How to Remove a File in C++
- File Handling in C++
-
Use the
remove()
Function From thecstdio
Library to Delete a File in C++ -
Use the
remove()
Function From thefilesystem
Library to Delete a File in C++ - Conclusion
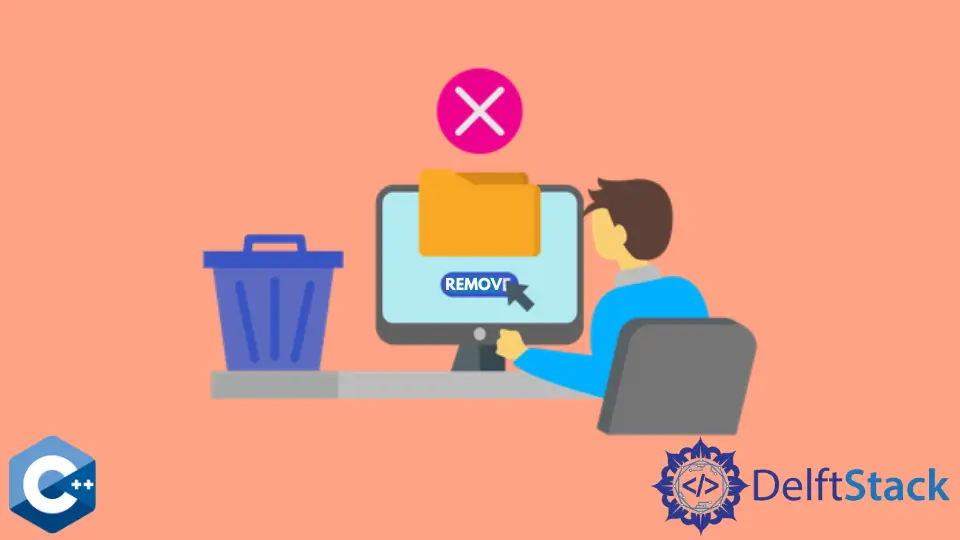
In C++, effective file handling is necessary for tasks such as data storage and retrieval. This article explores the fundamental concepts of file handling in C++, particularly in deleting files using the remove()
function from the cstdio
library.
We will also discuss a more modern approach using the C++17-introduced filesystem
library to provide a versatile file manipulation approach.
File Handling in C++
File handling in programming refers to the process of reading data from and writing data to external files. This is an essential aspect of many programs, as it allows data to be stored persistently and retrieved later.
Common operations include creating, opening, reading, writing, and closing files. File handling has evolved in modern times as more and more efficient libraries are being introduced to handle such operations.
C++ offers robust features and functions for file handling. With different libraries, we can create and modify files in C++.
The overall functionality is also straightforward to use.
The standard fstream
library provides loads of basic functions and objects. We can implement file handling in C++ using the fstream
library, which provides classes like ifstream
, ofstream
, and fstream
for reading and writing files.
As an upgrade to the traditional fstream
library, C++ 17 introduced the filesystem
library, which is part of the Standard Template Library (STL) to provide a comprehensive set of tools for working with file systems. The filesystem
library simplifies common file and directory operations, making it easier to navigate and manipulate file systems.
In this tutorial, we will discuss how to delete a file in C++.
Use the remove()
Function From the cstdio
Library to Delete a File in C++
In C++, the cstdio
library is part of the C Standard Library and provides facilities for performing input and output operations using the C Standard Input and Output functions. The cstdio
library is commonly used in C++ for file handling and other low-level I/O operations.
This library provides the remove()
function that allows us to delete files. It returns a value of zero if the file exists and is deleted; otherwise, it returns a non-zero integer.
Syntax:
int res = remove(file_name);
Here, we need to specify the filename and path (file_name
) within the function, and the file will be deleted permanently. In order to handle errors, the perror()
function can be used to print a descriptive error message.
Note that when we use this function, the deleted file can no longer be recovered from the Recycle Bin.
See the following example code.
#include <cstdio>
#include <iostream>
using namespace std;
int main() {
char file_name[] = "DelftStack.txt";
int res = remove(file_name);
if (res != 0) {
cout << "No deletion";
} else {
cout << "File deleted";
}
return 0;
}
Output:
In the above example, we delete an already created text file using the remove()
function. We store the filename and path in the file_name
character array.
We then pass this array to the remove()
function, and consequently, the file is deleted.
File deletion is a critical operation, and it’s important to confirm that the file deletion was successful. One should always check the return value of the remove()
function and handle errors appropriately.
The remove()
function is a straightforward function to delete a file, but it doesn’t provide any detailed error details. For more detailed error handling information, one might consider using a platform-specific file system API or any other additional available libraries.
Remember to put the correct path of the file to be deleted. There are many instances where the C++ compiler may interpret the path as incorrect due to a misuse of the \
character while specifying the pathname.
The \
can be interpreted as an escape character. Note the use of the \\
in the above example to avoid this error.
Use the remove()
Function From the filesystem
Library to Delete a File in C++
In C++, the filesystem
library provides a more modern and convenient way to handle files and directories.
We can use the remove()
function from the filesystem
library to delete the directories and files. This function returns True
if the file is successfully deleted; otherwise, it returns False
.
We can also use this function to return how many files or directories are deleted.
Syntax:
std::uintmax_t remove(const std::filesystem::path& p);
The above syntax returns the number of files deleted after specifying the path within the function while using a filesystem
object.
Let us understand this with an example.
#include <cstdint>
#include <filesystem>
#include <iostream>
namespace fsob = std::filesystem;
int main() {
fsob::path tmp{std::filesystem::temp_directory_path()};
std::filesystem::create_directories(tmp / "delftstack.txt");
std::uintmax_t n{fsob::remove(tmp / "delftstack.txt")};
if (n = 0) {
std::cout << "No Deletion";
} else {
std::cout << "File deleted";
}
}
Output:
In the above example, we create a filesystem
object, fsob
, and use it to create a temporary file using the tmp_directory_path()
function. The temporary file is termed delftstack.txt
.
Then, we delete this file using the remove()
function and return the count of files deleted. Since the file deletion is successful, the function returns 1
, and we display File Deleted
in the output console.
Using the filesystem
library is considered a more modern and safer approach compared to older C-style file-handling functions. It provides a higher level of abstraction and better error-handling mechanisms.
Conclusion
We have discussed the methods of how to delete a file in C++. We also discussed file handling in C++ and its basics.
To delete a file, we introduced the remove()
functions from the cstdio
and filesystem
libraries. The remove()
function from the cstdio
library takes a single argument, which is the name of the file to be removed.
We demonstrated an example where we take an already created file from the system and delete it using this function. One should remember that this deleted file cannot be retrieved from the recycle bin.
We also discussed the use of the filesystem
library to delete a file in C++. In our example above, we created a temporary file and proceeded to delete the same using the remove()
method.
This library is considered a better and safer option than the previous remove()
function of the cstdio
library, especially in terms of error handling.
Note that the filesystem
library is part of the C++17 standard, so make sure the compiler supports C++17 or above. The filesystem
library is part of the C++ Standard Library and is considered a better choice for many file-related operations.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn