How to Use the $Eval Function in Angular
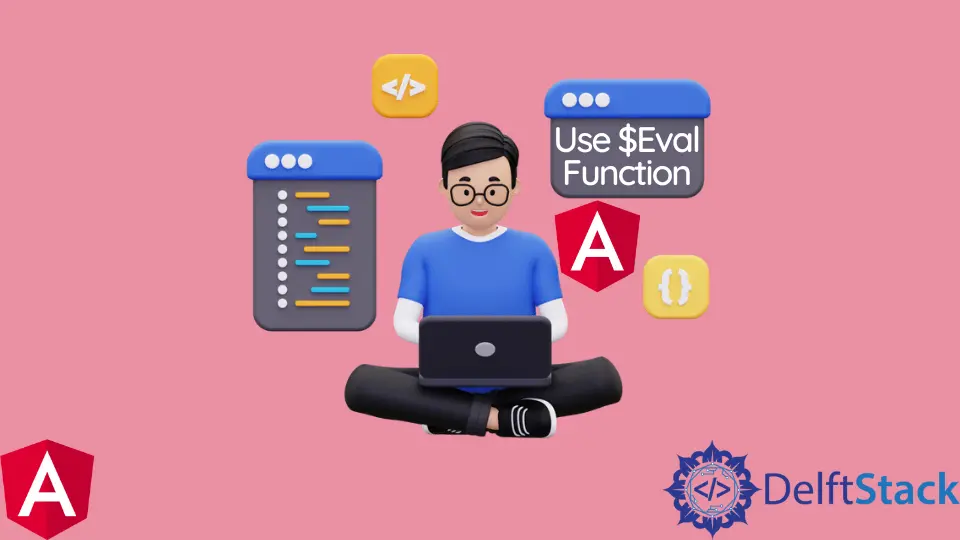
In Angular, we can use the ng-model
to bind the user input to a particular element. But if we want to access the value of an expression, we can use $eval
.
The $eval
service is a wrapper around the Angular compiler. It provides a clean interface for evaluating expressions that are passed to it.
It takes an expression and evaluates it in the context of the current scope, which means that any variable binding from the current scope will be available within the expression.
This is different from vanilla eval
. The $eval
service evaluates an expression in the context of the current scope, not globally as with vanilla eval
.
To use $eval
, you need to be aware that it will be evaluated relative to a specific scope and not outside of any scope.
Steps to Use the $eval
Function in Angular
We can use the $eval
function to execute an expression during the application’s lifetime. This can be useful for running code conditionally or implementing it only once.
-
Define an Angular service that will hold our context and
$eval
function. -
Create a directive to evaluate expressions or callbacks within its link function.
-
Inject it into the directive’s controller and bind it to a public variable called
$eval
. -
Use
$eval
in your template wherever you want to execute code based on its state or conditionals.
Let’s discuss an example of $eval
.
JavaScript code:
var app = angular.module('Adil', []);
app.controller("Demo", function($scope) {
$scope.int1 = 10;
$scope.int2 = 20;
$scope.result = $scope.$eval('int1 +int2');
});
HTML code:
<html>
<head>
<script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.0-beta.1/angular.min.js"></script>
<script src="script.js"></script>
</head>
<body >
<h2>Example of Angular $eval</h2>
<div ng-app="Adil">
<div ng-controller="Demo">
<input type="text" ng-model="int1" > + <input type="text" ng-model="int2" >
<p
>{{'Result: ' + result}}</p>
</div>
</div>
</body>
</html>
Click here to check the working of the code as mentioned above.
Pros and Cons of Using the $eval
Function in Angular
We can use the $eval
function in Angular to evaluate an expression at runtime. It is beneficial when you want to dynamically create a DOM element and add it to the DOM or when you need to do something with an object that doesn’t have any other way of being accessed.
However, the $eval
function has some disadvantages. The first disadvantage is that it can introduce security vulnerabilities because it makes your application more susceptible to injection attacks if not used correctly.
The second disadvantage is that using $eval
means your code becomes less readable because it relies on string concatenation instead of functions or variables for more common expressions.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook