在 C# 中比较数组
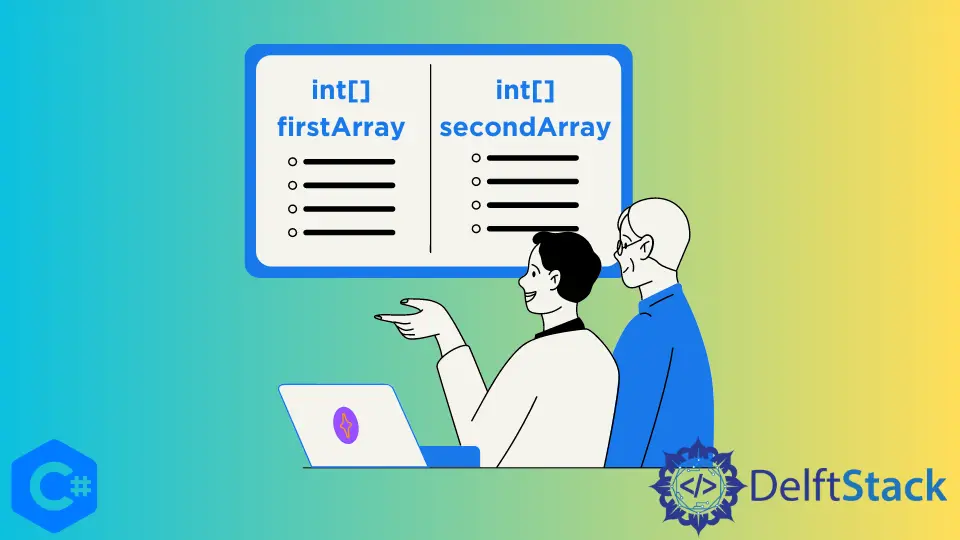
本文将讨论比较两个数组以查看它们是否相等。
我们将讨论和实现来比较数组的两种方法是,
SequenceEqual()
函数;- 比较每个数组中的匹配元素。
如果两个数组具有相同的匹配元素和相同数量的元素,则称它们相等。
在 C# 中使用 SequenceEqual()
函数比较数组
首先,我们必须导入库以访问 SequenceEqual()
函数。
using System.Linq;
创建一个带有 Main()
函数的 ArrayCompare
类。
class ArrayCompare {
static void Main(string[] args) {}
}
将 isEqual
变量声明为布尔数据类型变量。它将根据数组是否相等返回 True 或 False。
Boolean isEqual;
初始化两个名为 firstArray
和 secondArray
的数据类型 int[]
的数组,并为它们分配要比较的整数值。
int[] firstArray = { 10, 20, 30, 40, 40, 50 };
int[] secondArray = { 10, 20, 30, 40, 40, 50 };
我们将使用 SequenceEqual()
方法来比较这两个数组。
isEqual = firstArray.SequenceEqual(secondArray);
现在我们在 isEqual
变量中保存了一个真或假值,我们必须检查它返回的内容。如果返回值为 True,它将执行 if
块,如果返回值为 False,它将执行 else
块。
if (isEqual) {
Console.WriteLine("Both the arrays are equal");
} else {
Console.WriteLine("Both the arrays are not equal");
}
源代码:
using System;
using System.Linq;
class ArrayCompare {
static void Main(string[] args) {
Boolean isEqual;
int[] firstArray = { 10, 20, 30, 40, 40, 50 };
int[] secondArray = { 10, 20, 30, 40, 40, 50 };
isEqual = firstArray.SequenceEqual(secondArray);
if (isEqual) {
Console.WriteLine("Both the arrays are equal.");
} else {
Console.WriteLine("Both the arrays are not equal.");
}
}
}
当两个数组相等时,
输出:
Both the arrays are equal.
假设我们将 firstArray
中的任何元素更改为,
int[] firstArray = { 10, 20, 30, 40, 30, 60 };
输出:
Both the arrays are not equal.
在 C# 中比较匹配的元素来比较数组
我们将创建一个布尔变量并在 Main()
函数中将其值设置为 true。
Boolean isEqual = true;
以与上一个方法相同的方式初始化两个数组。
int[] firstArray = { 10, 20, 30, 40, 40, 50 };
int[] secondArray = { 10, 20, 30, 40, 40, 50 };
我们必须确定两个数组的长度。此外,要相等,所有索引值必须相同。
所以让我们应用一个条件来确保 firstArray
和 secondArray
的长度相等。如果它返回 True,我们必须验证所有索引处的值;否则,isEqual
值将为假。
为了检查数组的所有索引是否相等,我们将使用一个 for
循环,从索引 0 开始并遍历 secondArray
的长度。它将验证所有索引的值,如果它们都不相同,它将设置 isEqual
的值为 False。
if (firstArray.Length == secondArray.Length) {
for (int y = 0; y < secondArray.Length; y++) {
if (secondArray[y] != firstArray[y]) {
isEqual = false;
}
}
} else {
isEqual = false;
}
最后,我们需要输出数组是否相等的消息。
if (isEqual) {
Console.WriteLine("The arrays are equal");
} else {
Console.WriteLine("The arrays are not equal");
}
源代码:
using System;
class ArrayCompare {
static void Main(string[] args) {
Boolean isEqual = true;
int[] firstArray = { 10, 20, 30, 40, 40, 50 };
int[] secondArray = { 10, 20, 30, 40, 40, 50 };
if (firstArray.Length == secondArray.Length) {
for (int y = 0; y < secondArray.Length; y++) {
if (secondArray[y] != firstArray[y]) {
isEqual = false;
}
}
} else {
isEqual = false;
}
if (isEqual) {
Console.WriteLine("Both the arrays are equal.");
} else {
Console.WriteLine("Both the arrays are not equal.");
}
}
}
当两个数组相等时,
输出:
Both the arrays are equal.
如果我们修改任何两个数组中的任何元素,
输出:
Both the arrays are not equal.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn