How to Get File Extension in C++
- Method 1: Using String Manipulation
- Method 2: Using File System Library (C++17)
- Method 3: Handling Multiple File Extensions
- Conclusion
- FAQ
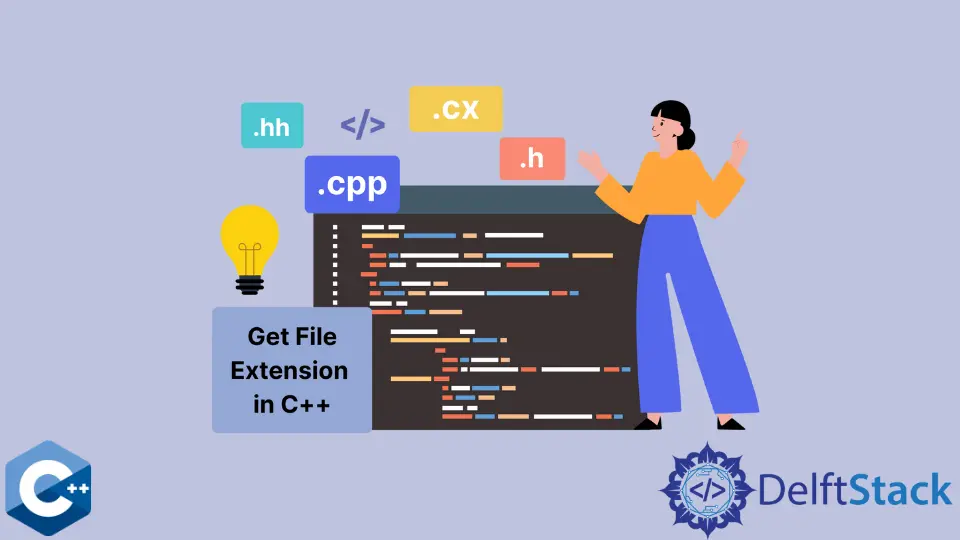
When working with files in C++, understanding file extensions is crucial. A file extension is the last part of a filename, typically following a dot, that indicates the file’s format and type of data it contains. Whether you’re developing software, managing files, or just curious about file handling, knowing how to retrieve and manipulate file extensions can enhance your programming skills.
In this article, we will explore various methods to get file extensions in C++, focusing on simple and effective techniques. We’ll also provide clear examples to help you grasp these concepts easily. Let’s dive into the world of file handling in C++!
Method 1: Using String Manipulation
One of the simplest ways to retrieve a file extension in C++ is through string manipulation. The idea is to find the last occurrence of the dot in the filename and extract the substring that follows it. Here’s how you can do that:
#include <iostream>
#include <string>
std::string getFileExtension(const std::string& filename) {
size_t dotPosition = filename.find_last_of('.');
if (dotPosition != std::string::npos) {
return filename.substr(dotPosition + 1);
}
return ""; // No extension found
}
int main() {
std::string filename = "example.txt";
std::cout << "File extension: " << getFileExtension(filename) << std::endl;
return 0;
}
Output:
File extension: txt
In this code, we define a function getFileExtension
that takes a filename as input. We use find_last_of
to locate the last dot in the filename. If a dot is found, substr
extracts the extension starting from the position right after the dot. If no dot is found, the function returns an empty string, indicating that there is no file extension.
Method 2: Using File System Library (C++17)
If you’re using C++17 or later, the standard library provides a more robust way to handle file paths, including getting file extensions. The filesystem
library simplifies this process significantly. Here’s how you can use it:
#include <iostream>
#include <filesystem>
std::string getFileExtension(const std::string& filename) {
std::filesystem::path filePath(filename);
return filePath.extension().string();
}
int main() {
std::string filename = "example.docx";
std::cout << "File extension: " << getFileExtension(filename) << std::endl;
return 0;
}
Output:
File extension: .docx
In this example, we utilize the filesystem
library to create a path
object from the filename. The extension()
method then retrieves the file extension, including the dot. This approach is cleaner and more reliable, especially when dealing with complex file paths.
Method 3: Handling Multiple File Extensions
Sometimes, files may have multiple extensions, such as “archive.tar.gz”. In such cases, you might want to retrieve all extensions or just the last one. Here’s how you can handle this scenario:
#include <iostream>
#include <string>
#include <vector>
#include <sstream>
std::vector<std::string> getFileExtensions(const std::string& filename) {
std::vector<std::string> extensions;
std::string::size_type dotPosition = filename.find_first_of('.');
while (dotPosition != std::string::npos) {
std::string extension = filename.substr(dotPosition + 1);
extensions.push_back(extension);
dotPosition = filename.find_first_of('.', dotPosition + 1);
}
return extensions;
}
int main() {
std::string filename = "archive.tar.gz";
std::vector<std::string> extensions = getFileExtensions(filename);
std::cout << "File extensions: ";
for (const auto& ext : extensions) {
std::cout << ext << " ";
}
std::cout << std::endl;
return 0;
}
Output:
File extensions: tar gz
In this code, we define a function getFileExtensions
that retrieves all extensions from a filename. We use a loop to find each dot and extract the substring that follows it. This way, we can handle files with multiple extensions effectively, storing them in a vector for easy access.
Conclusion
Getting file extensions in C++ is a straightforward task, whether you’re using basic string manipulation or the more advanced filesystem library introduced in C++17. Understanding how to handle file extensions can improve your file management capabilities and enhance your programming skills. By mastering these methods, you can efficiently work with various file types and formats in your C++ projects. Remember to choose the method that best fits your needs and the version of C++ you are using. Happy coding!
FAQ
-
How do I get the file extension of a file in C++?
You can retrieve the file extension by using string manipulation or the filesystem library in C++. The latter is more efficient if you’re using C++17 or later. -
What if a filename has no extension?
If a filename has no extension, you can return an empty string or handle it accordingly in your code. -
Can I get multiple file extensions from a filename?
Yes, you can extract multiple extensions by searching for all occurrences of the dot in the filename and retrieving the substrings that follow. -
Is the filesystem library available in older versions of C++?
No, the filesystem library was introduced in C++17. If you are using an older version, you will need to rely on string manipulation techniques. -
What should I do if my filename contains special characters?
Ensure your code handles special characters correctly. Using the filesystem library can help manage complex filenames more effectively.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook