Angular 中的 setTimeout() 函数
Rana Hasnain Khan
2024年2月15日
Angular
Angular Function
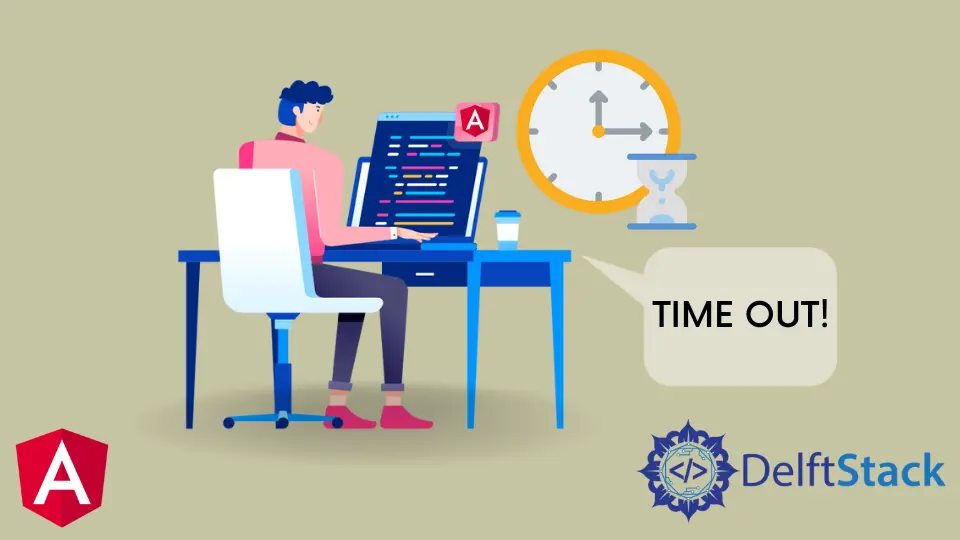
我们将使用立即调用函数表达式 (IIFE) 和函数递归来介绍 Angular 的 setTimeout()
函数。我们还将通过传递我们想要等待的时间列表并递归迭代它们来介绍 Angular 的 setTimeout()
函数。
Angular 中的 setTimeout()
函数使用 IIFE 和函数递归
我们可以出于各种原因使用 setTimeout()
函数。最好允许 Angular 在我们将同步执行的操作之间运行一次更改检测。
setTimeout()
是一个原生 JavaScript 函数,它设置一个计时器来执行回调函数,一旦计时器完成就调用该函数。
一个简单的 setTimeout()
函数示例:
let i = 0;
let max = 5;
(function repeat(){
if (++i > 5) return;
setTimeout(function(){
console.log("waited for: " + i + " seconds");
repeat();
}, 1000);
})();
输出:
在上面的代码块中,我们定义了一个整数 i
和 max
并使用了一个重复函数来重复我们的超时,直到满足条件。
如果 i
值大于 max
,此循环将中断。如果 i
的值仍然低于 max
值,它将每 1 秒重复一次并打印 waited for: 1 to 5 seconds
。
在 Angular 中 setTimeout()
函数通过传递值列表
如果我们有一个值列表,我们希望我们的程序等待并执行回调函数,我们可以将我们的函数设置为传递一个数组作为参数并运行直到数组完成。
示例代码:
let waitList = [5000, 4000, 3000, 1000];
function setDelay(times) {
if (times.length > 0) {
// Remove the first time from the array
let wait = times.shift();
console.log("Waiting For: " + wait/1000 + " seconds");
// Wait for the given amount of time
setTimeout(() => {
console.log("Waited For: " + wait/1000 + " seconds");
// Call the setDelay function again with the remaining times
setDelay(times);
}, wait);
}
}
setDelay(waitList);
输出:
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn