如何用 C++ 从文件中读取整数
-
在 C++ 中使用
while
循环和>>
操作符从文件中读取 Int -
使用
while
循环和>>
运算符结合push_back
方法从文件中读取 Int 值 -
不要使用
while
循环和eof()
方法从文件中读取 Int
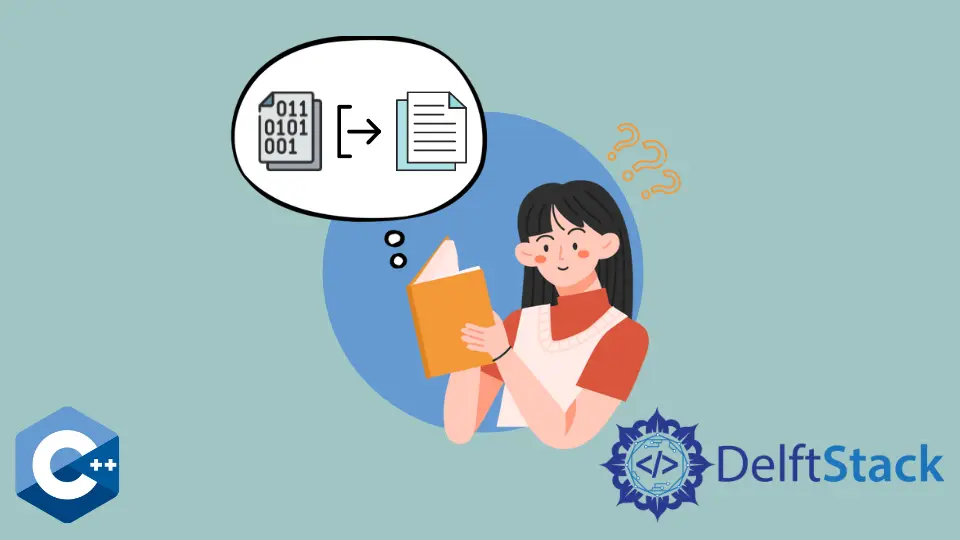
本文将解释几种 C++ 方法,如何从文件中读取 int
数据。
在下面的示例程序中,我们假设一个文本文件命名为 input.txt
,其中包含多行以空格分隔的整数。需要注意的是,每个示例代码都会检查这个文件名是否与实际的文件流相关联,并在出错时打印相应的失败信息。
input.txt
的内容:
123 178 1289 39 90 89 267 909 23 154 377 34 974 322
在 C++ 中使用 while
循环和 >>
操作符从文件中读取 Int
这个方法使用 while
循环进行迭代处理,直到到达 EOF(文件末尾),并将每个整数存储在数字变量中。然后,我们可以在循环体中把每个数字打印到控制台。
#include <fstream>
#include <iostream>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
int main() {
string filename("input.txt");
int number;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file >> number) {
cout << number << "; ";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
输出:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
使用 while
循环和 >>
运算符结合 push_back
方法从文件中读取 Int 值
作为另一种选择,我们可以从文件中获取每个整数,像前面的例子一样将其存储在数字变量中,然后在每次迭代时将它们推到 int
向量中。请注意,这个方案包括另一个 for
循环,以模仿更实际的系统,在这个系统中,需要对存储的矢量编号的元素进行操作。
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<int> numbers;
int number;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file >> number) {
numbers.push_back(number);
}
for (const auto &i : numbers) {
cout << i << "; ";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
输出:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
不要使用 while
循环和 eof()
方法从文件中读取 Int
可以考虑使用 eof()
成员函数作为 while
循环条件来解决同样的问题。遗憾的是,这可能会导致一次额外的迭代。由于函数 eof()
只有在 eofbit
被设置时才返回 true,所以可能会导致未初始化的迭代被修改。下面的代码示例演示了这种情况。
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<int> numbers;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (!input_file.eof()) {
int tmp;
input_file >> tmp;
numbers.push_back(tmp);
}
for (const auto &i : numbers) {
cout << i << "; ";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
输出:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu