Python os.path.samestat() Method
-
Understanding
os.path.samestat()
-
Syntax of Python
os.path.samestat()
Method -
Example 1: Basic Usage of Python
os.path.samestat()
Method -
Example 2: Use the
os.path.samestat()
Method to Check if thestat
Tuples Refer to the Same File in Python -
Example 3: Use the
os.getcwd()
Method With theos.path.samestat()
Method in Python -
Example 4: Make Your Own
os.path.samestat()
Method in Python - Conclusion
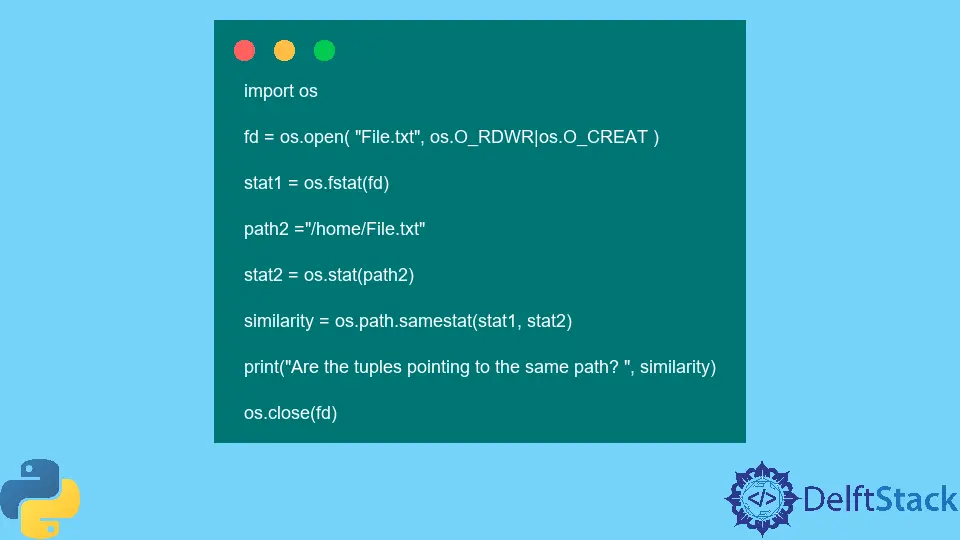
The Python programming language offers a robust set of modules for interacting with the operating system, and one such module is os.path
. Within this module, the os.path.samestat()
method stands out as a powerful tool for comparing the stat signature of two files or directories.
Python os.path.samestat()
method is an efficient way to check whether the stat
tuples refer to the same path file or not. A stat
tuple is an object that any of the Python methods can return: os.fstat()
, os.lstat()
or os.stat()
.
This article aims to provide a detailed, in-depth exploration of the os.path.samestat()
method, elucidating its syntax, parameters, return value, and practical applications.
Understanding os.path.samestat()
Overview of os.path
Before delving into the intricacies of os.path.samestat()
, it’s essential to grasp the role of os.path
in Python. This module provides a way to interact with the filesystem, offering functions for path manipulation and file-related operations.
Introduction to os.path.samestat()
The os.path.samestat()
method, introduced in Python 3.8, facilitates the comparison of the stat signatures of two files or directories. The stat signature is a tuple containing information about the file, including its size, modification time, and other attributes.
By comparing these signatures, developers can determine whether two paths point to the same file or directory.
Syntax of Python os.path.samestat()
Method
os.path.samestat(stat1, stat2)
Parameters
stat1 |
A tuple representing the status of a path. |
stat2 |
A second tuple representing the status of a path. |
Return Value
The return type of this method is a Boolean value representing true
if both the tuples refer to the same directories; otherwise false
if not.
Example 1: Basic Usage of Python os.path.samestat()
Method
Let’s explore a basic example to understand how the os.path.samestat()
method works:
import os
# Get the stat signatures of two files
stat1 = os.stat("file1.txt")
stat2 = os.stat("file2.txt")
# Check if the files have the same stat signature
result = os.path.samestat(stat1, stat2)
print("Do the files have the same stat signature?", result)
Output:
Do the files have the same stat signature? False
In this example, the stat signatures of two files, "file1.txt"
and "file2.txt"
are obtained using os.stat()
. The os.path.samestat()
method is then employed to determine if the files have the same stat signature.
Example 2: Use the os.path.samestat()
Method to Check if the stat
Tuples Refer to the Same File in Python
This code demonstrates the use of file descriptors and the os.path.samestat()
function to compare the stat information of two files. It opens or creates a file, obtains its stat information using a file descriptor, specifies another file for comparison, retrieves its stat information, and then compares the two using os.path.samestat()
.
The script concludes by printing whether the stat information tuples point to the same path and closes the file descriptor.
import os
fd = os.open("File.txt", os.O_RDWR | os.O_CREAT)
stat1 = os.fstat(fd)
path2 = "/home/File.txt"
stat2 = os.stat(path2)
similarity = os.path.samestat(stat1, stat2)
print("Are the tuples pointing to the same path? ", similarity)
os.close(fd)
Output:
Are the tuples pointing to the same path? True
In the background, this method uses the samefile()
and sameopenfile()
methods to compare the stat
tuples.
Example 3: Use the os.getcwd()
Method With the os.path.samestat()
Method in Python
This script retrieves the stat information (stat1
) for the opened or created file using os.fstat()
. It specifies another file for comparison by creating its path using os.path.join()
and the current working directory.
The stat information for the second file (stat2
) is obtained using os.stat()
. Finally, the code compares the stat information tuples of the two files using os.path.samestat()
and prints whether they point to the same path.
The file descriptor is closed using os.close()
. Overall, this script demonstrates file manipulation and stat comparison operations in Python.
import os
fd = os.open("File.txt", os.O_RDWR | os.O_CREAT)
stat1 = os.fstat(fd)
path2 = os.path.join(os.getcwd(), "File.txt")
stat2 = os.stat(path2)
similarity = os.path.samestat(stat1, stat2)
print("Are the tuples pointing to the same path? ", similarity)
os.close(fd)
Output:
Are the tuples pointing to the same path? True
This method returns true
if the tuples entered are for an original file and its shortcut is created in a different directory.
Example 4: Make Your Own os.path.samestat()
Method in Python
This code defines a custom function, os_path_samestat
, that compares the stat information of two files specified by their paths (tuple1
and tuple2
). It uses the os.path.isfile
function to check if the paths point to files, retrieves the stat information using os.stat
, and compares the device and inode numbers.
The main part of the script then opens or creates a file named "File.txt"
with read and write permissions, specifies two different paths to this file, and checks whether the stat information tuples for these paths are similar using the custom function.
The result is printed to indicate whether the stat information tuples point to the same path. The code demonstrates file-related operations and a custom comparison function for file stat information.
import os
def os_path_samestat(tuple1, tuple2):
stat1 = os.stat(tuple1) if os.path.isfile(tuple1) else None
if not stat1:
return False
stat2 = os.stat(tuple2) if os.path.isfile(tuple2) else None
if not stat2:
return False
return (stat1.st_dev == stat2.st_dev) and (stat1.st_ino == stat2.st_ino)
fd = os.open("File.txt", os.O_RDWR | os.O_CREAT)
tuple1 = "/home/File.txt"
tuple2 = os.path.join(os.getcwd(), "File.txt")
similarity = os_path_samestat(tuple1, tuple2)
print("Are the tuples pointing to the same path? ", similarity)
Output:
Are the tuples pointing to the same path? True
In some versions of Python, the original os.path.samestat()
method might not be available so that you can make your method.
Conclusion
The os.path.samestat()
method in Python provides a valuable tool for comparing the stat signatures of files or directories. Its ability to discern whether two paths point to the same filesystem entity is crucial for tasks involving file monitoring, synchronization, or integrity checks.
By understanding the syntax, parameters, and practical applications of this method, developers can leverage it effectively to enhance the robustness and reliability of their file-related operations.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn