How to Validate User Input in C++
-
How to Validate User Input in C++ Using
cin
With thecin.clear()
andcin.ignore()
Methods - How to Validate User Input in C++ Using a Custom-Defined Function
-
How to Validate User Input in C++ Using the
cin.fail()
Function -
How to Validate User Input in C++ Using the
getline()
Function - Conclusion
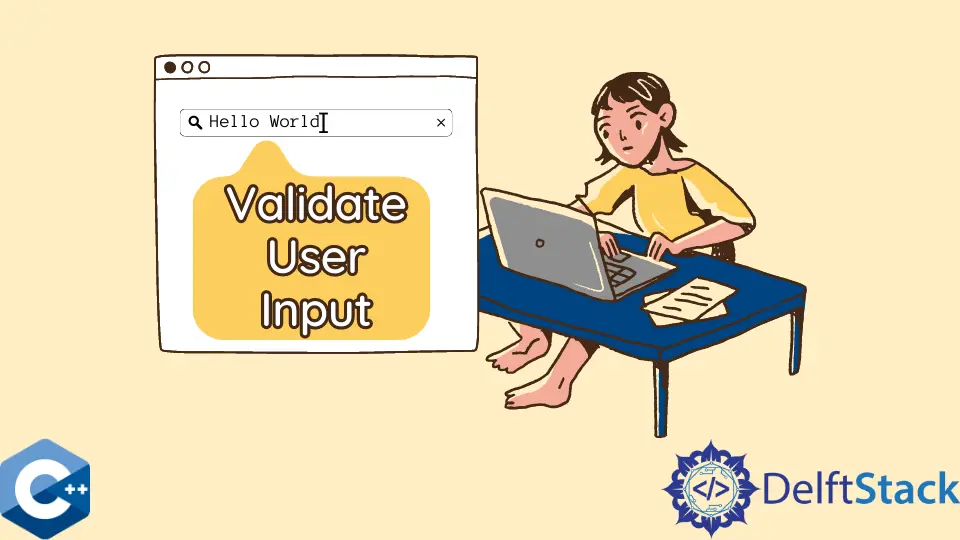
Validating user input is a fundamental aspect of programming, especially in languages like C++, where manual memory management and direct access to system resources are common. In C++, ensuring that user input meets certain criteria is essential for program correctness, security, and usability.
Whether it’s validating data types, handling unexpected input, or managing whitespace characters, implementing robust input validation techniques is critical for creating reliable and user-friendly applications. In this article, we’ll explore various methods and techniques for validating user input in C++ to ensure that the program interacts with users in a predictable and error-free manner.
How to Validate User Input in C++ Using cin
With the cin.clear()
and cin.ignore()
Methods
One common approach to validate user input in C++ involves using cin
along with cin.clear()
and cin.ignore()
methods. This method allows for robust error checking and recovery from unexpected input, ensuring a smoother user experience.
The cin
stream in C++ is used to read input from the user. However, when the input does not match the expected type, the failbit
flag of the cin
stream gets set, indicating a failure in the input operation.
To handle this, we use the cin.clear()
method to clear any error flags set on the cin
stream. This ensures that subsequent input operations can proceed normally.
Additionally, the cin.ignore()
method is used to discard any remaining characters in the input buffer. This is necessary because when an input operation fails, the incorrect characters remain in the input buffer, potentially causing subsequent input operations to fail as well.
By clearing the input buffer, we can prevent this issue and prompt the user for input again.
Let’s delve into how this method works and provide a code example.
#include <iostream>
#include <limits>
using namespace std;
int main() {
int age;
while (true) {
cout << "Enter the age of the wine: ";
if (cin >> age) {
break;
} else {
cout << "Invalid input. Please enter a valid integer value!" << endl;
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(), '\n');
}
}
cout << "Age of the wine entered: " << age << endl;
return 0;
}
In this code example, we first declare a variable age
to store the user input. We then enter a while
loop with a condition set to true
, ensuring continuous prompting until valid input is received.
Within the loop, we display a message asking the user to enter the age of the wine.
Next, we attempt to read an integer value from the user input using cin >> age
. If the input operation succeeds (i.e., the user enters a valid integer), we break out of the loop and proceed with the rest of the program.
However, if the input operation fails (e.g., due to non-integer input), we enter the else
block. Here, we print an error message indicating invalid input and proceed to clear any error flags on the cin
object using cin.clear()
.
Additionally, we use cin.ignore()
to discard any extraneous characters present in the input buffer, ensuring a clean input stream for the next input operation.
After clearing error flags and discarding extraneous characters, the loop continues, prompting the user to enter input again until a valid integer is provided.
Output:
This demonstrates the functionality of the code, where it repeatedly prompts the user until a valid integer input is provided and then proceeds with the rest of the program.
How to Validate User Input in C++ Using a Custom-Defined Function
Another effective approach to validate user input in C++ involves defining a custom function.
This method helps streamline the validation process, especially when dealing with multiple input variables. By encapsulating the validation logic within a function, we can enhance code readability and reusability.
To implement input validation using a custom-defined function, we first declare the function template. The function takes a reference to a variable as its argument and returns the validated value.
template <typename T>
T validateInput(T& input) {
// Validation logic
// Prompt user for input
// Check if the input is valid
// Return validated input
}
Inside the function, we will use a while
loop similar to the previous approach. The difference lies in the fact that the while
loop is now encapsulated within the function, making the main
code cleaner and more organized.
The function repeatedly prompts the user for input until a valid value is obtained, using cin.clear()
and cin.ignore()
to handle incorrect input.
Here’s an example demonstrating how to validate user input using a custom-defined function:
#include <iostream>
#include <limits>
using namespace std;
template <typename T>
T &validateInput(T &val, const string &prompt) {
while (true) {
cout << prompt;
if (cin >> val) {
break;
} else {
cout << "Invalid input. Please enter a valid value.\n";
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(), '\n');
}
}
return val;
}
int main() {
int age;
int volume;
int price;
validateInput(age, "Enter the age of the wine: ");
validateInput(volume, "Enter the volume of the wine in ml: ");
validateInput(price, "Enter the price of the wine: ");
cout << "\nYour order's in!\n"
<< age << " years old Cabernet"
<< " in " << volume << "ml bottle\nPrice: " << price << "$\n";
return 0;
}
In our C++ program, we’re focused on validating user input, particularly for a wine ordering system. We’ve implemented a custom function called validateInput
to handle input validation efficiently. This function takes advantage of C++ templates to ensure flexibility in handling various data types.
Within the validateInput
function, we use a while
loop to repeatedly prompt the user for input until a valid value is obtained. If the input operation fails, indicating that the user has entered invalid input, we output an error message and clear the error flags using cin.clear()
.
Additionally, we discard any remaining characters in the input buffer using cin.ignore()
. This ensures that subsequent input operations are not affected by the incorrect input.
Moving on to the main()
function, we declare three integer variables: age
, volume
, and price
, which will store the user’s inputs.
We then call the validateInput
function for each variable to validate the user’s input. This function handles the validation process, ensuring that the user provides valid input before proceeding.
Finally, once valid inputs are provided, we output the validated inputs along with a message indicating the user’s order details.
Output:
By encapsulating the input validation logic within a custom function and using appropriate error-handling techniques, our program ensures that user input is validated effectively, enhancing the reliability and usability of our wine ordering system.
How to Validate User Input in C++ Using the cin.fail()
Function
Another effective approach to validate user input in C++ involves utilizing the cin.fail()
function. This method allows us to check whether the input operation has failed due to an invalid type or format, providing a straightforward way to handle erroneous input.
The cin.fail()
function returns true
if the last input operation failed. We can use this function to determine whether the user’s input is valid.
After reading the user’s input using cin
, we can immediately check if cin.fail()
returns true
. If it does, we know that the input was invalid, and we can take appropriate action to handle the error.
This may include displaying an error message to the user, clearing the error flags using cin.clear()
, and discarding any remaining characters in the input buffer using cin.ignore()
.
Here’s a code example demonstrating how to validate user input using cin.fail()
:
#include <iostream>
#include <limits>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
while (cin.fail()) {
cout << "Invalid input. Please enter a valid integer.\n";
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(), '\n');
cout << "Enter your age: ";
cin >> age;
}
cout << "You entered: " << age << endl;
return 0;
}
In this code, we first declare an integer variable age
to store the user’s input. We then prompt the user to enter their age using cout
.
Afterward, we read the user’s input into the age
variable using cin
. Immediately after the input operation, we use a while
loop to continuously check whether cin.fail()
returns true
.
If it does, we output an error message indicating that the input was invalid. We then clear the error flags using cin.clear()
, discard any remaining characters in the input buffer using cin.ignore()
, and prompt the user again for input.
If cin.fail()
returns false
, indicating that the input was successful, we output the user’s entered age to the console.
Output:
In this example, if the user enters a non-integer value (such as "abc"
), the program will output an error message indicating that the input was invalid.
How to Validate User Input in C++ Using the getline()
Function
Another method to validate user input in C++ involves using the getline()
function, which is particularly useful when dealing with strings or when expecting input that may contain whitespace characters.
The getline()
function in C++ is typically used to read a line of text from an input stream, such as std::cin
, and store it into a string variable. Its syntax is as follows:
#include <iostream>
#include <string>
std::istream& getline(std::istream& is, std::string& str, char delim = '\n');
Where:
is
: The input stream from whichgetline()
will read the input.str
: The string variable where the input line will be stored.delim
: (Optional) The delimiter character used to determine the end of the input line. By default, it is the newline character'\n'
.
The getline()
function reads characters from the input stream is
until either the specified delimiter character (delim
) is encountered or the end of the file is reached. It then stores these characters, excluding the delimiter, into the string variable str
.
Here’s a code example demonstrating how to validate user input using getline()
:
#include <iostream>
#include <limits>
using namespace std;
int main() {
string name;
cout << "Enter your name: ";
getline(cin, name);
while (name.empty()) {
cout << "Invalid input. Please enter a non-empty name.\n";
cout << "Enter your name: ";
getline(cin, name);
}
cout << "Hello, " << name << "!" << endl;
return 0;
}
First, we prompt the user to enter their name using cout
. We then use the getline()
function to read the user’s input from the standard input stream (cin
) and store it in the name
string variable.
This allows us to handle input that may contain whitespace characters, ensuring that the entire name is captured.
Next, we set up a while
loop to continuously check if the input string is empty using the empty()
function. If the input is found to be empty, indicating that the user did not provide any characters, we display an error message prompting the user to enter a non-empty name.
The loop continues until the user provides a non-empty input. Once a non-empty name is provided, the while
loop terminates, and we output a greeting message using cout
, addressing the user by their entered name.
Output:
In this example, if the user enters an empty name, the program displays an error message and prompts the user again until a non-empty name is provided. Once a non-empty name is entered, the program greets the user accordingly.
Conclusion
In conclusion, validating user input is a crucial aspect of programming in C++ to ensure that the program operates smoothly and handles user interactions gracefully.
Throughout this article, we’ve explored various methods for validating user input, including using cin
with cin.clear()
and cin.ignore()
methods, custom-defined functions, cin.fail()
, and getline()
. Each method offers its advantages and can be chosen based on the specific requirements of the program.
By using these validation techniques, we can enhance the reliability and robustness of our C++ programs, providing users with a more intuitive and error-free experience. Whether it’s checking for valid data types, handling empty inputs, or managing whitespace characters, effective input validation is essential for building robust and user-friendly C++ applications.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook