Angular の setTimeout()関数
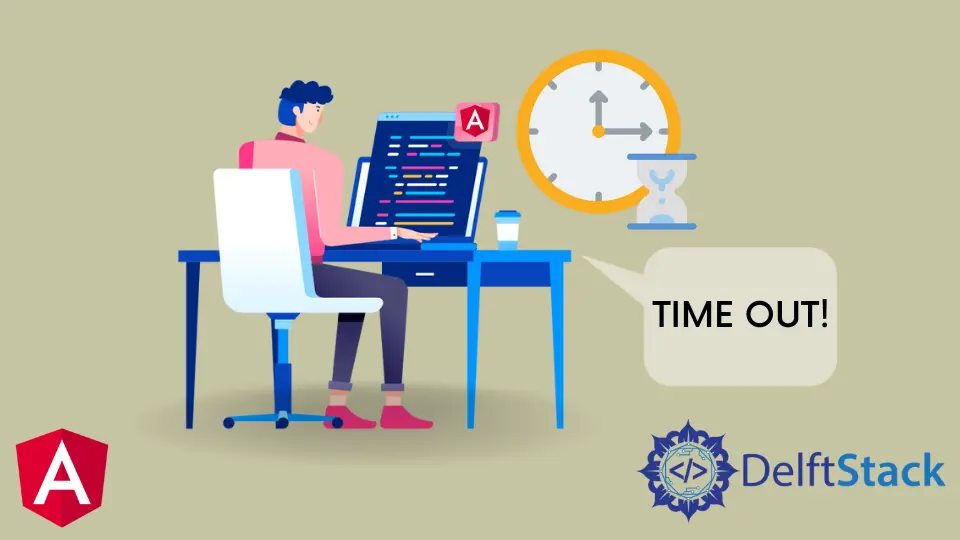
即時呼び出し関数式(IIFE)と関数再帰を使用した Angular の setTimeout()
関数を紹介します。また、待機したい時間のリストを渡し、それらを再帰的に繰り返すことで、Angular の setTimeout()
関数を紹介します。
setTimeout()
IIFE と関数再帰を使用した Angular の関数
さまざまな理由で setTimeout()
関数を使用できます。Angular が、他の方法では同期的に実行するアクションの間に、変更検出を 1 回実行できるようにするのが最善です。
setTimeout()
は、コールバック関数を実行するようにタイマーを設定するネイティブ JavaScript 関数であり、タイマーが完了すると関数を呼び出します。
簡単な setTimeout()
関数の例:
let i = 0;
let max = 5;
(function repeat(){
if (++i > 5) return;
setTimeout(function(){
console.log("waited for: " + i + " seconds");
repeat();
}, 1000);
})();
出力:
上記のコードブロックでは、整数 i
と max
を定義し、repeat 関数を使用して、条件が満たされるまでタイムアウトを繰り返しました。
i
の値が max
より大きくなると、このループは中断されます。i
の値が max
値を下回っている場合は、1 秒ごとに繰り返され、waited for: 1 to 5 seconds
が出力されます。
Angular で値のリストを渡すことによる setTimeout()
関数
プログラムが待機してコールバック関数を実行する値のリストがある場合は、配列をパラメーターとして渡し、配列が完了するまで実行するように関数を設定できます。
サンプルコード:
let waitList = [5000, 4000, 3000, 1000];
function setDelay(times) {
if (times.length > 0) {
// Remove the first time from the array
let wait = times.shift();
console.log("Waiting For: " + wait/1000 + " seconds");
// Wait for the given amount of time
setTimeout(() => {
console.log("Waited For: " + wait/1000 + " seconds");
// Call the setDelay function again with the remaining times
setDelay(times);
}, wait);
}
}
setDelay(waitList);
出力:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn