How to Right Justify Output in C++
-
Use
std::right
andstd::setw
to Right Justify Output in C++ -
Use the
printf
Function to Right Justify Output in C++
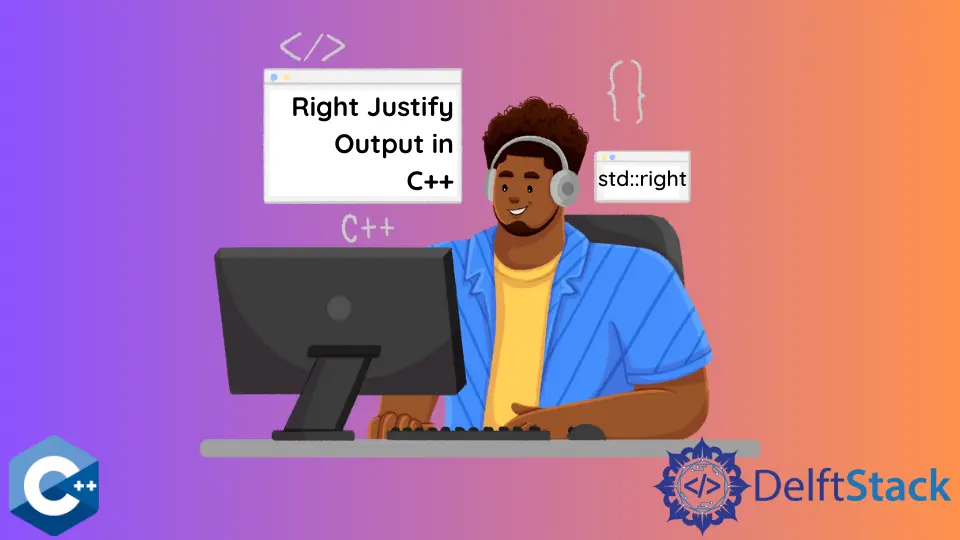
This article will demonstrate multiple methods about how to right justify the output stream in C++.
Use std::right
and std::setw
to Right Justify Output in C++
The C++ standard library provides I/O manipulator helper functions to control streams better when used with << >>
operators, and they are included in the <iomanip>
header file. std::right
is one of the stream manipulators that sets the position of fill characters. Thus it should be used in conjunction with the std::setw
manipulator function, which is used to set the stream width. std::setw
takes a single integer as an argument to specify the number of characters to allocate for the stream width.
In the next example code, we initialize a vector of double
with arbitrary values that have different precision and then output all of them as justified on the console’s right side.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::right;
using std::setw;
using std::vector;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.324, 0.012,
26.9491092019, 11013, 92.001112, 0.000000234};
for (auto &i : d_vec) {
cout << right << setw(20) << fixed << i << endl;
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
123.231000
2.234300
0.324000
0.012000
26.949109
11013.000000
92.001112
0.000000
Use the printf
Function to Right Justify Output in C++
Another powerful function to deal with I/O formatting is printf
. Even though printf
is not used with cin
/cout
streams, it can format variable arguments separately. Notice that we use the %f
format specifier for the floating-point values, and use an arbitrary number 20
to add filling characters to each element. As a result, each line is 20
character wide, and since the number is positive, the filling chars are appended from the left.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::right;
using std::setw;
using std::vector;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.324, 0.012,
26.9491092019, 11013, 92.001112, 0.000000234};
for (auto &i : d_vec) {
printf("%20f\n", i);
}
return EXIT_SUCCESS;
}
Output:
123.231000
2.234300
0.324000
0.012000
26.949109
11013.000000
92.001112
0.000000
Alternatively, we can insert a negative integer in the format specifier to fill characters on the right side, justifying output left. Another powerful feature of the printf
is to format literal string values, as demonstrated in the following sample code. Note that %c
is the char
format specifier, and 0x20
value is hexadecimal for a space character.
#include <iomanip>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::fixed;
using std::right;
using std::setw;
using std::vector;
int main() {
vector<double> d_vec = {123.231, 2.2343, 0.324, 0.012,
26.9491092019, 11013, 92.001112, 0.000000234};
for (auto &i : d_vec) {
printf("%-20f\n", i);
}
printf("%60s\n", "this ought to be justified right");
printf("%-20s|%20c|%20s\n", "wandering", 0x20, "the tower");
return EXIT_SUCCESS;
}
Output:
123.231000
2.234300
0.324000
0.012000
26.949109
11013.000000
92.001112
0.000000
this ought to be justified right
wandering | | the tower
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook