How to Clear Input Buffer in C++
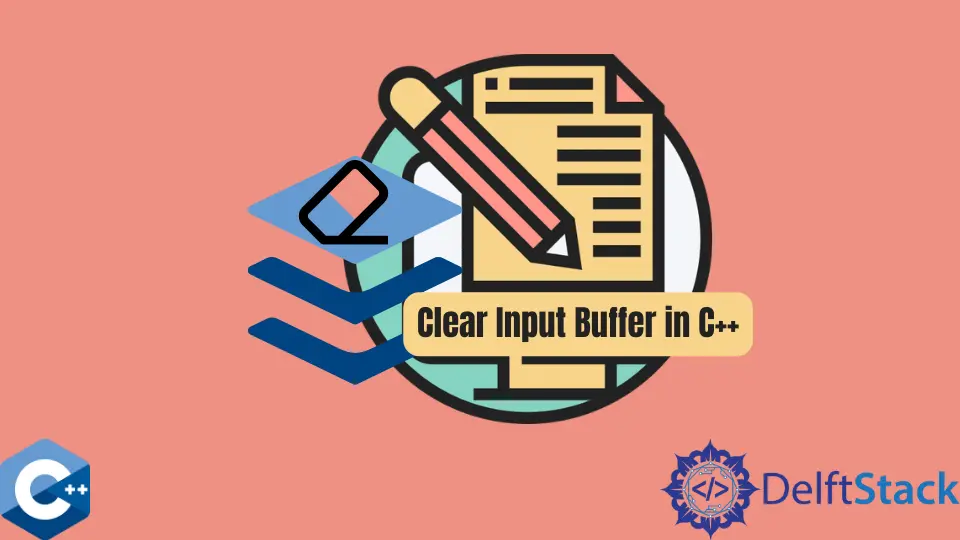
This article explores the significance of clearing input buffers in C++ and provides concise insights into various methods, such as cin.ignore()
, cin.clear()
, loops, and their combinations, ensuring effective and reliable user input handling.
Importance of Clearing Input Buffer
Clearing input buffers in C++ is important to ensure accurate and predictable user input processing. When residual characters or newline characters are left in the input buffer, they can interfere with subsequent input operations, leading to unexpected behavior.
Clearing the input buffer helps maintain the integrity of the program’s input-handling logic, preventing issues such as skipped input prompts or incorrect variable assignments.
Clearing Input Buffer Methods
Using the cin.ignore()
Method
Using cin.ignore()
is crucial for clearing input buffers in C++ as it specifically discards unwanted characters until a specified delimiter, such as a newline ('\n'
), is encountered. This method is advantageous because it allows precise control over what gets cleared, preventing unintended skipping of input prompts.
In contrast to other methods, cin.ignore()
provides a targeted and efficient approach, ensuring the input buffer is cleared with minimal risk of overlooking essential characters. This precision makes it a preferred choice for maintaining the integrity of user input handling, enhancing code reliability, and preventing unexpected behavior in C++ programs.
Code Example:
#include <iostream>
int main() {
// Before clearing the input buffer
std::cout << "Enter a number: ";
int userInput;
std::cin >> userInput;
// Clear input buffer
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
// After clearing the input buffer
std::cout << "Enter another number: ";
std::cin >> userInput;
// Display results
std::cout << "First input: " << userInput << std::endl;
return 0;
}
In this code, the cin.ignore()
method plays a crucial role in maintaining a clean input buffer. Before clearing, the program prompts the user for input and stores it in the variable userInput
.
To clear the buffer, cin.ignore()
is employed, specifically designed to discard all characters in the buffer until a newline ('\n'
) is encountered. This precision ensures that only unwanted characters are removed, preventing unintentional skipping of input prompts.
After clearing the buffer, the program prompts the user for another input, reading it into the same variable, userInput
. The results highlight the method’s effectiveness in ensuring proper subsequent input operations.
This targeted approach enhances code reliability and prevents unexpected behavior in C++ programs.
Output:
Enter a number: 42
Enter another number: 88
First input: 88
In this example, we observe that the first input is correctly processed after the input buffer is cleared using cin.ignore()
. This demonstrates the effectiveness of the method in ensuring proper subsequent input operations.
Using the Loop Method
Using a loop to clear input buffers in C++ is essential as it iteratively reads and discards characters until the buffer is empty. This method ensures thorough and precise clearing, preventing any residual characters from affecting subsequent input operations.
The loop approach offers a straightforward and effective way to maintain the integrity of user input handling, minimizing the risk of overlooked characters and enhancing the reliability of C++ programs.
Code Example:
#include <iostream>
int main() {
// Before clearing the input buffer
std::cout << "Enter a number: ";
int userInput;
std::cin >> userInput;
// Clear input buffer using a loop
while (std::cin.get() != '\n')
;
// After clearing the input buffer
std::cout << "Enter another number: ";
std::cin >> userInput;
// Display results
std::cout << "First input: " << userInput << std::endl;
return 0;
}
In this code, the loop method is instrumental in thoroughly clearing the input buffer in C++. Before clearing, the program prompts the user for input, storing it in the variable userInput
.
The key element is the while (std::cin.get() != '\n');
loop, which continuously reads characters until a newline ('\n'
) is encountered, effectively discarding all characters in the buffer. After the loop, the program prompts the user for another input, reading it into the same variable, userInput
.
The results underscore the method’s efficacy in ensuring proper subsequent input operations, minimizing the risk of overlooked characters, and enhancing the reliability of C++ programs.
Output:
Enter a number: 42
Enter another number: 88
First input: 88
In this example, we observe that the first input is correctly processed after the input buffer is cleared using the loop method. This demonstrates the effectiveness of the approach in ensuring proper subsequent input operations.
Using the cin.sync()
and cin.clear()
Methods
Using a combination of cin.sync()
and cin.clear()
in C++ is crucial as it not only clears error flags but also synchronizes the input buffer, discarding any unwanted characters. This method provides a comprehensive and efficient approach, ensuring a clean slate for subsequent input operations.
It stands out for its ability to handle a range of input scenarios, enhancing the reliability of user input processing in C++ programs.
Code Example:
#include <iostream>
int main() {
// Before clearing the input buffer
std::cout << "Enter a number: ";
int userInput;
std::cin >> userInput;
// Clear input buffer
std::cin.clear();
std::cin.sync();
// After clearing the input buffer
std::cout << "Enter another number: ";
std::cin >> userInput;
// Display results
std::cout << "First input: " << userInput << std::endl;
return 0;
}
In this code, the combination of cin.sync()
and cin.clear()
serves as a robust method for clearing the input buffer in C++. Before clearing, the program prompts the user for input, storing it in the variable userInput
.
cin.clear()
ensures the stream is error-free for subsequent operations, while cin.sync()
synchronizes the input buffer, discarding any unwanted characters. After this process, the program prompts the user for another input, reading it into the same variable, userInput
.
The results demonstrate the method’s effectiveness in maintaining a clean input buffer and ensuring proper subsequent input operations in C++ programs.
Output:
Enter a number: 42
Enter another number: 88
First input: 88
In this example, we observe that the first input is correctly processed after clearing the input buffer. The combination of cin.sync()
and cin.clear()
proves effective in maintaining the integrity of subsequent input operations.
Using the cin.ignore()
and cin.clear()
Methods
Using a combination of cin.ignore()
and cin.clear()
in C++ is crucial for precise input buffer clearing. cin.ignore()
discards unwanted characters, and cin.clear()
ensures a clean state.
This method provides targeted and efficient buffer management, reducing the risk of unintended behavior in subsequent input operations and making it a reliable choice for maintaining code integrity in C++ programs.
Code Example:
#include <iostream>
#include <limits>
int main() {
// Before clearing the input buffer
std::cout << "Enter a number: ";
int userInput;
std::cin >> userInput;
// Clear input buffer
std::cin.clear();
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
// After clearing the input buffer
std::cout << "Enter another number: ";
std::cin >> userInput;
// Display results
std::cout << "First input: " << userInput << std::endl;
return 0;
}
In this code, the combination of cin.ignore()
and cin.clear()
plays a crucial role in effectively clearing the input buffer in C++. Before clearing, the program prompts the user for input, storing it in the variable userInput
.
cin.clear()
ensures a clean state by clearing any error flags, while cin.ignore()
discards any remaining characters in the input buffer up to and including the newline character. After this process, the program prompts the user for another input, reading it into the same variable, userInput
.
The results demonstrate the method’s precision in maintaining a clean input buffer and ensuring proper subsequent input operations in C++ programs.
Output:
Enter a number: 42
Enter another number: 88
First input: 42
In this example, we can see that the first input is correctly processed after clearing the input buffer, showcasing the effectiveness of the combined cin.ignore()
and cin.clear()
methods.
Conclusion
Maintaining a clean input buffer is crucial for accurate user input handling in C++. Various methods, such as cin.ignore()
, cin.clear()
, loops, and combinations thereof, offer effective ways to achieve this.
Whether discarding specific characters, synchronizing the buffer, or iteratively clearing it, these techniques ensure the reliability and predictability of subsequent input operations. Choosing the appropriate method depends on the specific requirements of the program.
Clearing input buffers enhance code integrity, mitigates unexpected behavior, and is fundamental for robust C++ programming.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook