Multiple Code Files in C++
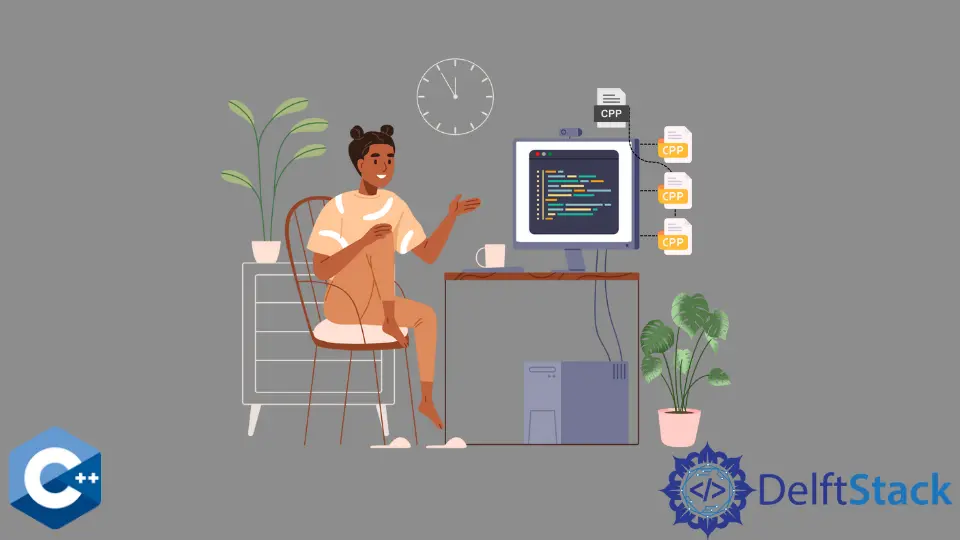
This tutorial is about using multiple files in your single c++ program. Writing all your code in a single file becomes cumbersome for bigger and lengthy projects, so we will learn how to separate files in a project and make it more manageable and easy to refactor.
You have written all of your code in a single file up to this point in your programming career. It had everything: the main function, prototypes, system functions, extra functions, everything!
This is not the best method for assembling a (large) programming project. You’ll learn to break up your programming project into multiple files to make it more organized, secure, portable, modifiable, and faster to recompile.
There are many reasons behind this, but we can’t go into detail because of time and information overload.
Use Multiple Code Files in a C++ Program
We will start with an example file that has everything in one file, and then we will separate that file.
Example code:
#include <iostream>
using namespace std;
void helloFunc();
int main() {
helloFunc();
return 0;
}
void helloFunc() { cout << "Hello World" << endl; }
In this code snippet, you can see that we have first declared the helloFunc()
function and then defined it at a later stage in the program. Then, we have written the main
function.
This can be done in three files. We will declare the function in the header file and define it in the cpp
file.
Example code (hello.h
):
#include <iostream>
using namespace std;
void helloFunc();
Example code (hello.cpp
):
#include "hello.h"
void helloFunc() { cout << "Hello World" << endl; }
Example code (main.cpp
):
#include "hello.h"
int main() {
helloFunc();
return 0;
}
Let us start with the header file hello.h
. Header files contain the definitions of everything like function definitions, any struct
or class
definitions, or any constants definitions.
This .h
extension tells the compiler that this file is not to be compiled; it is just like a text file and is readable by anyone. This implies that the header file is a documenting file.
If any programmer wants to use some functions in the future, they only need to check the prototype of the functions and don’t need to go into detail about function definitions. Lastly, the template code should also be in the header file.
Now comes the cpp
file, which contains the function definition declared in the header file. The CPP file tells the compiler that this file is to be compiled and converted into a binary file.
Your code’s integrity is protected, and no one else can modify it without your permission. This means that this separation of code also ensures the security of your code files.
Another reason behind this technique is portability; for example, you have written a binary search code that can be used later in many other programs. If you have these separate files for functions, you can easily use such functions in any other project.
At last, the main
file contains only the main
function and includes the header file at the top. This file contains only the main
function, which only calls all the functions and nothing else.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn