How to Use the STL Stringstream Class in C++
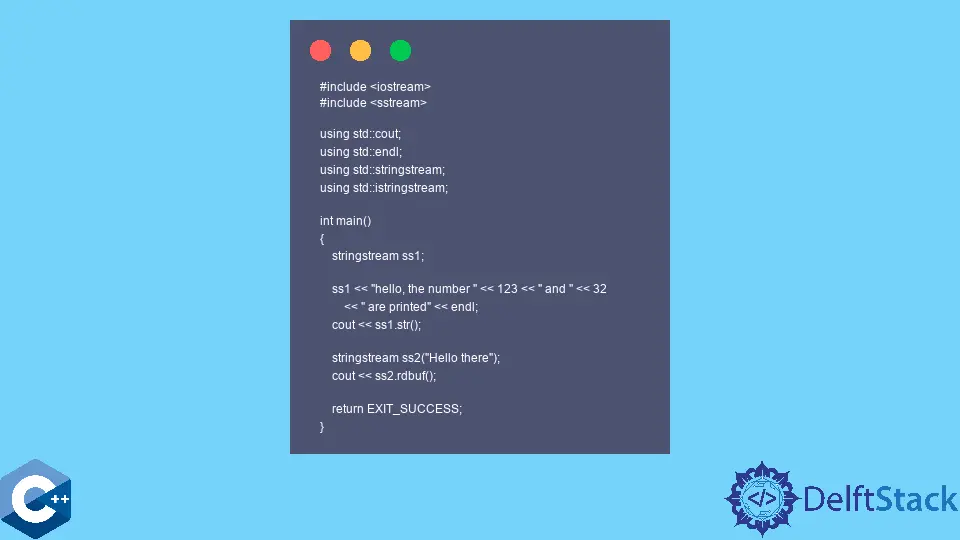
This article will demonstrate how to use the STL stringstream
class in C++.
Use the stringstream
Class to Conduct Input/Output Operations on String Streams in C++
There are generally three types of STL stream-based I/O library classes: character-based, file, and string. Each of them is usually utilized for scenarios best suited to their properties. Namely, string strings don’t provide a temporary string buffer to store their data, in contrast to connecting to some I/O channel. The std::stringstream
class implements input/output operations for string-based streams. You can treat an object of this type as a string buffer that can be manipulated using stream operations and powerful member functions.
Two of the most useful functions included in stringstream
class are str
and rdbuf
. The first one is used to retrieve the copy of the underlying string object. Take note that the returned object is temporary and destroys the end of the expression. So, you should call a function from the result of the str
function.
The same process can be called with a string object as an argument to set the contents of the stringstream
with the provided value. You should always call the str
function on the stringstream
object when it’s being inserted into a cout
stream, or else a compiler error will occur. Note also that the previous behavior is implemented since the C++11 standard.
On the other hand, the rdbuf
member function can be used to retrieve the pointer to the underlying raw string object. This one acts as if the string object is passed to the cout
stream. Consequently, the contents of the buffer are printed, as shown in the following example.
#include <iostream>
#include <sstream>
using std::cout;
using std::endl;
using std::istringstream;
using std::stringstream;
int main() {
stringstream ss1;
ss1 << "hello, the number " << 123 << " and " << 32 << " are printed" << endl;
cout << ss1.str();
stringstream ss2("Hello there");
cout << ss2.rdbuf();
return EXIT_SUCCESS;
}
Output:
hello, the number 123 and 32 are printed
Hello there
Another feature of a stringstream
class is that it can convert numeric values to a string type. Notice that the previous code snippet inserted the string literals and numbers into a stringstream
object. Additionally, when we retrieve the contents using str
function, the whole thing is a string type and can be treated as such.
There are three distinct string-based stream objects: stringstream
, istringstream
, and ostringstream
. The latter two are different because they only provide input and output operations, respectively; this implies that some member functions only affect certain stream types.
For example, the following code sample calls the putback
function on the stringstream
and istringstream
objects separately. The putback
member function is used to append the single character to the input stream. Thus, it only succeeds on a stringstream
object that provides input and output properties.
#include <iostream>
#include <sstream>
using std::cout;
using std::endl;
using std::istringstream;
using std::stringstream;
int main() {
stringstream ss2("Hello there");
cout << ss2.rdbuf();
if (ss2.putback('!'))
cout << ss2.rdbuf() << endl;
else
cout << "putback failed" << endl;
istringstream ss3("Hello there");
ss3.get();
if (ss3.putback('!'))
cout << ss3.rdbuf() << endl;
else
cout << "putback failed" << endl;
return EXIT_SUCCESS;
}
Output:
Hello there!
putback failed
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook