C++에서 사용자 입력 유효성 검사
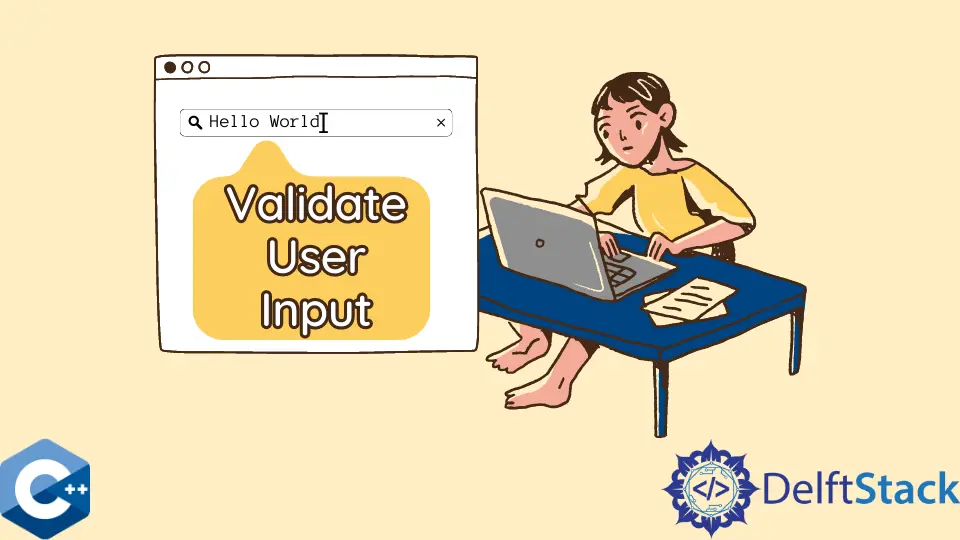
이 기사에서는 C++에서 사용자 입력의 유효성을 검사하는 방법에 대한 여러 방법을 보여줍니다.
cin.clear
및cin.ignore
메서드와 함께cin
을 사용하여 사용자 입력 유효성 검사
이 예제는 강력한 사용자 입력 유효성 검사 방법에 중점을 둡니다. 즉, 기본 오류 검사 요구 사항을 충족하고 입력 유형이 잘못된 경우에도 계속 실행해야합니다.
이 기능을 구현하는 첫 번째 구성은while
루프이며 조건에true
를 지정합니다. 이 반복은 올바른 값이 변수에 저장 될 때까지 반복되는 동작을 보장합니다. 루프 내에서if
문은 성공적인 삽입 작업에서 반환 값이 양수이므로cin >> var
표현식을 평가합니다. 값이 올바르게 저장되면 루프를 종료 할 수 있습니다. 그렇지 않으면cin
표현식이 성공하지 못하면 실행이cin.clear
호출로 이동하여 예기치 않은 입력 후 failbit
를 설정 해제합니다. 다음으로 입력 버퍼의 남은 문자를 건너 뛰고 다음 반복으로 이동하여 사용자에게 묻습니다.
#include <iostream>
#include <limits>
#include <sstream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::numeric_limits;
using std::string;
using std::vector;
int main() {
int age;
while (true) {
cout << "Enter the age of the wine: ";
if (cin >> age) {
break;
} else {
cout << "Enter a valid integer value!\n";
cin.clear();
cin.ignore(numeric_limits<std::streamsize>::max(), '\n');
}
}
return EXIT_SUCCESS;
}
출력:
Enter the age of the wine: 32
사용자 정의 함수를 사용하여 사용자 입력 유효성 검사
이전 방법은 여러 입력 변수에 대해 매우 번거로울 수 있으며 여러 개의while
루프로 인해 코드 공간이 낭비 될 수 있습니다. 따라서 서브 루틴은 함수로 일반화되어야합니다. validateInput
은 변수의 참조를 받아 성공적으로 저장된 값을 반환하는 함수 템플릿입니다.
100 개의 질문 퀴즈를 구현해야하더라도이 방법은 이전 버전보다 훨씬 깔끔한 코드를 보장합니다.
#include <iostream>
#include <limits>
#include <sstream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::numeric_limits;
using std::string;
using std::vector;
template <typename T>
T &validateInput(T &val) {
while (true) {
cout << "Enter the age of the wine: ";
if (cin >> val) {
break;
} else {
cout << "Enter a valid integer value!\n";
cin.clear();
cin.ignore(numeric_limits<std::streamsize>::max(), '\n');
}
}
return val;
}
int main() {
int age;
int volume;
int price;
age = validateInput(age);
volume = validateInput(volume);
price = validateInput(price);
cout << "\nYour order's in!\n"
<< age << " years old Cabernet"
<< " in " << volume << "ml bottle\nPrice: " << price << "$\n";
return EXIT_SUCCESS;
}
출력:
Enter the age of the wine: 13
Enter the volume of the wine in milliliters: 450
Enter the price of the bottle: 149
Your order's in!
13 years old Cabernet in 450ml bottle
Price: 149$
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook