How to Read File Char by Char in C++
- Method 1: Using fstream
- Method 2: Using std::getline with a String
-
Method 3: Use the
getc
Function - Method 4: Using C-style FILE* and fgetc
- Conclusion
- FAQ
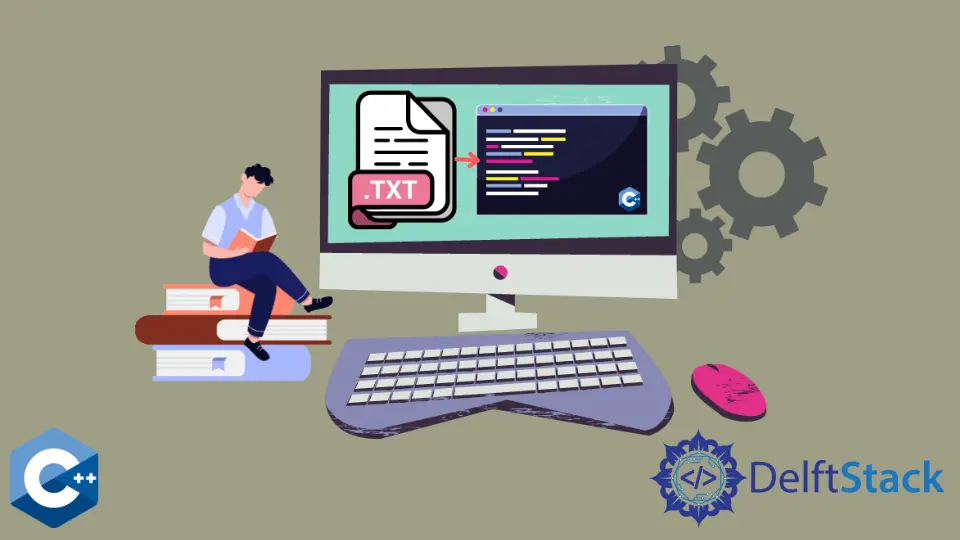
Reading a file character by character in C++ can be a fundamental task for many developers, especially when dealing with text processing or file manipulation. This method allows you to analyze each character individually, which can be particularly useful for applications like text editors, compilers, or data parsers.
In this article, we will explore various methods to read a file character by character in C++. We will also provide clear code examples and detailed explanations to help you understand the process better. Whether you’re a beginner or an experienced programmer, this guide will equip you with the knowledge you need to handle file reading effectively.
Method 1: Using fstream
One of the most common ways to read a file character by character in C++ is by using the fstream
library. This method allows you to open a file and read its contents sequentially, character by character.
Here’s a simple example to illustrate this approach:
#include <iostream>
#include <fstream>
int main() {
std::ifstream file("example.txt");
char ch;
if (file.is_open()) {
while (file.get(ch)) {
std::cout << ch;
}
file.close();
} else {
std::cerr << "Unable to open file";
}
return 0;
}
In this code snippet, we first include the necessary headers: iostream
for input-output operations and fstream
for file handling. We then declare an input file stream file
and a character variable ch
. After checking if the file opens successfully, we enter a loop that reads each character using file.get(ch)
. If the end of the file is reached, the loop terminates. Finally, we close the file to free up resources. If the file cannot be opened, an error message is displayed.
Output:
Content of example.txt
This method is straightforward and effective for reading files character by character. It provides a clear way to handle file streams and is suitable for both small and large files.
Method 2: Using std::getline with a String
Another method to read a file character by character is by using std::getline
to read the entire line and then iterating through each character of that line. This approach can be particularly useful when you want to process the file line by line before accessing individual characters.
Here is how you can implement this:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
if (file.is_open()) {
while (std::getline(file, line)) {
for (char ch : line) {
std::cout << ch;
}
}
file.close();
} else {
std::cerr << "Unable to open file";
}
return 0;
}
In this code, we use std::getline
to read each line from the file into a string variable line
. We then use a range-based for loop to iterate through each character in the line. This method allows us to handle larger blocks of text while still being able to process individual characters as needed. After processing, we close the file as before.
Output:
Content of example.txt
Using std::getline
can be advantageous when dealing with files that contain multiple lines, as it allows you to handle each line distinctly before diving into character-level processing.
Method 3: Use the getc
Function
Another method to read file char by char is to use getc
function, which takes FILE*
stream as an argument and reads the next character if available. Next, the input file stream should be iterated until the last char
is reached, and that’s implemented with the feof
function, checking if the end of the file has been reached. Note that it’s always recommended to close the open file streams once they are no longer needed.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<char> bytes;
FILE* input_file = fopen(filename.c_str(), "r");
if (input_file == nullptr) {
return EXIT_FAILURE;
}
unsigned char character = 0;
while (!feof(input_file)) {
character = getc(input_file);
cout << character << "-";
}
cout << endl;
fclose(input_file);
return EXIT_SUCCESS;
}
The above code demonstrates file input operations using C-style I/O functions in a C++ program. It opens a file named input.txt
using fopen
, reads it character by character with getc
, and prints each character followed by a dash.
The program first attempts to open the file. If unsuccessful (i.e., input_file
is nullptr
), it exits with a failure status. It then enters a loop that continues until the end of the file is reached (feof
). Each character is read using getc
and immediately printed.
This approach mixes C and C++ styles, using C’s file I/O functions (fopen
, feof
, getc
, fclose
) within a C++ program. While functional, it’s worth noting that in modern C++, it’s generally preferred to use C++ stream classes for file I/O for better type safety and exception handling.
Method 4: Using C-style FILE* and fgetc
For those who prefer a more traditional approach, you can use C-style file handling with FILE*
and the fgetc
function. This method gives you a lower-level access to file reading, which some programmers may find more familiar.
Here’s a simple implementation:
#include <stdio.h>
int main() {
FILE *file = fopen("example.txt", "r");
int ch;
if (file) {
while ((ch = fgetc(file)) != EOF) {
putchar(ch);
}
fclose(file);
} else {
perror("Unable to open file");
}
return 0;
}
In this example, we use fopen
to open the file in read mode. We then use fgetc
in a loop to read each character until we reach the end of the file (EOF). The putchar
function is used to output each character to the standard output. Finally, we close the file using fclose
. If the file cannot be opened, we use perror
to print an error message.
Output:
Content of example.txt
This method is particularly useful for programmers who are accustomed to C-style input/output functions and provides direct control over file operations.
Conclusion
Reading a file character by character in C++ is a fundamental skill that can enhance your programming toolkit. Whether you choose to use fstream
, std::getline
, or C-style file handling, each method has its own advantages and use cases. By mastering these techniques, you can effectively manipulate text files, parse data, and build applications that require precise control over file contents. With the examples and explanations provided in this article, you should feel confident in your ability to read files character by character in C++.
FAQ
-
What is the best method to read a file character by character in C++?
The best method depends on your specific needs.fstream
is commonly used for its simplicity, whilestd::getline
is useful for line-based processing. C-style methods offer low-level control. -
Can I read binary files character by character in C++?
Yes, you can read binary files character by character using the same methods, but ensure you open the file in binary mode to avoid data corruption. -
What happens if the file does not exist?
If the file does not exist, the program will typically display an error message indicating that the file could not be opened. -
Is it necessary to close the file after reading?
Yes, it is good practice to close the file after reading to free up system resources and avoid potential file corruption. -
Can I read files larger than memory using these methods?
Yes, these methods allow you to read files character by character, which means you can process large files without loading them entirely into memory.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook