The trackBy Function With ngFor in Angular
-
Using the
trackBy
Function WithngFor
in Angular -
Using the
ngFor
Function in Angular -
Using the
trackBy
WithngFor
in Angular
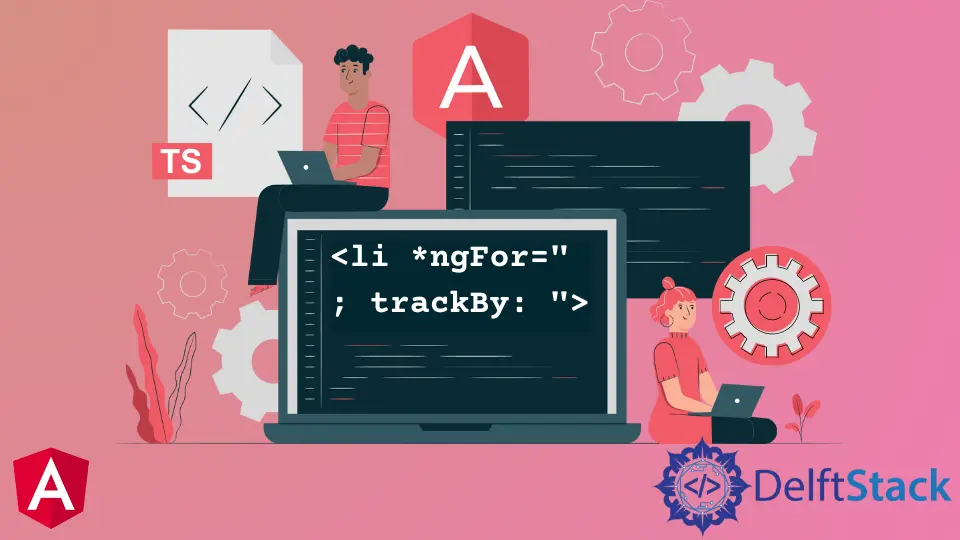
This article will tackle trackyBy
with the ngFor
directive and its utilization in Angular.
Using the trackBy
Function With ngFor
in Angular
In Angular, the trackBy
feature allows the user to select a specific key that will be used to analyze each item in the list based on that key. trackBy
is especially useful when you have nested arrays and objects that want to provide a unique identifier for each one.
It is important to note that trackBy
only applies to the current iteration of an array or object, not all future iterations.
Using the ngFor
Function in Angular
Since HTML
lacks a built-in template language, Angular adds a powerful template syntax, containing several directives like ngFor
, analogous to for-loops
in computer languages.
NgFor
is a built-in directive in Angular
that can be used to iterate over an array or an object. This directive creates a template for each item in the list, adds it to the DOM
, and updates the DOM
when there are changes in items.
Syntax:
<ul>
<li *ngFor="let item of items">{{ item.name }}</li>
</ul>
We use the ngFor
directive to iterate any array or object collection. However, if we need to update the information in the collection at some time, such as in response to an HTTP
request, we’ll run into issues.
As a result, Angular must delete and recreate all the DOM elements linked with the data. We help the Angular trackBy
function to solve this issue.
The trackBy
function accepts two arguments, the index
and the current item
. It must return the item’s specific identity.
Angular
can now track which components were added or removed depending on the particular identity. Only the constructor deletes the items that have already been modified when you update the array.
Using the trackBy
With ngFor
in Angular
Let’s discuss utilizing the trackBy
function with Angular’s ngFor
directive.
- First, we must understand the fundamentals of
Angular
and how it works. - We must install the newest version of the
Angular
Command Line Interface. - The system must have installed the most recent
Node JS
version.
We’ll now create a program that uses the template’s array to display information about the employees. We used the ngFor
directive to loop through the array of employees and display the basic information for each.
We also create a trackBy
method, which must uniquely identify each employee and allocate it to the ngFor
directive.
Code Snippet - app.component.ts
:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
informations: { id: number; name: string; Age: string; }[];
EmplyeesInfo(){
this.informations = [
{ id:1, name:'Adil', Age:' (Age 24)' },
{ id:2, name:'Steve' , Age:' (Age 30)'},
{ id:3, name:'John' ,Age:' (Age 27)'},
{ id:2, name:'Sofia' , Age:' (Age 23)' },
{ id:3, name:'Adam', Age: ' (Age 36)' }
];
}
trackEmployee(index: any,information: { id: any; }){
return information ? information.id : undefined;
}
}
Code Snippet - app.component.html
:
<button (click)="EmplyeesInfo()">Employees Information</button>
<ul>
<li *ngFor="let information of informations; trackBy: trackEmployee">
{{ information.name }}
{{ information.Age }}
</li>
</ul>
Output:
Click here to check the live demonstration of the code sample.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook