C++의 파일에서 Int를 읽는 방법
-
while
루프 및>>
연산자를 사용하여 C++의 파일에서 Int 읽기 -
while
루프와>>
연산자를push_back
메서드와 결합하여 파일에서 Int를 읽습니다 -
while
루프 및eof()
메서드를 사용하여 파일에서 Int를 읽지 마십시오
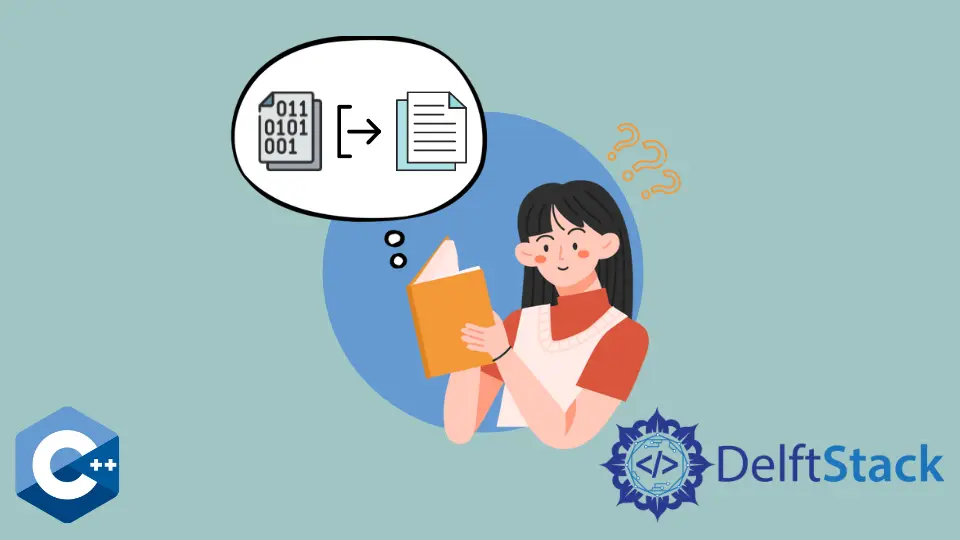
이 기사에서는 파일에서int
데이터를 읽는 방법에 대한 여러 C++ 메서드를 설명합니다.
다음 예제 프로그램에서 텍스트 파일 이름이 input.txt
라고 가정합니다.이 파일에는 여러 줄에 공백으로 구분 된 정수가 포함됩니다. 각 샘플 코드는이 파일 이름이 실제 파일 스트림과 연결되어 있는지 확인하고 오류 발생시 해당 오류 메시지를 인쇄합니다.
input.txt
의 내용 :
123 178 1289 39 90 89 267 909 23 154 377 34 974 322
while
루프 및>>
연산자를 사용하여 C++의 파일에서 Int 읽기
이 메서드는while
루프를 사용하여 EOF (파일 끝)에 도달 할 때까지 프로세스를 반복하고 각 정수를 숫자 변수에 저장합니다. 그런 다음 루프 본문에서 콘솔에 각 번호를 인쇄 할 수 있습니다.
#include <fstream>
#include <iostream>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
int main() {
string filename("input.txt");
int number;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file >> number) {
cout << number << "; ";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
출력:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
while
루프와>>
연산자를push_back
메서드와 결합하여 파일에서 Int를 읽습니다
또 다른 대안으로, 파일에서 각 정수를 가져 와서 이전 예제와 같이 숫자 변수에 저장 한 다음 반복 할 때마다 int
벡터로 푸시 할 수 있습니다. 이 시나리오에는 저장된 벡터 번호의 요소를 조작해야하는보다 실용적인 시스템을 모방하는 또 다른 for
루프가 포함되어 있습니다.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<int> numbers;
int number;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file >> number) {
numbers.push_back(number);
}
for (const auto &i : numbers) {
cout << i << "; ";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
출력:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
while
루프 및eof()
메서드를 사용하여 파일에서 Int를 읽지 마십시오
동일한 문제에 접근하기 위해while
루프 조건으로eof()
멤버 함수를 사용하는 것을 고려할 수 있습니다. 불행히도 추가 반복이 발생할 수 있습니다. 함수eof()
는eofbit
가 설정된 경우에만 true
를 반환하므로 초기화되지 않은 항목이 수정되는 반복으로 이어질 수 있습니다. 이 시나리오는 아래 코드 샘플로 설명됩니다.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<int> numbers;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (!input_file.eof()) {
int tmp;
input_file >> tmp;
numbers.push_back(tmp);
}
for (const auto &i : numbers) {
cout << i << "; ";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
출력:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook