$interval in Angular 5
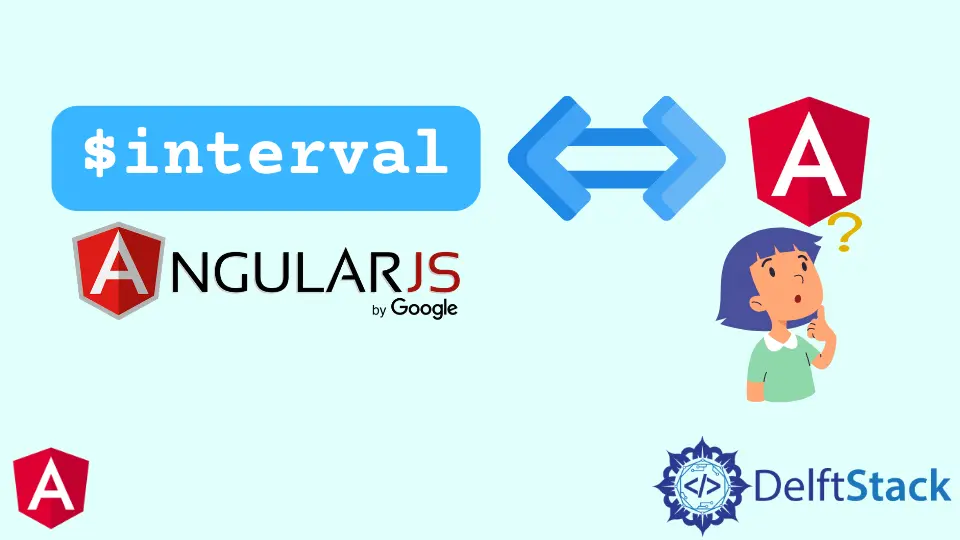
AngularJS $interval
was a way to execute a function at regular Time intervals. In Angular 5, there is a timer static method in the RxJS
library that can be used to do the same thing.
The static timer Method takes two arguments. The first is the interval in milliseconds, and the second is an optional function called when this interval elapses.
The take operator can be used to cancel any pending timers when an observable sequence completes. Let’s first discuss the $interval
method in detail and then we will move to the timer static method
and take operator
in Angular.
$interval
Service in AngularJS
The $interval
service in AngularJS does the same thing as the setInterval()
Method in JavaScript. The $interval variable is a wrapper for the setInterval()
method, making it simple to alter, delete, or mimic for testing purposes.
The setInterval
function executes the given function repeatedly at an interval in milliseconds as long as it has been called at least once.
The first call to setInterval
will execute the given function immediately, and then every specified number of milliseconds until clearInterval
is executed on the interval object itself.
Example:
let timer = setInterval(() => alert('start'), 1000);
// after 20 seconds
setTimeout(() => { clearInterval(timer); alert('stop'); }, 10000);
Use Static Time and Take
Method in Angular 5
Static Time is a new feature in Angular 5 which allows developers to set the time zone for their application’s data. This can be done at runtime by passing a value for the time zone to the Static Time
service or selecting it as an application configuration parameter.
This feature has been added to allow developers to use Angular 5 with data from different time zones without manually converting data strings. The Take
Method is another new feature that allows developers to specify how long a particular operation should take before being aborted if it takes longer.
Example Code:
import { Component, VERSION } from '@angular/core';
import { Observable, timer } from 'rxjs';
import { map, take } from 'rxjs/operators';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
counter$: Observable<number>;
count = 5;
ngOnInit() {
this.counter$ = timer(0, 5000)
.pipe(
take(5),
map(() => (this.count))
);
this.counter$.subscribe((c) => {
console.log(c);
}, () => {}, () => {
console.log('Done', this.count);
});
}
}
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook