C#에서 배열 비교
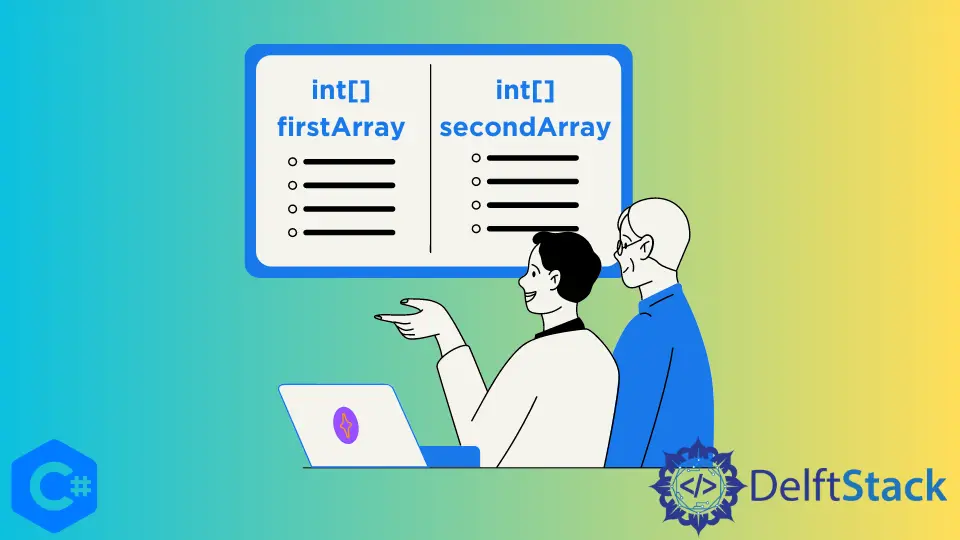
이 기사에서는 두 배열을 비교하여 동일한지 확인하는 방법에 대해 설명합니다.
배열을 비교하기 위해 논의하고 구현할 두 가지 방법은 다음과 같습니다.
SequenceEqual()
함수;- 각 배열에서 일치하는 요소의 비교.
두 배열이 동일한 일치 요소와 동일한 수의 요소를 갖는 경우 동일하다고 합니다.
SequenceEqual()
함수를 사용하여 C#
의 배열 비교
먼저 SequenceEqual()
함수에 액세스하려면 라이브러리를 가져와야 합니다.
using System.Linq;
내부에 Main()
함수를 사용하여 ArrayCompare
클래스를 만듭니다.
class ArrayCompare {
static void Main(string[] args) {}
}
isEqual
변수를 부울 데이터 유형 변수로 선언합니다. 배열이 동일한지 여부에 따라 True 또는 False를 반환합니다.
Boolean isEqual;
firstArray
및 secondArray
라는 데이터 유형 int[]
의 두 배열을 초기화하고 비교할 정수 값을 할당합니다.
int[] firstArray = { 10, 20, 30, 40, 40, 50 };
int[] secondArray = { 10, 20, 30, 40, 40, 50 };
SequenceEqual()
메서드를 사용하여 이 두 배열을 비교할 것입니다.
isEqual = firstArray.SequenceEqual(secondArray);
이제 isEqual
변수에 참 또는 거짓 값이 저장되었으므로 반환되는 값을 확인해야 합니다. 반환된 값이 True이면 if
블록을 실행하고, 반환된 값이 False이면 else
블록을 수행합니다.
if (isEqual) {
Console.WriteLine("Both the arrays are equal");
} else {
Console.WriteLine("Both the arrays are not equal");
}
소스 코드:
using System;
using System.Linq;
class ArrayCompare {
static void Main(string[] args) {
Boolean isEqual;
int[] firstArray = { 10, 20, 30, 40, 40, 50 };
int[] secondArray = { 10, 20, 30, 40, 40, 50 };
isEqual = firstArray.SequenceEqual(secondArray);
if (isEqual) {
Console.WriteLine("Both the arrays are equal.");
} else {
Console.WriteLine("Both the arrays are not equal.");
}
}
}
두 배열이 같을 때,
출력:
Both the arrays are equal.
firstArray
의 요소를 다음과 같이 변경한다고 가정합니다.
int[] firstArray = { 10, 20, 30, 40, 30, 60 };
출력:
Both the arrays are not equal.
C#
에서 일치하는 요소를 비교하여 배열 비교
부울 변수를 만들고 Main()
함수 내에서 값을 true로 설정합니다.
Boolean isEqual = true;
이전 방법과 동일한 방법으로 두 개의 배열을 초기화합니다.
int[] firstArray = { 10, 20, 30, 40, 40, 50 };
int[] secondArray = { 10, 20, 30, 40, 40, 50 };
두 배열의 길이를 결정해야 합니다. 또한 동일하려면 모든 인덱스 값이 동일해야 합니다.
따라서 firstArray
와 secondArray
의 길이가 동일한지 확인하는 조건을 적용해 보겠습니다. True를 반환하면 모든 인덱스에서 값의 유효성을 검사해야 합니다. 그렇지 않으면 isEqual
값은 false가 됩니다.
배열의 모든 인덱스가 동일한지 확인하기 위해 인덱스 0에서 시작하여 secondArray
의 길이에 걸쳐 반복하는 for
루프를 사용합니다. 모든 인덱스의 값을 확인하고 모두 같지 않으면 isEqual
값을 False로 설정합니다.
if (firstArray.Length == secondArray.Length) {
for (int y = 0; y < secondArray.Length; y++) {
if (secondArray[y] != firstArray[y]) {
isEqual = false;
}
}
} else {
isEqual = false;
}
마지막으로 배열이 동일한지 여부를 메시지로 출력해야 합니다.
if (isEqual) {
Console.WriteLine("The arrays are equal");
} else {
Console.WriteLine("The arrays are not equal");
}
소스 코드:
using System;
class ArrayCompare {
static void Main(string[] args) {
Boolean isEqual = true;
int[] firstArray = { 10, 20, 30, 40, 40, 50 };
int[] secondArray = { 10, 20, 30, 40, 40, 50 };
if (firstArray.Length == secondArray.Length) {
for (int y = 0; y < secondArray.Length; y++) {
if (secondArray[y] != firstArray[y]) {
isEqual = false;
}
}
} else {
isEqual = false;
}
if (isEqual) {
Console.WriteLine("Both the arrays are equal.");
} else {
Console.WriteLine("Both the arrays are not equal.");
}
}
}
두 배열이 같을 때,
출력:
Both the arrays are equal.
두 배열의 요소를 수정하면
출력:
Both the arrays are not equal.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn