How to Check if a String Is Null or Empty in C#
-
Check if a String Is Empty or Null in
C#
-
Check if a String Is Null in
C#
-
Check if a String Variable Is Empty in
C#
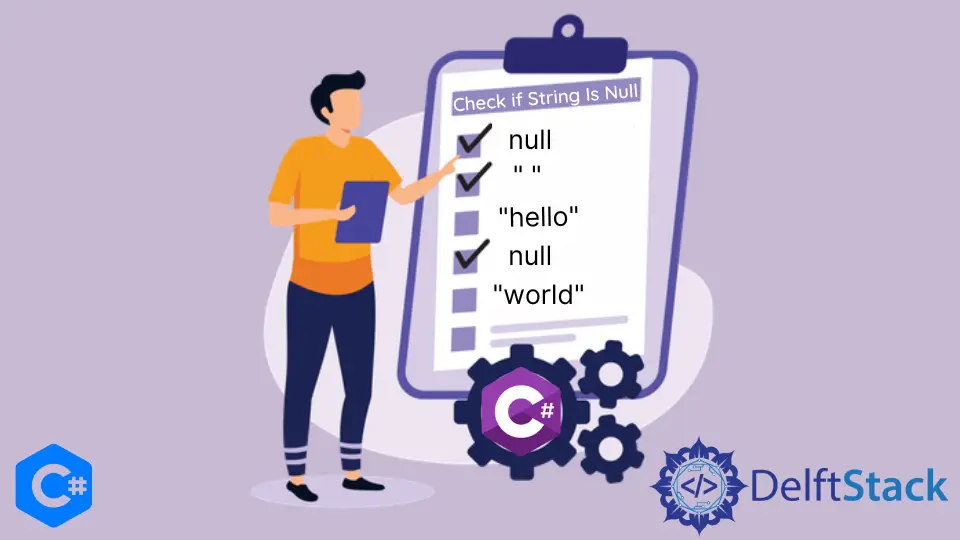
This tutorial will discuss the methods of checking if a string is empty or null in C#.
Check if a String Is Empty or Null in C#
If we want to check for a string that has either null
value or ""
value in it, we can use the string.IsNullOrEmpty()
method in C#. The string.IsNullOrEmpty()
method has a boolean return type. It returns true
if the string is either empty or null. See the following code example.
using System;
namespace check_string {
class Program {
static void Main(string[] args) {
string s = null;
if (string.IsNullOrEmpty(s)) {
Console.WriteLine("String is either null or empty");
}
}
}
}
Output:
String is either null or empty
In the above code, we assigned the null
value to the string variable s
and check whether it is empty or null with the string.IsNullOrEmpty()
method in C#.
Check if a String Is Null in C#
In the above section, we check for the null
value and ""
value combined. If we want to separately check whether a string is null
or not, we can use the ==
comparison operator. See the following code example.
using System;
namespace check_string {
class Program {
static void Main(string[] args) {
string s = null;
if (s == null) {
Console.WriteLine("String is null");
}
}
}
}
Output:
String is null
In the above code, we check whether the string variable s
is null
or not with the ==
comparison operator in C#.
Check if a String Variable Is Empty in C#
As in the previous example, we can also separately check whether a string is empty or not with the string.Empty
field in C#. The string.Empty
field represents an empty in C#. See the following code example.
using System;
namespace check_string {
class Program {
static void Main(string[] args) {
string s = "";
if (s == string.Empty) {
Console.WriteLine("String is empty");
}
}
}
}
Output:
String is empty
In the above code, we check whether the string is empty with the string.Empty
field in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn