在 C++ 中写入文件
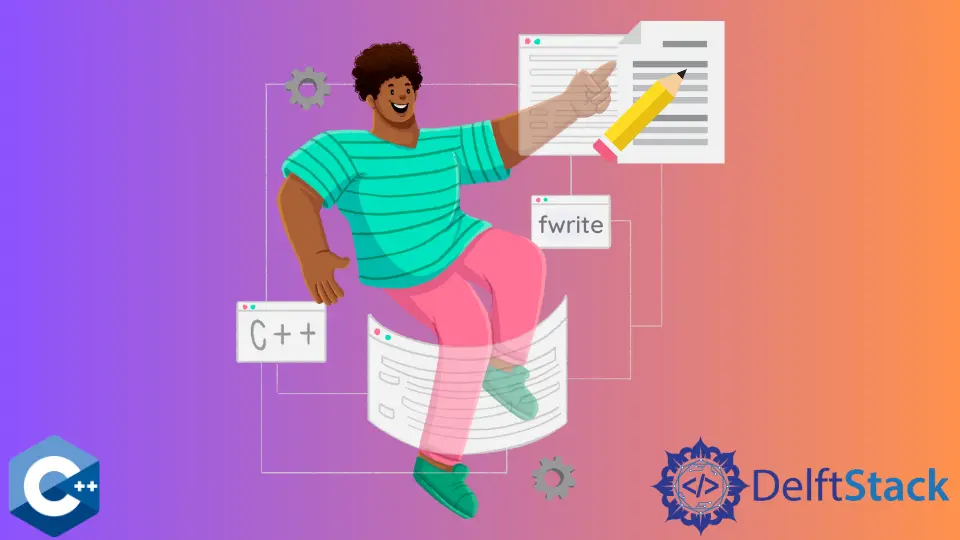
本文将为大家讲解几种在 C++ 中写入文件的方法。
使用 fstream
和 <<
操作符写到文件上
C++ 中的文件 I/O
是用 fstream
类来处理的,它提供了多种内置的流操作和定位方法。一旦声明了 fstream
对象,我们就可以调用 open
函数,传递文件的名称和打开文件的模式作为参数。
当向文件写入时,我们指定 std::ios_base::out
模式,对应的是打开的写入状态。接下来,我们检查文件是否已经与流对象成功关联,也就是在 if
条件下调用 is_open
方法。此时,文件就可以使用 <<
操作符接受输入,并根据需要处理输出。
注意,else
块可以用于来进行错误记录,或者我们可以实现错误处理例程,以达到更好的程序执行容错的目的。
#include <fstream>
#include <iostream>
using std::cout;
using std::endl;
using std::fstream;
using std::ofstream;
using std::string;
int main() {
string filename("tmp.txt");
fstream file_out;
file_out.open(filename, std::ios_base::out);
if (!file_out.is_open()) {
cout << "failed to open " << filename << '\n';
} else {
file_out << "Some random text to write." << endl;
cout << "Done Writing!" << endl;
}
return EXIT_SUCCESS;
}
输出:
Done Writing!
使用 fstream
和 write
函数写入文件
另一种写入文件的方法是内置的 write
函数,可以从 fstream
对象中调用。write
函数接受一个字符串指针和存储在该地址的数据大小。结果,给定的数据被插入到与调用流对象相关联的文件中。
在下面的代码示例中,我们通过打印 open
函数调用的状态来演示最小的错误报告,但在现实世界的应用中,它需要更强大的异常处理,这在手册页中有所记载。
#include <fstream>
#include <iostream>
using std::cout;
using std::endl;
using std::fstream;
using std::ofstream;
using std::string;
int main() {
string text("Some huge text to write to\n");
string filename2("tmp2.txt");
fstream outfile;
outfile.open(filename2, std::ios_base::out);
if (!outfile.is_open()) {
cout << "failed to open " << filename2 << '\n';
} else {
outfile.write(text.data(), text.size());
cout << "Done Writing!" << endl;
}
return EXIT_SUCCESS;
}
输出:
Done Writing!
使用 fwrite
函数写入文件
另外,我们还可以使用 C 式文件 I/O
中的 fwrite
函数来写入文件。fwrite
从 void
指针中获取数据,程序员负责传递该地址存储的元素以及每个元素的大小。该函数需要文件流的类型为 FILE*
,可以通过调用 fopen
函数获得。
注意,fwrite
操作成功后,返回写入文件的元素数。
#include <fstream>
#include <iostream>
using std::cout;
using std::endl;
using std::ofstream;
using std::string;
int main() {
string text("Some huge text to write to\n");
string filename3("tmp3.txt");
FILE *o_file = fopen(filename3.c_str(), "w+");
if (o_file) {
fwrite(text.c_str(), 1, text.size(), o_file);
cout << "Done Writing!" << endl;
}
return EXIT_SUCCESS;
}
输出:
Done Writing!
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu