C++ でユーザー入力を検証する
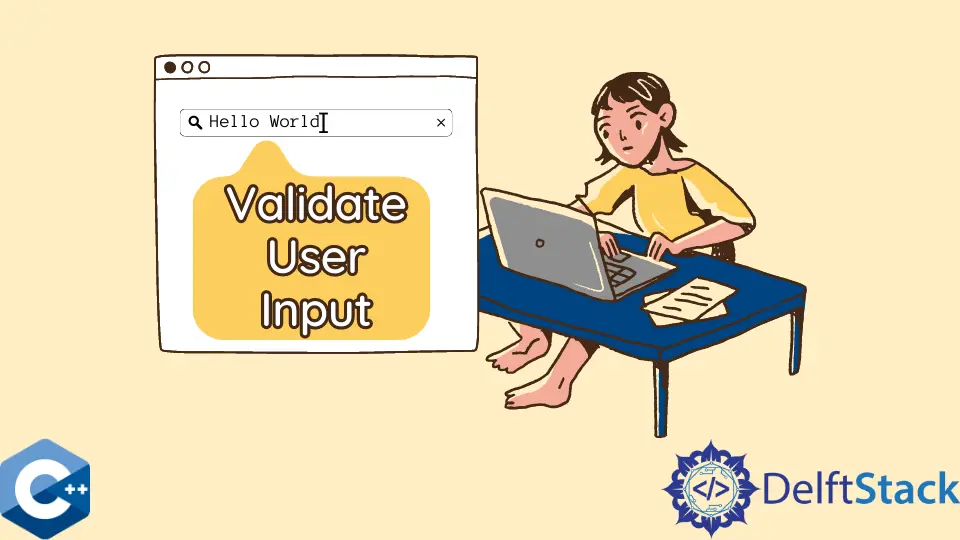
この記事では、C++ でユーザ入力を検証する方法について複数のメソッドを紹介します。
ユーザ入力を検証するために cin.clear
と cin.ignore
メソッドと一緒に cin
を使用する
つまり、基本的なエラーチェックの要件を満たし、入力の型が間違っていても実行を継続する必要があるということです。
この機能を実装するための最初の構成は while
ループであり、条件に true
を指定します。この繰り返しにより、正しい値が変数に格納されるまで繰り返し動作が保証されています。ループ内では、if
文が cin >> var
式を評価します。値が正しく格納されればループを抜けることができます。そうでなければ、cin
式が成功しなかった場合、実行は cin.clear
コールに移ります。次に、入力バッファに残っている文字をスキップして次の反復処理に進み、ユーザに質問します。
#include <iostream>
#include <limits>
#include <sstream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::numeric_limits;
using std::string;
using std::vector;
int main() {
int age;
while (true) {
cout << "Enter the age of the wine: ";
if (cin >> age) {
break;
} else {
cout << "Enter a valid integer value!\n";
cin.clear();
cin.ignore(numeric_limits<std::streamsize>::max(), '\n');
}
}
return EXIT_SUCCESS;
}
出力:
Enter the age of the wine: 32
カスタム定義関数を使ってユーザの入力を検証する
これまでの方法では、複数の入力変数でもかなり面倒で、複数の while
ループでコードスペースが無駄になってしまいます。そこで、このサブルーチンは関数として一般化する必要があります。validateInput
は変数の参照を受け取り、正常に格納された値を返す関数テンプレートです。
100 問の小テストを実装する必要があったとしても、このメソッドは以前のバージョンよりもはるかにクリーンなコードを保証します。
#include <iostream>
#include <limits>
#include <sstream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::numeric_limits;
using std::string;
using std::vector;
template <typename T>
T &validateInput(T &val) {
while (true) {
cout << "Enter the age of the wine: ";
if (cin >> val) {
break;
} else {
cout << "Enter a valid integer value!\n";
cin.clear();
cin.ignore(numeric_limits<std::streamsize>::max(), '\n');
}
}
return val;
}
int main() {
int age;
int volume;
int price;
age = validateInput(age);
volume = validateInput(volume);
price = validateInput(price);
cout << "\nYour order's in!\n"
<< age << " years old Cabernet"
<< " in " << volume << "ml bottle\nPrice: " << price << "$\n";
return EXIT_SUCCESS;
}
出力:
Enter the age of the wine: 13
Enter the volume of the wine in milliliters: 450
Enter the price of the bottle: 149
Your order's in!
13 years old Cabernet in 450ml bottle
Price: 149$
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: 胡金庫