C++에서 한 단어 씩 파일 읽기
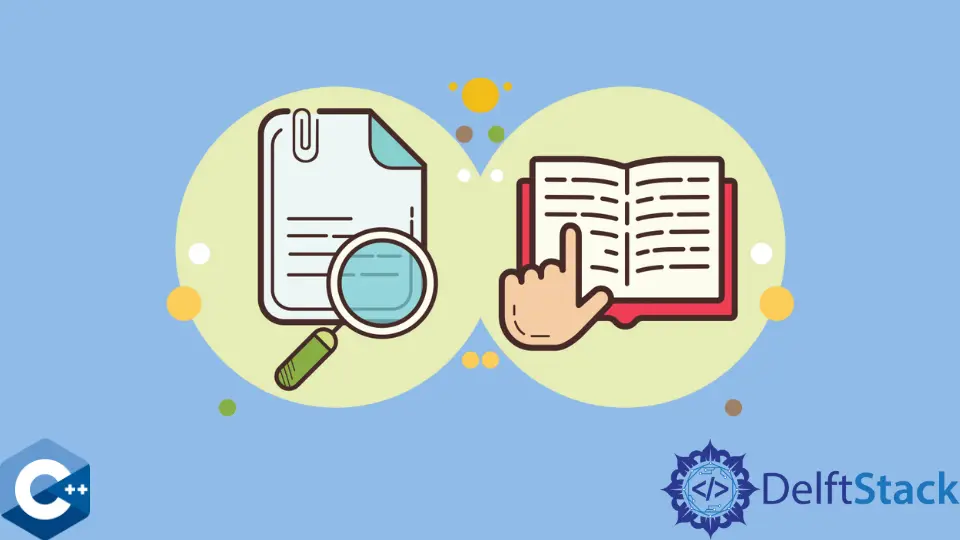
이 기사는 C++에서 단어 단위로 파일을 읽는 방법에 대한 여러 방법을 보여줍니다.
std::ifstream
을 사용하여 C++에서 한 단어 씩 파일 읽기
std::ifstream
클래스를 사용하여 입력 작업 파일 기반 스트림을 수행 할 수 있습니다. 즉,std::ifstream
유형은 파일 버퍼와 인터페이스하고 추출 연산자를 사용하여 작동하는 데 사용됩니다. std::fstream
유형은 추출 (>>
) 및 삽입 연산자 (<<
)와 호환되는 I/O 라이브러리에도 제공됩니다.
먼저 생성자 중 하나를 호출하여ifstream
유형의 객체를 만들어야합니다. 이 경우 파일 이름 문자열 만 생성자 함수에 전달됩니다. ifstream
객체가 생성되면 해당 메소드 중 하나 인is_open
을 호출하여 호출이 성공했는지 확인한 다음 계속해서 파일 내용을 읽어야합니다.
파일을 단어 단위로 읽으려면ifstream
객체에서 추출 연산자를 호출합니다. 첫 번째 공백 문자를 만나기 전에 자동으로 첫 번째 단어를 읽는 문자열 변수로 리디렉션합니다. 파일이 끝날 때까지 각 단어를 읽어야하므로 추출 문을while
루프 표현식에 삽입합니다. 또한 우리는 문자열의벡터
를 선언하여 모든 반복에 각 단어를 저장하고 나중에 별도의 루프 블록으로 인쇄합니다.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<string> words;
string word;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file >> word) {
words.push_back(word);
}
for (const auto &i : words) {
cout << i << endl;
}
input_file.close();
return EXIT_SUCCESS;
}
std::ispunct
및std::string::erase
함수를 사용하여 C++에서 구두점 기호 구문 분석
이전 방법의 유일한 단점은 단어에 가까운 구두점 문자를 대상벡터
에 저장한다는 것입니다. 각 단어를 구문 분석 한 다음vector
컨테이너에 저장하는 것이 좋습니다. 단일 문자를int
매개 변수로 취하고 문자가 구두점이면 0이 아닌 정수 값을 리턴하는ispunct
함수를 사용하고 있습니다. 그렇지 않으면 0이 반환됩니다.
주어진 인수가unsigned char
로 표현할 수없는 경우ispunct
함수의 동작은 정의되지 않습니다. 따라서 문자를 해당 유형으로 캐스트하는 것이 좋습니다. 다음 예제에서는 두 개의 간단한if
조건을 구현하여 각 단어의 첫 번째와 마지막 문자를 확인합니다. 구두점이 발견되면 내장 문자열 함수 인erase
를 호출하여 발견 된 문자를 제거합니다.
#include <fstream>
#include <iostream>
#include <vector>
using std::cerr;
using std::cout;
using std::endl;
using std::ifstream;
using std::string;
using std::vector;
int main() {
string filename("input.txt");
vector<string> words;
string word;
ifstream input_file(filename);
if (!input_file.is_open()) {
cerr << "Could not open the file - '" << filename << "'" << endl;
return EXIT_FAILURE;
}
while (input_file >> word) {
if (ispunct(static_cast<unsigned char>(word.back())))
word.erase(word.end() - 1);
else if (ispunct(static_cast<unsigned char>(word.front())))
word.erase(word.begin());
words.push_back(word);
}
for (const auto &i : words) {
cout << i << endl;
}
input_file.close();
return EXIT_SUCCESS;
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook