Leggi File Char da Char in C++
-
Usa il metodo
ifstream
eget
per leggere il file Char da Char -
Usa la funzione
getc
per leggere il file Char per Char -
Usa la funzione
fgetc
per leggere il file Char per Char
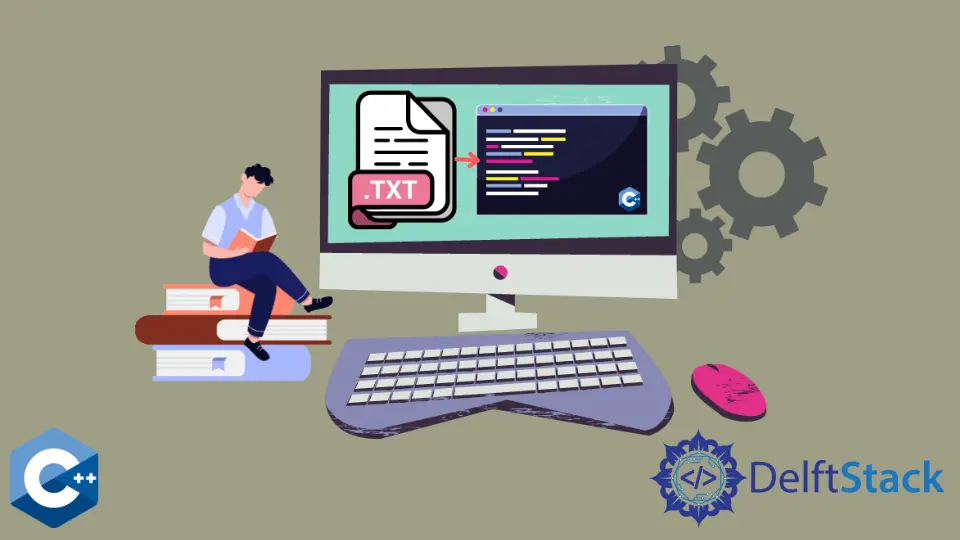
Questo articolo spiegherà diversi metodi su come leggere un file di testo char
con char
in C++.
Usa il metodo ifstream
e get
per leggere il file Char da Char
Il modo più comune per gestire l’I/O di file in C++ è usare std::ifstream
. All’inizio, un oggetto ifstream
viene inizializzato con l’argomento del nome del file che deve essere aperto. Si noti che, l’istruzione if
verifica se l’apertura di un file è riuscita. Successivamente, usiamo la funzione incorporata get
per recuperare il testo all’interno del file char per char e push_back
nel contenitore vector
. Infine, inviamo elementi vettoriali alla console a scopo dimostrativo.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<char> bytes;
char byte = 0;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file.get(byte)) {
bytes.push_back(byte);
}
for (const auto &i : bytes) {
cout << i << "-";
}
cout << endl;
input_file.close();
return EXIT_SUCCESS;
}
Usa la funzione getc
per leggere il file Char per Char
Un altro metodo per leggere un file carattere per carattere consiste nell’usare la funzione getc
, che accetta il flusso FILE*
come argomento e legge il carattere successivo se disponibile. Successivamente, il flusso del file di input dovrebbe essere iterato fino a raggiungere l’ultimo char
, e questo viene implementato con la funzione feof
, controllando se è stata raggiunta la fine del file. Tieni presente che è sempre consigliabile chiudere i flussi di file aperti una volta che non sono più necessari.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<char> bytes;
FILE* input_file = fopen(filename.c_str(), "r");
if (input_file == nullptr) {
return EXIT_FAILURE;
}
unsigned char character = 0;
while (!feof(input_file)) {
character = getc(input_file);
cout << character << "-";
}
cout << endl;
fclose(input_file);
return EXIT_SUCCESS;
}
Usa la funzione fgetc
per leggere il file Char per Char
fgetc
è un metodo alternativo alla funzione precedente, che implementa esattamente la stessa funzionalità di getc
. In questo caso, il valore restituito da fgetc
viene utilizzato nell’istruzione if
poiché restituisce EOF
se viene raggiunta la fine del flusso di file. Come consigliato, prima dell’uscita dal programma, chiudiamo lo stream con una chiamata fclose
.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<char> bytes;
FILE* input_file = fopen(filename.c_str(), "r");
if (input_file == nullptr) {
return EXIT_FAILURE;
}
int c;
while ((c = fgetc(input_file)) != EOF) {
putchar(c);
cout << "-";
}
cout << endl;
fclose(input_file);
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook