The setTimeout() Function in Angular
-
Introduction to
setTimeout()
Function in Angular -
Use the
setTimeout()
Function in Angular With IIFE and Function Recursion -
Use the
setTimeout()
Function by Passing a List of Values in Angular -
Common Use Cases of
setTimeout()
in Angular -
Solving Performance Issues Caused by
setTimeout()
andsetInterval()
in Angular - Best Practices and Solutions
- Conclusion
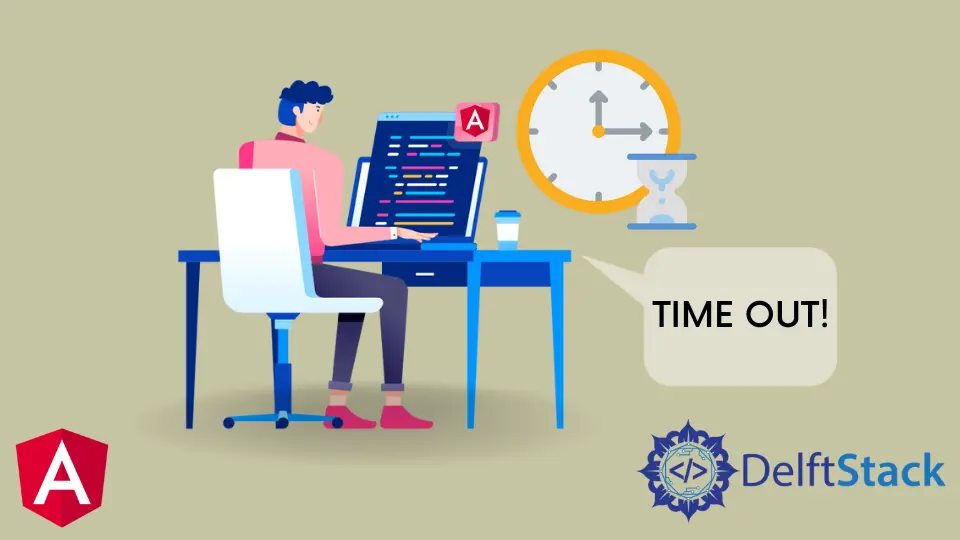
Angular, a widely used front-end framework, provides developers with a rich set of tools and functions to create dynamic web applications. One such function is setTimeout()
, a fundamental JavaScript function that plays a crucial role in managing asynchronous operations within Angular applications.
In this post, we’ll delve into the intricacies of the setTimeout()
function, its purpose, syntax, usage, and best practices within the context of Angular development. We will introduce this function using Immediately Invoked Function Expression (IIFE) and function recursion, passing a list of times we want to wait and iterate them recursively.
In Angular development, optimizing the user experience involves handling asynchronous operations with finesse. One indispensable tool for introducing controlled delays is the setTimeout()
function.
This native JavaScript feature empowers developers to orchestrate the timed execution of tasks, making it a cornerstone of responsive and efficient Angular applications.
Introduction to setTimeout()
Function in Angular
The setTimeout()
function is a JavaScript method that allows you to schedule the execution of a function after a specified amount of time has passed. This function is particularly useful in scenarios where you need to delay the execution of a piece of code, create animations, or simulate asynchronous behavior.
Syntax and Parameters:
setTimeout(function, delay, param1, param2, ...);
function
: The function to be executed after the specified delay.delay
: The time (in milliseconds) to wait before executing the function.param1, param2, ...
: Optional parameters that can be passed to the function.
When setTimeout()
is called, it registers a function to be executed once the specified delay has elapsed.
The JavaScript event loop is responsible for managing the execution of this function. It places the function in a queue after the delay period, and when the call stack is empty, it will execute the function.
Use the setTimeout()
Function in Angular With IIFE and Function Recursion
We can use the setTimeout()
function for various reasons. It is best to allow Angular to run change detection once between the actions that we would otherwise perform synchronously.
setTimeout()
is a native JavaScript function that sets a timer to execute a callback function, calling the function once the timer is done.
A powerful approach is to combine setTimeout()
with an Immediately Invoked Function Expression (IIFE) and function recursion. This technique allows Angular to execute its change detection mechanism between asynchronous tasks, maintaining UI responsiveness.
Write this simple setTimeout()
function code:
let i = 0;
let max = 5;
(function repeat(){
if (++i > 5) return;
setTimeout(function(){
console.log("waited for: " + i + " seconds");
repeat();
}, 1000);
})();
Output in the console:
In the above code block, we defined an integer i
and max
and used a repeat function to repeat our timeout until the condition is met. In this example, i
tracks the number of iterations, and max
defines the limit.
The recursive function repeat()
encapsulates setTimeout()
, adjusting delay times. This approach ensures Angular’s change detection runs between iterations.
If i
values get greater than max
, this loop will break. If the value of i
remains under the max
value, it will repeat every 1s and display waited for: 1 to 5 seconds
in the console log
.
Use the setTimeout()
Function by Passing a List of Values in Angular
If we have a list of values for which we want our program to wait and execute the callback function, we can set our function to pass an array as a parameter and run until the array is complete.
For more intricate scenarios where different delays are required for various tasks, utilizing an array of delay times is effective. This approach allows the controlled execution of tasks in a specific sequence.
Example Code:
let waitList = [5000, 4000, 3000, 1000];
function setDelay(times) {
if (times.length > 0) {
// Remove the first time from the array
let wait = times.shift();
console.log("Waiting For: " + wait/1000 + " seconds");
// Wait for the given amount of time
setTimeout(() => {
console.log("Waited For: " + wait/1000 + " seconds");
// Call the setDelay function again with the remaining times
setDelay(times);
}, wait);
}
}
setDelay(waitList);
In this code, setDelay()
processes an array of delay times sequentially. Each delay corresponds to a distinct task, creating a controlled execution sequence.
This technique is especially valuable for scenarios involving data fetching or animations that require precise timing.
Expected output:
The above code defines a function called setDelay
, which takes an array of time intervals in milliseconds as input. It recursively processes each interval, logging a message indicating the time to wait and then waits for that duration before moving to the next interval.
This simulates a sequential delay. The code executes with an example array [5000, 4000, 3000, 1000]
, resulting in output messages indicating the wait times and actual wait durations.
Common Use Cases of setTimeout()
in Angular
Angular leverages the setTimeout()
function for handling asynchronous operations, particularly when working with observables, HTTP requests, animations, and other tasks that require timing control.
import { Component } from '@angular/core';
@Component({
selector: 'app-example',
template: '<p>{{ message }}</p>'
})
export class ExampleComponent {
message: string='';
constructor() {
setTimeout(() => {
this.message = 'Delayed message displayed!';
}, 2000);
}
}
In this example, a component is defined with a property message
. Within the constructor
, setTimeout()
is used to change the value of message
after a 2-second delay.
Animations
setTimeout()
can be used in conjunction with Angular’s animation module to create timed transitions and effects.
import { animate, style, transition, trigger } from '@angular/animations';
@Component({
// ...
animations: [
trigger('fadeInOut', [
transition(':enter', [
style({ opacity: 0 }),
animate(300, style({ opacity: 1 }))
]),
transition(':leave', [
animate(300, style({ opacity: 0 }))
])
])
]
})
This code sets up an animation trigger named 'fadeInOut'
with two transitions: one for when an element enters the DOM and one for when it leaves. These transitions handle opacity changes to create a fade-in and fade-out effect.
This code can be used within an Angular component to animate elements based on specific triggers and transitions. Remember to include the necessary HTML template code and apply the animation trigger where needed for it to take effect.
HTTP Requests
Managing asynchronous HTTP requests is a common use case for setTimeout()
.
import { HttpClient } from '@angular/common/http';
@Component({
// ...
})
export class DataService {
constructor(private http: HttpClient) {}
fetchData() {
setTimeout(() => {
this.http.get('https://api.example.com/data')
.subscribe(data => {
// Handle the fetched data
});
}, 2000);
}
}
This service is designed to fetch data from an external API after a 2-second delay. It’s important to note that you’ll need to replace 'https://api.example.com/data'
with the actual API endpoint you intend to use.
Additionally, don’t forget to handle the fetched data within the subscribe
block.
Solving Performance Issues Caused by setTimeout()
and setInterval()
in Angular
Angular applications often rely on asynchronous operations for various functionalities. While setTimeout()
and setInterval()
are commonly used for asynchronous tasks, they can introduce performance issues, especially in Angular applications where the change detection mechanism and application lifecycle play significant roles.
Understanding Change Detection in Angular
Angular employs a change detection mechanism to detect and update the DOM when the application state changes. This process involves checking all components and their associated templates for any changes.
When using timers like setTimeout()
or setInterval()
, note that these asynchronous operations might cause changes to the application state, triggering unnecessary and frequent change detection cycles.
Issues Caused by Timers in Angular
- Frequent Change Detection: Timers often trigger change detection cycles, even if changes aren’t related to the DOM.
- Memory Leaks: Improper handling of timers might lead to memory leaks if they’re not cleared or properly managed.
Best Practices and Solutions
1. Use Angular’s NgZone
Angular’s NgZone
provides control over the change detection mechanism, allowing us to manage asynchronous operations more efficiently.
Example:
import { NgZone } from '@angular/core';
constructor(private zone: NgZone) {}
this.zone.runOutsideAngular(() => {
setTimeout(() => {
// Your asynchronous task
this.zone.run(() => {
// Perform DOM updates or state changes inside the Angular zone
});
}, 1000);
});
The runOutsideAngular()
runs tasks outside Angular’s change detection. The run()
allows controlled execution of tasks inside the Angular zone, ensuring proper change detection.
2. Use RxJS Observables
RxJS provides observables that integrate seamlessly with Angular and can handle asynchronous tasks efficiently.
Example:
import { Observable, interval } from 'rxjs';
// Use RxJS interval for periodic tasks
const subscription = interval(1000).subscribe(() => {
// Your task here
});
// To unsubscribe
subscription.unsubscribe();
RxJS observables offer more control and are well-integrated with Angular’s change detection. Use unsubscribe()
to clean up and prevent memory leaks.
3. Optimize and Limit Timer Usage
Limit the usage of setTimeout()
and setInterval()
when possible. setTimeout()
relies on browsers’ event loop, which can lead to imprecise timing.
For critical timing operations, consider using the Web API requestAnimationFrame()
or utilizing Angular’s lifecycle hooks for managing asynchronous tasks within components.
4. Proper Memory Management
Always clear timers when they’re no longer needed to prevent memory leaks. Use lifecycle hooks like ngOnDestroy()
to clean up subscriptions and timers.
Conclusion
The setTimeout()
function in Angular schedules tasks after a specified time, enhancing application responsiveness. Techniques like IIFE and recursion optimize task timing.
It’s powerful for orchestrating sequences, especially in data fetching and animations. Best practices include balancing responsiveness, adapting to conditions, and exploring advanced techniques.
Testing and user-centered design are crucial. Avoid common pitfalls like overuse and nesting. Consider alternatives for precise timing. Mastering setTimeout()
empowers developers to create dynamic, user-friendly applications.
Read this post about setInterval()
method in AngularJS.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn