在 C++ 中寫入檔案
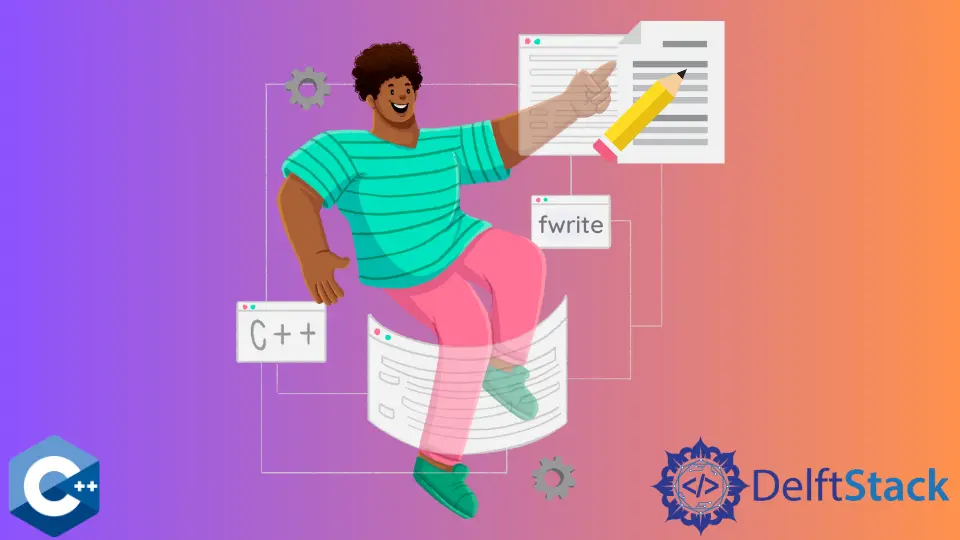
本文將為大家講解幾種在 C++ 中寫入檔案的方法。
使用 fstream
和 <<
操作符寫到檔案上
C++ 中的檔案 I/O
是用 fstream
類來處理的,它提供了多種內建的流操作和定位方法。一旦宣告瞭 fstream
物件,我們就可以呼叫 open
函式,傳遞檔案的名稱和開啟檔案的模式作為引數。
當向檔案寫入時,我們指定 std::ios_base::out
模式,對應的是開啟的寫入狀態。接下來,我們檢查檔案是否已經與流物件成功關聯,也就是在 if
條件下呼叫 is_open
方法。此時,檔案就可以使用 <<
操作符接受輸入,並根據需要處理輸出。
注意,else
塊可以用於來進行錯誤記錄,或者我們可以實現錯誤處理例程,以達到更好的程式執行容錯的目的。
#include <fstream>
#include <iostream>
using std::cout;
using std::endl;
using std::fstream;
using std::ofstream;
using std::string;
int main() {
string filename("tmp.txt");
fstream file_out;
file_out.open(filename, std::ios_base::out);
if (!file_out.is_open()) {
cout << "failed to open " << filename << '\n';
} else {
file_out << "Some random text to write." << endl;
cout << "Done Writing!" << endl;
}
return EXIT_SUCCESS;
}
輸出:
Done Writing!
使用 fstream
和 write
函式寫入檔案
另一種寫入檔案的方法是內建的 write
函式,可以從 fstream
物件中呼叫。write
函式接受一個字串指標和儲存在該地址的資料大小。結果,給定的資料被插入到與呼叫流物件相關聯的檔案中。
在下面的程式碼示例中,我們通過列印 open
函式呼叫的狀態來演示最小的錯誤報告,但在現實世界的應用中,它需要更強大的異常處理,這在手冊頁中有所記載。
#include <fstream>
#include <iostream>
using std::cout;
using std::endl;
using std::fstream;
using std::ofstream;
using std::string;
int main() {
string text("Some huge text to write to\n");
string filename2("tmp2.txt");
fstream outfile;
outfile.open(filename2, std::ios_base::out);
if (!outfile.is_open()) {
cout << "failed to open " << filename2 << '\n';
} else {
outfile.write(text.data(), text.size());
cout << "Done Writing!" << endl;
}
return EXIT_SUCCESS;
}
輸出:
Done Writing!
使用 fwrite
函式寫入檔案
另外,我們還可以使用 C 式檔案 I/O
中的 fwrite
函式來寫入檔案。fwrite
從 void
指標中獲取資料,程式設計師負責傳遞該地址儲存的元素以及每個元素的大小。該函式需要檔案流的型別為 FILE*
,可以通過呼叫 fopen
函式獲得。
注意,fwrite
操作成功後,返回寫入檔案的元素數。
#include <fstream>
#include <iostream>
using std::cout;
using std::endl;
using std::ofstream;
using std::string;
int main() {
string text("Some huge text to write to\n");
string filename3("tmp3.txt");
FILE *o_file = fopen(filename3.c_str(), "w+");
if (o_file) {
fwrite(text.c_str(), 1, text.size(), o_file);
cout << "Done Writing!" << endl;
}
return EXIT_SUCCESS;
}
輸出:
Done Writing!
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu