison process:
using System;
class ArrayComparison {
static void Main() {
bool isEqual = true;
int[] array1 = { 10, 20, 30, 40, 50 };
int[] array2 = { 10, 20, 30, 40, 50 };
if (array1.Length == array2.Length) {
for (int i = 0; i < array1.Length; i++) {
if (array1[i] != array2[i]) {
isEqual = false;
break;
}
}
} else {
isEqual = false;
}
if (isEqual) {
Console.WriteLine("The arrays are equal.");
} else {
Console.WriteLine("The arrays are not equal.");
}
}
}
In this manual comparison approach, a boolean variable isEqual
is created to track the overall equality of the arrays. The arrays (array1
and array2
) are initialized, and their lengths are checked.
If the lengths are equal, a for
loop is used to iterate through the elements of one array and compare them with the corresponding elements of the other array. If a mismatch is found, the isEqual
variable is set to false
, and the loop breaks.
Finally, based on the value of isEqual
, a message is displayed to indicate whether the arrays are equal or not.
When you execute this code with equal arrays, the output will be:
The arrays are equal.
If you modify any element in one of the arrays, the output will change to:
The arrays are not equal.
Use the EqualityComparer.Default.Equals()
Method to Compare Arrays in C#
In addition to the SequenceEqual()
method and manual comparison, the EqualityComparer.Default.Equals()
method provides another versatile way to compare arrays for equality. This method is particularly useful when working with arrays of custom types or when a specific comparison logic is required.
The syntax for using EqualityComparer.Default.Equals()
is as follows:
bool result = EqualityComparer<T>.Default.Equals(array1, array2);
Here, T
represents the type of elements in the arrays (array1
and array2
). The Equals()
method returns a boolean value (true
if the arrays are equal, false
otherwise).
First, ensure that you have the necessary using
directive at the beginning of your code to access the EqualityComparer
class.
using System.Collections.Generic;
Start by initializing the arrays you want to compare, ensuring that they are of the same type.
int[] first = { 10, 20, 30, 40, 50 };
int[] second = { 10, 20, 30, 40, 50 };
Define a method, such as AreArraysEqual<T>
, that takes two arrays of type T
and uses the EqualityComparer<T>.Default.Equals()
method in a loop to compare corresponding elements.
public static bool AreArraysEqual<T>(T[] first, T[] second) {
EqualityComparer<T> comparer = EqualityComparer<T>.Default;
for (int i = 0; i < first.Length; i++) {
if (!comparer.Equals(first[i], second[i])) {
return false;
}
}
return true;
}
Call the method, passing the arrays you want to compare. In the example code, we use the AreArraysEqual
method to compare the arrays named first
and second
.
if (AreArraysEqual(first, second)) {
Console.WriteLine("The arrays are equal.");
} else {
Console.WriteLine("The arrays are not equal.");
}
Here is the complete working code example:
using System;
using System.Collections.Generic;
public class Example {
public static bool AreArraysEqual<T>(T[] first, T[] second) {
EqualityComparer<T> comparer = EqualityComparer<T>.Default;
for (int i = 0; i < first.Length; i++) {
if (!comparer.Equals(first[i], second[i])) {
return false;
}
}
return true;
}
public static void Main() {
int[] first = { 10, 20, 30, 40, 50 };
int[] second = { 10, 20, 30, 40, 50 };
if (AreArraysEqual(first, second)) {
Console.WriteLine("The arrays are equal.");
} else {
Console.WriteLine("The arrays are not equal.");
}
}
}
In this example, we define a method AreArraysEqual<T>
that takes two arrays (first
and second
) and utilizes EqualityComparer<T>.Default.Equals()
to compare corresponding elements. The method is then invoked in the Main
method to compare two arrays of integers.
When you run this code, the output will be:
The arrays are equal.
This demonstrates the successful comparison of arrays using EqualityComparer.Default.Equals()
. If the arrays were not equal, the output would be The arrays are not equal
.
Conclusion
This comprehensive article has provided you with multiple methods to compare arrays in C#, allowing you to choose the most suitable approach based on your specific requirements. Whether you prefer the simplicity of SequenceEqual()
, the control of manual comparison, or the flexibility of EqualityComparer.Default.Equals()
, you now have the tools to compare arrays efficiently in various scenarios.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn