How to Use the $setValidity Function in Angular
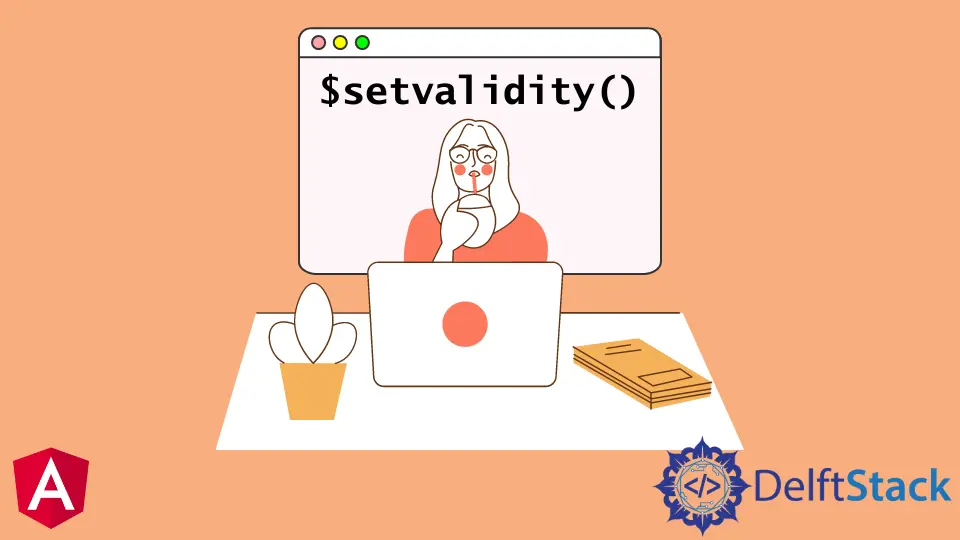
The angular $setvalidity()
is a function that sets the validity of the given form field. This function can be used both in the constructor and other controller methods.
This article will show how to use $setValidity
inside a Controller. It will also show how to set the validity of input on the server side before submitting it to the client-side.
the $setValidity
in Angular
Angular provides a directive called $setValidity
, which can set the validity of the input. It is used inside the controller and not inside a view.
The $setValidity
function assigns a key/value pair that may be used to validate the validity of a form element.
The key is unique, and the value is true or false in this context. The specific value is added to a $error
object when the $setValidity
method is called, which may be used to verify if a value is acceptable or not.
The $setValidity
function can be used in the following ways:
-
Setting the validity of an entire form:
$scope.$setValidity($scope.myForm, 'myFormIsValid');
-
Setting the validity of a field:
$scope.$setValidity($scope.myForm, 'fieldName', true);
It is important to note that the $setValidity
directive does not affect the validity of a form field. It just sets the validity of a form field and its corresponding validator.
Step to Use $setValidity
Inside a Controller in Angular
This tutorial will demonstrate how to use $setValidity
inside a Controller in Angular.
-
Create a Controller with the name
HomeController
. -
Import the
$setValidity
andFormsModule
modules. -
Create a function called
$setValidity()
. Inside this function, we will assign the value of$setValidity
withtrue
orfalse
. -
Call this function inside the
ngOnInit()
event. This will ensure that when the Angular app is initialized, it will call our$setValidity()
function.
Let’s discuss an example of how to use $setValidity
in Angular by using the steps mentioned above.
JavaScript code:
angular.module('Adil', [
'ngRoute',
'ui.router'
])
.controller('HomeController', ['$scope', function ($scope) {
$scope.loading = false;
$scope.init = function () {
$scope.data = {
};
};
$scope.save = function (data, form) {
console.log(form);
form.input.$setValidity("name", true);
}
$scope.init();
}]);
HTML code:
<html>
<head>
<h1>Example of $setValidity in Angular</h1>
<script src="script.js"></script>
</head>
<body ng-app="Adil">
<form name="Name" ng-controller="HomeController">
<div class="form-group">
<label>Please Enter a Valid URL of your Website</label>
<input type="url" />
<span ng-show="Name.input.$valid"></span>
</div>
<button ng-click="save(data, Name)">Save</button>
</form>
</body>
</html>
Click here to check the working of the code above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook