C# で文字列が Null または空かどうかをチェックする
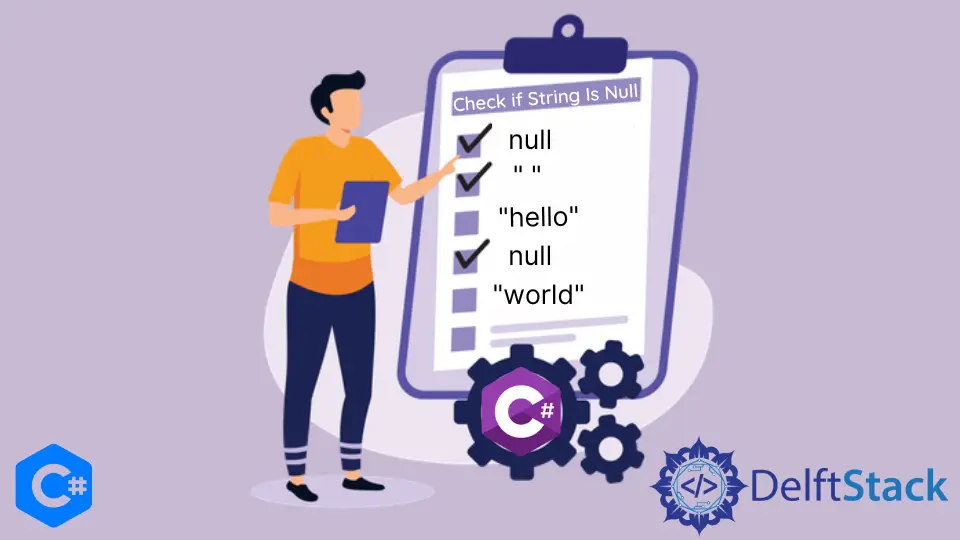
このチュートリアルでは、C# で文字列が空か null かを確認する方法について説明します。
C# で文字列が空か Null かを確認する
null
値または""
値のいずれかを含む文字列を確認する場合は、C# で string.IsNullOrEmpty()
メソッドを使用できます。string.IsNullOrEmpty()
メソッドにはブール値の戻り型があります。文字列が空または null の場合、true
を返します。次のコード例を参照してください。
using System;
namespace check_string {
class Program {
static void Main(string[] args) {
string s = null;
if (string.IsNullOrEmpty(s)) {
Console.WriteLine("String is either null or empty");
}
}
}
}
出力:
String is either null or empty
上記のコードでは、文字列変数 s
に null
値を割り当て、C# の string.IsNullOrEmpty()
メソッドを使用して、それが空か null かを確認しました。
C# で文字列が Null かどうかを確認する
上記のセクションでは、null
値と""
値の組み合わせを確認します。文字列が null
であるかどうかを個別に確認したい場合は、==
比較演算子を使用できます。次のコード例を参照してください。
using System;
namespace check_string {
class Program {
static void Main(string[] args) {
string s = null;
if (s == null) {
Console.WriteLine("String is null");
}
}
}
}
出力:
String is null
上記のコードでは、C# の ==
比較演算子を使用して、文字列変数 s
が null
であるかどうかを確認します。
文字列変数が C# で空かどうかを確認する
前の例のように、C# の string.Empty
フィールドを使用して、文字列が空であるかどうかを個別に確認することもできます。string.Empty
フィールドは、C# の空を表します。次のコード例を参照してください。
using System;
namespace check_string {
class Program {
static void Main(string[] args) {
string s = "";
if (s == string.Empty) {
Console.WriteLine("String is empty");
}
}
}
}
出力:
String is empty
上記のコードでは、C# の string.Empty
フィールドで文字列が空かどうかを確認します。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn