How to Read Int From a File in C++
-
How to Read Int From a File in C++ Using the
while
Loop and the>>
Operator -
How to Read Int From a File in C++ Using the
while
Loop and the>>
Operator With thepush_back
Method -
How to Read Int From a File in C++ Using the
while
Loop andgetline
Withstringstream
-
Avoid Using the
eof()
Method With thewhile
Loop to Read Int From a Text File in C++ - Conclusion
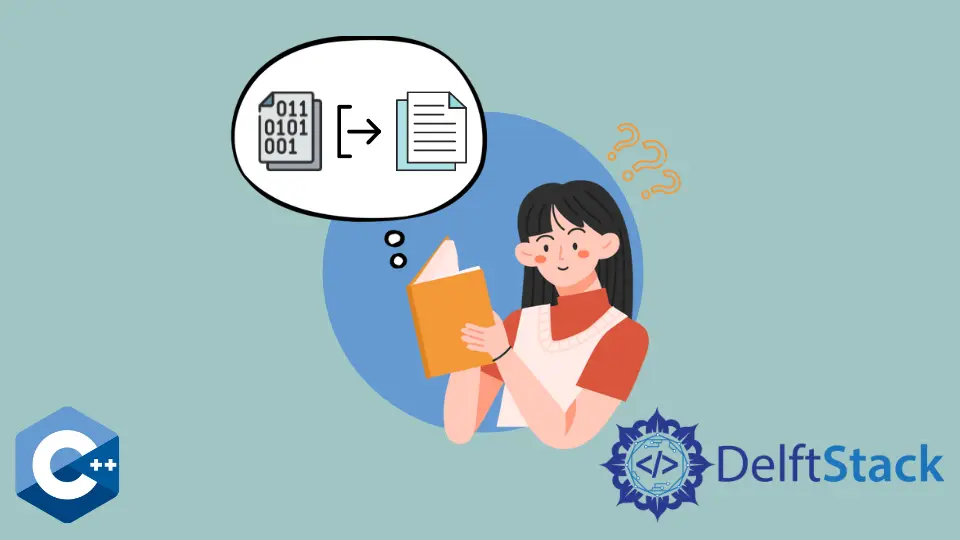
Reading integer data from a file is a common task in C++ programming, often encountered when dealing with data stored in external files. Whether working with configuration files, input datasets, or other types of data sources, the ability to efficiently read integer data from a file is essential for many applications.
In this article, we’ll explore various methods for accomplishing this task in C++, discussing their advantages, limitations, and best practices.
Before diving into reading integer data from a file, ensure you have a basic understanding of file handling in C++. You should be familiar with concepts like file streams (ifstream
), opening and closing files, error handling, and loops.
First, you need a file containing integer data. Create a text file (let’s name it input.txt
) and populate it with integers separated by spaces, tabs, or newlines, like so:
123 178 1289 39 90 89 267 909 23 154 377 34 974 322
How to Read Int From a File in C++ Using the while
Loop and the >>
Operator
One straightforward approach to reading integer data from a text file is by utilizing a while
loop in conjunction with the >>
operator. This method reads integer data sequentially from the file until the end of the file (EOF) is reached.
The while
loop continuously reads data from the file using the >>
operator. This operator reads data from the file stream and stores it in the specified variable.
The loop continues iterating until it reaches the end of the file, at which point the EOF condition is met, and the loop terminates. Each integer read from the file is then processed as needed.
Example: Reading Integer Data Using the >>
Operator
Now, let’s write the C++ code to read integers from the file using a while
loop and the >>
operator.
#include <fstream>
#include <iostream>
using namespace std;
int main() {
string filename("input.txt");
int data;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file >> data) {
// Process each integer
cout << data << "; ";
}
input_file.close();
return EXIT_SUCCESS;
}
First, we include the necessary header files, fstream
for file handling and iostream
for input/output operations. Then, we declare two variables: filename
, a string to hold the name of the input file, and number
, an integer to store each integer read from the file.
Moving forward, we open the file stream using ifstream
and associate it with the input file specified by filename
. Subsequently, we perform a check to ensure that the file was opened successfully.
If not, we print an error message indicating the failure to open the text file and exit the program with a failure status.
Now, we enter a while
loop where the core reading operation takes place. Inside the loop, we utilize the >>
operator to sequentially read integers from the file stream into the number
variable.
while (input_file >> number) {
cout << number << "; ";
}
This operation continues until the end of the file is reached, as the loop condition depends on the success of the input operation. With each iteration of the loop, a new integer is read from the file and stored in number
.
Within the loop, we have the opportunity to process each integer as needed. In this particular example, we simply print each integer to the console, followed by a semicolon and a space, indicating the separation between integers.
This printing occurs within the loop body and repeats for each integer read from the file.
Once all integers have been read and processed, we reach the end of the loop. At this point, we have successfully read all integer data from the file.
Code Output:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
This output demonstrates the successful reading and processing of integer data from the file input.txt
, with each integer separated by a semicolon and a space.
How to Read Int From a File in C++ Using the while
Loop and the >>
Operator With the push_back
Method
Another approach to read integer data from a text file involves utilizing the while
loop in combination with the >>
input operator, coupled with the push_back
method to store the integers in a vector dynamically. This method allows for the flexible storage and manipulation of integer data read from the file.
As discussed above, the while
loop iterates through the file until the end of the file (EOF) is reached. During each iteration, the >>
operator reads an integer from the file stream and stores it in a variable.
However, instead of directly processing the integer, as seen in the previous method, here, we utilize the push_back
method to add each integer to a dynamically resizing vector. This vector serves as a container for storing the integers read from the file.
By using push_back
, we can accommodate an arbitrary number of integers from the file without needing to know the file’s size beforehand.
Example: Reading Integer Data Using the push_back
Method
Let’s modify the previous code to read integer data using the push_back
method of a vector.
#include <fstream>
#include <iostream>
#include <vector>
using namespace std;
int main() {
string filename("input.txt");
vector<int> numbers;
int number;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file >> number) {
numbers.push_back(number);
}
input_file.close();
for (const auto &num : numbers) {
cout << num << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
The code begins by including necessary header files for file handling, input/output operations, and using vectors. We declare a string
variable filename
to hold the input.txt
file and a vector<int>
named numbers
to store the integers read from the file dynamically.
Next, we open the file stream using ifstream
and associate it with the input file specified by filename
.
We perform a check to ensure that the file was opened successfully. If not, we print an error message and exit the program with a failure status.
Within the while
loop, integers are read from the file using the >>
operator, similar to the previous method. However, instead of processing each integer immediately, we use the push_back
method to add it to the numbers
vector.
while (input_file >> number) {
numbers.push_back(number);
}
This allows us to dynamically store each integer as it is read from the file.
Once the entire file has been traversed and all integers have been read and stored in the vector, we close the file stream using the close()
method to release associated resources.
Finally, we output the integers stored in the vector by iterating over it using a range-based for
loop. Each integer is printed to the console, followed by a semicolon and a space, similar to the previous method.
Code Output:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
This output demonstrates the successful reading and storage of integer data from the file input.txt
into a dynamically resizing vector. Each integer value is separated by a semicolon and a space, reflecting the contents of the input file.
How to Read Int From a File in C++ Using the while
Loop and getline
With stringstream
An alternative method to read integer data from a text file involves using the while
loop in combination with the getline
function to extract each line from the file as a string and then utilizing stringstream
to parse the integers from each line. This method provides flexibility when integers are separated by delimiters like spaces or newlines.
The getline
function reads each line from the file until the end of the file (EOF) is reached. Within each iteration of the while
loop, a line from the file is stored as a string.
We then use stringstream
to parse this string and extract integers. By treating the string as a stream and using the >>
operator, we can extract integers one by one from the string.
This process continues until all integers from the current line have been extracted. The loop proceeds to the next line in the file until the EOF condition is met.
Example: Reading Integer Data Using stringstream
Let’s adapt our previous code to read integer data using getline
and stringstream
. Take a look at the following code:
#include <fstream>
#include <iostream>
#include <sstream>
#include <string>
using namespace std;
int main() {
string filename = "input.txt";
ifstream inputFile(filename);
string line;
int count = 0;
if (!inputFile.is_open()) {
cerr << "Error: Unable to open file " << filename << endl;
return EXIT_FAILURE;
}
while (getline(inputFile, line)) {
stringstream ss(line);
int num;
while (ss >> num) {
cout << num << "; ";
count++;
}
}
cout << "\nTotal numbers read: " << count << endl;
inputFile.close();
return EXIT_SUCCESS;
}
In the above program, we begin by including necessary header files for file handling, input/output operations, and string manipulation. We declare a string variable, filename
, to hold the name of the input file and an input file stream, inputFile
, to read from the file.
Additionally, we define the variable line
to store each line read from the file.
We then open the input file stream using ifstream
and check if the file was opened successfully. If not, we print an error message and exit the program with a failure status.
Within the while
loop, we use the getline
function to read each line from the text file into the variable line
. For each line, we create a stringstream
object ss
to treat the line as a stream.
We then use the >>
operator to extract integers from the stringstream
and store them in the variable num
. This process continues until all integers from the current line have been extracted. Additionally, a count variable named count
is incremented each time a number is successfully read.
Once all integers from the current line have been processed, the while
loop moves on to the next line in the file, this process continues until the end of the file is reached.
Finally, we close the input file stream using the close()
method to release associated resources.
Code Output:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
Total numbers read: 14
This output demonstrates the successful reading and processing of integer data from the file input.txt
using the while
loop and getline
with stringstream
. Each integer is separated by a semicolon and a space, reflecting the contents of the input file.
Avoid Using the eof()
Method With the while
Loop to Read Int From a Text File in C++
When it comes to reading data from files in C++, one might be tempted to use the eof()
method in a while
loop condition to extract integer data until the end of the file is reached. However, relying solely on eof()
for loop termination can lead to unexpected behavior and errors in the code.
The eof()
member function in C++ is used to check if the end-of-file (EOF) indicator has been set on the input stream. It returns true
if the end of the file has been reached and false
otherwise.
This method is commonly misused in loop conditions for file reading operations, especially when combined with while
loops.
Let’s delve into why it’s advisable to avoid using eof()
in this context.
-
End-of-File Condition Detection: The
eof()
method only detects the end-of-file condition after attempting to read past the end of the file. This means that in a loop condition, the loop might execute one extra iteration before detecting the end of the file, leading to unexpected behavior. -
Input Stream State: Using
eof()
alone does not consider the state of the input stream. Even after reaching the end of the file, the input stream may still be in a valid state for reading.Therefore, relying solely on
eof()
can cause premature termination of the loop, missing the last valid data. -
Infinite Loop Risk: Misusing
eof()
in a loop condition can result in an infinite loop. This typically occurs when the loop reads data inside its body, and theeof()
check is done only at the beginning of each iteration.If the end-of-file condition is not reached but a read operation fails, the loop continues indefinitely.
In order to avoid these pitfalls, it’s recommended to use alternative methods for file reading, such as using the >>
operator in conjunction with a while
loop or getline
with stringstream
, both of which were discussed earlier. These methods provide more reliable and predictable behavior for reading integer data from a file.
Conclusion
Reading integer data from a text file in C++ is a fundamental task with various methods to achieve it. Throughout this article, we’ve explored several approaches, each offering its advantages and considerations.
Firstly, we discussed the straightforward method of using a while
loop in conjunction with the >>
operator to read integers directly from the file stream. This method is simple and efficient, making it suitable for many scenarios where integers are separated by whitespace or newlines.
Next, we explored an alternative approach using getline
with stringstream
, which provides more flexibility when dealing with integers separated by custom delimiters or appearing on multiple lines. This method allows for more robust parsing of integer data from the file.
Additionally, we highlighted the importance of avoiding the eof()
method in a while
loop for integer data extraction due to its potential for causing unexpected behavior and errors.
In practice, the choice of method depends on factors such as the format of the input file, the complexity of the data, and performance considerations. Select the most appropriate method for your specific requirements.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook