Leggi Int da un file in C++
-
Usa il cicli
while
e l’operatore>>
per leggere Int dal file in C++ -
Usa
while
Loop e>>
Operatore combinato con il metodopush_back
per leggere Int da file -
Non usare il cicli
while
e il metodoeof()
per leggere Int dal file
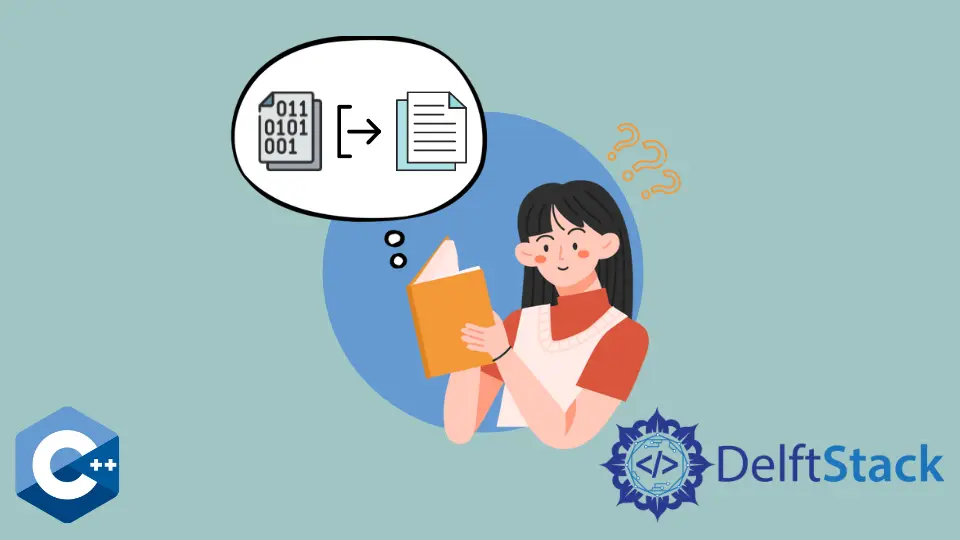
Questo articolo spiegherà diversi metodi C++ su come leggere i dati int
da un file.
Nei seguenti programmi di esempio, assumiamo che un file di testo sia denominato input.txt
, che contiene numeri interi separati da spazi su più linee. Si noti che ogni codice di esempio controlla se questo nome file viene associato al flusso di file effettivo e stampa il messaggio di errore corrispondente in caso di errore.
- Contenuto di
input.txt
:
123 178 1289 39 90 89 267 909 23 154 377 34 974 322
Usa il cicli while
e l’operatore >>
per leggere Int dal file in C++
Questo metodo utilizza il cicli while
per iterare il processo fino a raggiungere l’EOF (fine del file) e memorizzare ogni numero intero nella variabile numerica. Quindi, possiamo stampare ogni numero sulla console nel corpo del bucle.
#include <fstream>
#include <iostream>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
int main() {
string filename("input.txt");
int number;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file >> number) {
cout << number << "; ";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
Produzione:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
Usa while
Loop e >>
Operatore combinato con il metodo push_back
per leggere Int da file
In alternativa, possiamo ottenere ogni numero intero dal file, memorizzarlo nella variabile numerica come nell’esempio precedente, e poi inserirli nel vettore int
in ogni iterazione. Si noti che questo scenario include un altro cicli for
per imitare il sistema più pratico in cui gli elementi dei numeri vettoriali memorizzati devono essere manipolati.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<int> numbers;
int number;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file >> number) {
numbers.push_back(number);
}
for (const auto &i : numbers) {
cout << i << "; ";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
Produzione:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
Non usare il cicli while
e il metodo eof()
per leggere Int dal file
Si potrebbe considerare l’utilizzo della funzione membro eof()
come condizione del cicli while
per affrontare lo stesso problema. Sfortunatamente, ciò potrebbe portare a un’iterazione extra. Poiché la funzione eof()
restituisce true solo se eofbit
è impostato, potrebbe portare all’iterazione in cui viene modificato non inizializzato. Questo scenario è dimostrato dall’esempio di codice riportato di seguito:
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<int> numbers;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (!input_file.eof()) {
int tmp;
input_file >> tmp;
numbers.push_back(tmp);
}
for (const auto &i : numbers) {
cout << i << "; ";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
Produzione:
123; 178; 1289; 39; 90; 89; 267; 909; 23; 154; 377; 34; 974; 322;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook