如何用 C++ 從檔案中讀取整數
-
在 C++ 中使用
while
迴圈和>>
操作符從檔案中讀取 Int -
使用
while
迴圈和>>
運算子結合push_back
方法從檔案中讀取 Int 值 -
不要使用
while
迴圈和eof()
方法從檔案中讀取 Int
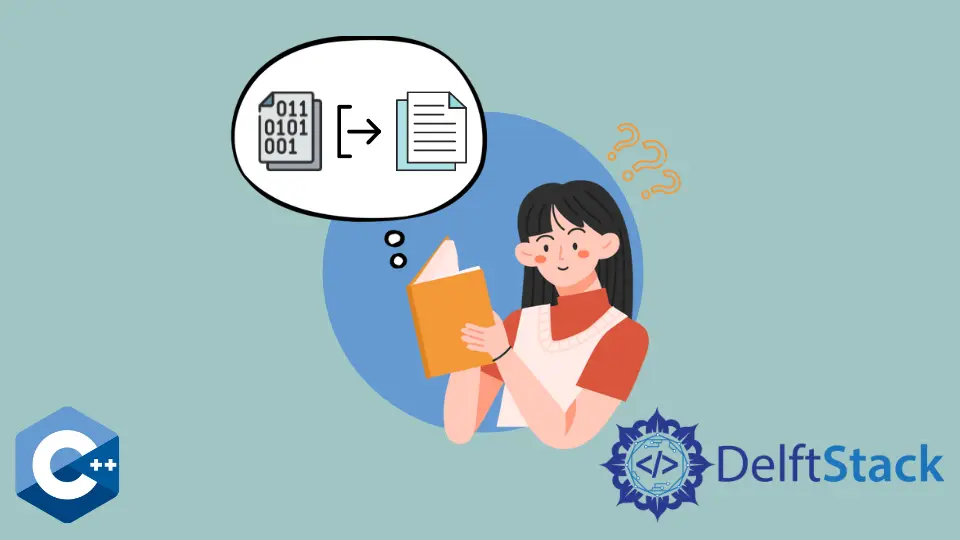
本文將解釋幾種 C++ 方法,如何從檔案中讀取 int
資料。
在下面的示例程式中,我們假設一個文字檔案命名為 input.txt
,其中包含多行以空格分隔的整數。需要注意的是,每個示例程式碼都會檢查這個檔名是否與實際的檔案流相關聯,並在出錯時列印相應的失敗資訊。
input.txt
的內容:
123 178 1289 39 90 89 267 909 23 154 377 34 974 322
在 C++ 中使用 while
迴圈和 >>
操作符從檔案中讀取 Int
這個方法使用 while
迴圈進行迭代處理,直到到達 EOF(檔案末尾),並將每個整數儲存在數字變數中。然後,我們可以在迴圈體中把每個數字列印到控制檯。
#include <fstream>
#include <iostream>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
int main() {
string filename("input.txt");
int number;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file >> number) {
cout << number << "; ";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
輸出:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
使用 while
迴圈和 >>
運算子結合 push_back
方法從檔案中讀取 Int 值
作為另一種選擇,我們可以從檔案中獲取每個整數,像前面的例子一樣將其儲存在數字變數中,然後在每次迭代時將它們推到 int
向量中。請注意,這個方案包括另一個 for
迴圈,以模仿更實際的系統,在這個系統中,需要對儲存的向量編號的元素進行操作。
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<int> numbers;
int number;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file >> number) {
numbers.push_back(number);
}
for (const auto &i : numbers) {
cout << i << "; ";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
輸出:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
不要使用 while
迴圈和 eof()
方法從檔案中讀取 Int
可以考慮使用 eof()
成員函式作為 while
迴圈條件來解決同樣的問題。遺憾的是,這可能會導致一次額外的迭代。由於函式 eof()
只有在 eofbit
被設定時才返回 true,所以可能會導致未初始化的迭代被修改。下面的程式碼示例演示了這種情況。
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<int> numbers;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (!input_file.eof()) {
int tmp;
input_file >> tmp;
numbers.push_back(tmp);
}
for (const auto &i : numbers) {
cout << i << "; ";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
輸出:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu